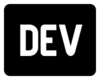

DEV Community

Posted on Jan 29, 2020
JavaScript DOM Practice Exercises For Beginners
This week on the YouTube channel I run I posted a few videos on completing practical JavaScript exercises all focused on manipulating the DOM.
I thought it would be a good way of applying your JavaScript skills, to more ‘real-life’ situations.
Here’s a sample of some of the exercises...
You can check out the setup for the exercises on their respective Codepen pages and I give an example solution (not saying it’s the best way!) for each exercise in the tutorial videos.
Video 1 : Exercise 1
See the code and full exercise on Codepen
Highlight all of the words over 8 characters long in the paragraph text (with a yellow background for example)
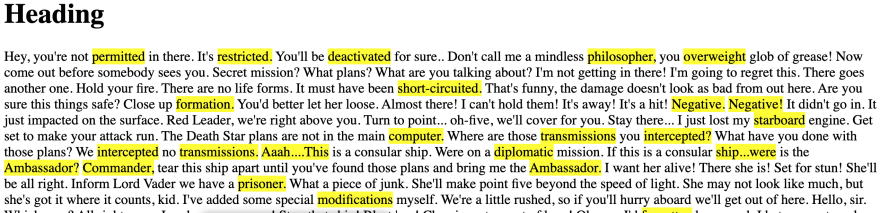
In this exercise, I was asking users to extract the contents of a paragraph tag and then put a highlighted background behind words that are longer than 8 characters. It’s always tricky to work determine where a word starts and ends in a string (multiple spaces, symbols etc.) but because we only needed to apply the rule to words over 8 characters we can get away with a relaxed approach.
How would you go about solving this one?
Video 1 : Exercise 5
Replace all question marks (?) with thinking faces (🤔) and exclamation marks (!) with astonished faces (😲)
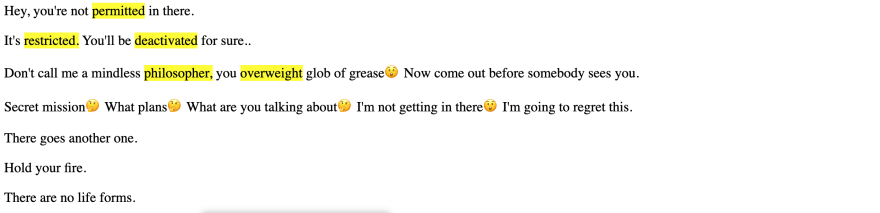
This one was, hopefully, quite straightforward although it did get a bit more complicated as a previous exercise had created multiple paragraph tags on the page. It’s a good bit of string manipulation practice too.
Got your own solution for this?
Video 2 : Exercise 2
Add a required validation to each input that shows an error message next to the entry if it does not have any text entered.
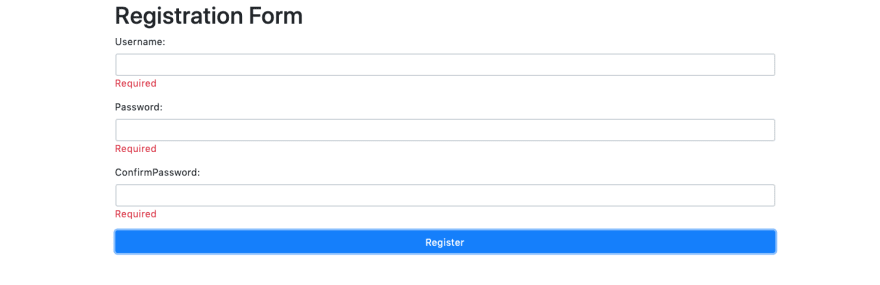
So in Video 2 we were working with a simple Bootstrap based registration form and this exercise was based around setting up some form validation. I was looking for a simple solution to this one but it was complicated managing multiple instances of errors (like them stacking on top of each other when the validation hasn’t been met). So the solution I provided was a bit messy, but did the trick.
Can you solve this with a simpler solution?
Video 3 : Exercise 2
To make the ordering of the plans more logical, using JavaScript, move the basic plan to be before (to the left) of the pro plan.

In Video 3 we had a simple pricing table with two products, a basic and pro plan and this was an exercise in moving elements around in the DOM. There’s a simple solution to this using CSS but can you achieve this using JavaScript?
Video 3 : Exercise 3
To make the Pro plan have a stronger call to action, update the current 'Get started' button to be blue (#007bff) with white text and have the text 'Buy Now'
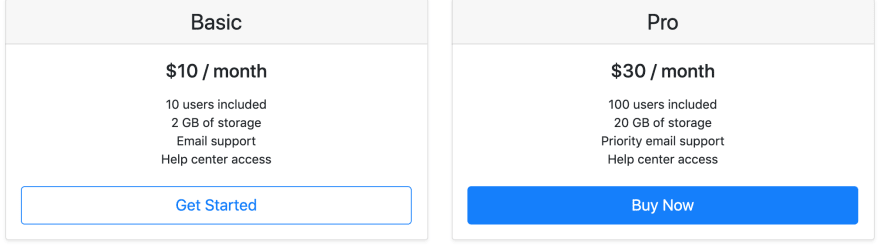
Normally you would update your styles directly in your CSS (or it’s pre-processor) but this exercise was asking you to do this with JavaScript and there’s a shortcut you can take if you know your Bootstrap classes.
If you check out the exercises then I hope you find them useful for practicing your JavaScript skills. If you do then consider subscribing to the Junior Developer Central channel and don’t forget to leave a comment with your own solutions to the exercises.
Top comments (0)
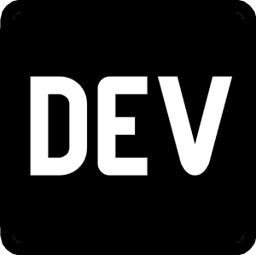
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
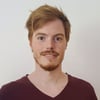
Path To A Clean(er) React Architecture - Domain Entities & DTOs
Johannes Kettmann - May 24

Modals with Remix
ddm4313 - May 24

Exploring React Router 6
just-vicky - May 24

Bookmyflight : A web app that helps you find cheap flights and book them based on your budget.
Nitin Singh - May 23
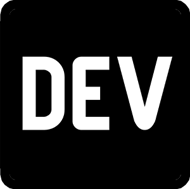
We're a place where coders share, stay up-to-date and grow their careers.
The Modern JavaScript DOM Cheat Sheet
This article provides a comprehensive cheat sheet for modern JavaScript DOM, covering a wide range of methods and properties that you can use to interact with the Document Object Model in your web applications.
Selecting Elements
- document.querySelector(selector) – selects the first element that matches the CSS selector.
- document.querySelectorAll(selector) – selects all elements that match the CSS selector.
- document.getElementById(id) – selects the element with the specified ID.
- document.getElementsByClassName(className) – selects all elements with the specified class name.
- document.getElementsByTagName(tagName) – selects all elements with the specified tag name.
- element.matches(selector) – returns a Boolean indicating whether the element matches the specified CSS selector.
Creating and Modifying Elements
- document.createElement(tagName) – creates a new element with the specified tag name.
- element.appendChild(childElement) – appends a child element to the end of the parent element.
- element.insertBefore(newElement, referenceElement) – inserts a new element before the specified reference element.
- element.setAttribute(name, value) – sets the value of the specified attribute for an element.
- element.removeAttribute(name) – removes the specified attribute from an element.
- element.innerHTML – sets or gets the HTML content of an element.
- element.textContent – sets or gets the text content of an element.
- element.insertAdjacentHTML(position, htmlString) – inserts HTML into the specified position relative to the element.
- element.insertAdjacentText(position, text) – inserts text into the specified position relative to the element.
Styling Elements
- element.style.property = value – sets a CSS property for an element.
- element.classList.add(className) – adds a class to an element.
- element.classList.remove(className) – removes a class from an element.
- element.classList.toggle(className) – toggles a class on or off for an element.
- element.classList.contains(className) – returns a Boolean value indicating whether an element has a specified class.
- window.getComputedStyle(element) – returns the computed style of an element.
Event Handling
- element.addEventListener(event, function) – adds an event listener to an element.
- element.removeEventListener(event, function) – removes an event listener from an element.
- event.preventDefault() – prevents the default action of an event.
- event.stopPropagation() – stops the propagation of an event to parent elements.
- event.target – returns the element that triggered the event.
- event.currentTarget – returns the element to which the event listener is attached.
- event.type – returns the type of the event.
- event.key – returns the key that was pressed (for keyboard events).
- event.keyCode – returns the Unicode value of the key that was pressed (for keyboard events).
- element.parentNode – returns the parent node of an element.
- element.childNodes – returns a collection of all child nodes of an element.
- element.firstChild – returns the first child node of an element.
- element.lastChild – returns the last child node of an element.
- element.previousSibling – returns the previous sibling node of an element.
- element.nextSibling – returns the next sibling node of an element.
- element.parentElement – returns the parent element of an element (excluding text and comment nodes).
- element.children – returns a collection of all child elements of an element (excluding text and comment nodes).
Attributes and Properties
- element.getAttribute(name) – returns the value of the specified attribute for an element.
- element.propertyName – sets or gets the value of a property for an element.
- element.dataset – returns a DOMStringMap containing all the custom data attributes of an element.
- element.hasAttribute(name) – returns a Boolean indicating whether an element has the specified attribute.
- element.propertyName = value – sets the value of a property for an element.
DOM Manipulation
- document.createDocumentFragment() – creates a new empty document fragment.
- element.cloneNode(deep) – creates a clone of an element, optionally cloning its child nodes.
- element.removeChild(childElement) – removes a child element from the parent element.
- element.replaceChild(newElement, oldElement) – replaces an old child element with a new child element.
- element.contains(childElement) – returns a Boolean indicating whether an element is a descendant of another element.
- document.importNode(node, deep) – imports a node from another document into the current document.
Performance Optimization
- requestAnimationFrame(callback) – schedules a function to run the next time the browser renders a frame, usually at 60 frames per second.
- window.performance.mark(name) – creates a performance mark with the specified name.
- window.performance.measure(name, startMark, endMark) – creates a performance measure with the specified name, using the specified start and end marks.
- element.getBoundingClientRect() – returns a DOMRect object containing the size and position of an element.
- element.offsetWidth – returns the width of an element, including padding and border but not margin.
- element.offsetHeight – returns the height of an element, including padding and border but not margin.
- element.offsetLeft – returns the distance between an element’s left edge and its offset parent’s left edge.
- element.offsetTop – returns the distance between an element’s top edge and its offset parent’s top edge.
More Methods and Properties
- document.cookie – sets or gets the cookies associated with the current document.
- document.title – sets or gets the title of the current document.
- window.location – sets or gets the current URL of the window.
- window.navigator – returns an object containing information about the user’s browser and operating system.
- window.alert(message) – displays an alert dialog with the specified message.
- window.prompt(message, defaultValue) – displays a prompt dialog with the specified message and default value.
The above is the most concise information about the DOM in modern JavaScript. If you want to see more detailed guides and specific examples, keep reading the tutorials in this series, such as:
- JavaScript: Set HTML lang attribute programmatically
- JavaScript: Detect a click outside an HTML element
Happy coding and have a nice day!
Next Article: JavaScript: Set HTML lang attribute programmatically
Series: JavaScript: Document Object Model Tutorials
Related Articles
- JavaScript: Press ESC to exit fullscreen mode (2 examples)
- Can JavaScript programmatically bookmark a page? (If not, what are alternatives)
- Dynamic Import in JavaScript: Tutorial & Examples (ES2020+)
- JavaScript: How to implement auto-save form
- JavaScript: Disable a Button After a Click for N Seconds
- JavaScript: Detect when a Div goes into viewport
- JavaScript Promise.any() method (basic and advanced examples)
- Using logical nullish assignment in JavaScript (with examples)
- Understanding WeakRef in JavaScript (with examples)
- JavaScript Numeric Separators: Basic and Advanced Examples
- JavaScript: How to Identify Mode(s) of an Array (3 Approaches)
- JavaScript: Using AggregateError to Handle Exceptions
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js
DOM Manipulation and Events
Foundations course, introduction.
One of the most unique and useful abilities of JavaScript is its ability to manipulate the DOM. But what is the DOM, and how do we go about changing it? Let’s jump right in…
Lesson overview
This section contains a general overview of topics that you will learn in this lesson.
- Explain what the DOM is in relation to a webpage.
- Explain the difference between a “node” and an “element”.
- Explain how to target nodes with “selectors”.
- Explain the basic methods for finding, adding, removing, and altering DOM nodes.
- Explain the difference between a “NodeList” and an “array of nodes”.
- Explain what “bubbling” is and how it works.
Document Object Model
The DOM (or Document Object Model) is a tree-like representation of the contents of a webpage - a tree of “nodes” with different relationships depending on how they’re arranged in the HTML document.
In the above example, the <div class="display"></div> is a “child” of <div id="container"></div> and a “sibling” to <div class="controls"></div> . Think of it like a family tree. <div id="container"></div> is a parent , with its children on the next level, each on their own “branch”.
Targeting nodes with selectors
When working with the DOM, you use “selectors” to target the nodes you want to work with. You can use a combination of CSS-style selectors and relationship properties to target the nodes you want. Let’s start with CSS-style selectors. In the above example, you could use the following selectors to refer to <div class="display"></div> :
- div.display
- #container > .display
- div#container > div.display
You can also use relational selectors (i.e., firstElementChild or lastElementChild , etc.) with special properties owned by the nodes.
So you’re identifying a certain node based on its relationships to the nodes around it.
DOM methods
When your HTML code is parsed by a web browser, it is converted to the DOM, as was mentioned above. One of the primary differences is that these nodes are JavaScript objects that have many properties and methods attached to them. These properties and methods are the primary tools we are going to use to manipulate our webpage with JavaScript. We’ll start with the query selectors - those that help you target nodes.
Query selectors
- element.querySelector(selector) - returns a reference to the first match of selector .
- element.querySelectorAll(selectors) - returns a “NodeList” containing references to all of the matches of the selectors .
It’s important to remember that when using querySelectorAll, the return value is not an array. It looks like an array, and it somewhat acts like an array, but it’s really a “NodeList”. The big distinction is that several array methods are missing from NodeLists. One solution, if problems arise, is to convert the NodeList into an array. You can do this with Array.from() or the spread operator.
Element creation
- document.createElement(tagName, [options]) - creates a new element of tag type tagName. [options] in this case means you can add some optional parameters to the function. Don’t worry about these at this point.
This function does NOT put your new element into the DOM - it creates it in memory. This is so that you can manipulate the element (by adding styles, classes, ids, text, etc.) before placing it on the page. You can place the element into the DOM with one of the following methods.
Append elements
- parentNode.appendChild(childNode) - appends childNode as the last child of parentNode .
- parentNode.insertBefore(newNode, referenceNode) - inserts newNode into parentNode before referenceNode .
Remove elements
- parentNode.removeChild(child) - removes child from parentNode on the DOM and returns a reference to child .
Altering elements
When you have a reference to an element, you can use that reference to alter the element’s own properties. This allows you to do many useful alterations, like adding, removing, or altering attributes, changing classes, adding inline style information, and more.
Adding inline style
When accessing a kebab-cased CSS property like background-color with JS, you will need to either use camelCase with dot notation or bracket notation. When using bracket notation, you can use either camelCase or kebab-case, but the property name must be a string.
Editing attributes
See MDN’s section on HTML Attributes for more information on available attributes.
Working with classes
It is often standard (and cleaner) to toggle a CSS style rather than adding and removing inline CSS.
Adding text content
Adding html content.
Note that using textContent is preferred over innerHTML for adding text, as innerHTML should be used sparingly to avoid potential security risks. To understand the dangers of using innerHTML, watch this video about preventing the most common cross-site scripting attack .
Let’s take a minute to review what we’ve covered and give you a chance to practice this stuff before moving on. Check out this example of creating and appending a DOM element to a webpage.
In the JavaScript file, first we get a reference to the container div that already exists in our HTML. Then we create a new div and store it in the variable content . We add a class and some text to the content div and finally append that div to container . After the JavaScript code is run, our DOM tree will look like this:
Keep in mind that the JavaScript does not alter your HTML, but the DOM - your HTML file will look the same, but the JavaScript changes what the browser renders.
Your JavaScript, for the most part, is run whenever the JS file is run or when the script tag is encountered in the HTML. If you are including your JavaScript at the top of your file, many of these DOM manipulation methods will not work because the JS code is being run before the nodes are created in the DOM. The simplest way to fix this is to include your JavaScript at the bottom of your HTML file so that it gets run after the DOM nodes are parsed and created.
Alternatively, you can link the JavaScript file in the <head> of your HTML document. Use the <script> tag with the src attribute containing the path to the JS file, and include the defer keyword to load the file after the HTML is parsed, as such:
Find out more about the defer attribute for script tags .
Copy the example above into files on your own computer. To make it work, you’ll need to supply the rest of the HTML skeleton and either link your JavaScript file or put the JavaScript into a script tag on the page. Make sure everything is working before moving on!
Add the following elements to the container using ONLY JavaScript and the DOM methods shown above:
- a <p> with red text that says “Hey I’m red!”
- an <h3> with blue text that says “I’m a blue h3!”
- another <h1> that says “I’m in a div”
- a <p> that says “ME TOO!”
- Hint for this one: after creating the <div> with createElement, append the <h1> and <p> to it before adding it to the container.
Now that we have a handle on manipulating the DOM with JavaScript, the next step is learning how to make that happen dynamically or on demand! Events are how you make that magic happen on your pages. Events are actions that occur on your webpage, such as mouse-clicks or key-presses. Using JavaScript, we can make our webpage listen to and react to these events.
There are three primary ways to go about this:
- You can specify function attributes directly on your HTML elements.
- You can set properties in the form of on<eventType> , such as onclick or onmousedown , on the DOM nodes in your JavaScript.
- You can attach event listeners to the DOM nodes in your JavaScript.
Event listeners are definitely the preferred method, but you will regularly see the others in use, so we’re going to cover all three.
We’re going to create three buttons that all alert “Hello World” when clicked. Try them all out using your own HTML file or using something like CodePen .
This solution is less than ideal because we’re cluttering our HTML with JavaScript. Also, we can only set one “onclick” property per DOM element, so we’re unable to run multiple separate functions in response to a click event using this method.
If you need to review the arrow syntax () => , check this article about arrow functions .
This is a little better. We’ve moved the JS out of the HTML and into a JS file, but we still have the problem that a DOM element can only have one “onclick” property.
Now, we maintain separation of concerns, and we also allow multiple event listeners if the need arises. Method 3 is much more flexible and powerful, though it is a bit more complex to set up.
Note that all three of these methods can be used with named functions like so:
Using named functions can clean up your code considerably, and is a really good idea if the function is something that you are going to want to do in multiple places.
With all three methods, we can access more information about the event by passing a parameter to the function that we are calling. Try this out on your own machine:
When we pass in alertFunction or function (e) {...} as an argument to addEventListener , we call this a callback . A callback is simply a function that is passed into another function as an argument.
The e parameter in that callback function contains an object that references the event itself. Within that object you have access to many useful properties and methods (functions that live inside an object) such as which mouse button or key was pressed, or information about the event’s target - the DOM node that was clicked.
and now this:
Pretty cool, eh?
Attaching listeners to groups of nodes
This might seem like a lot of code if you’re attaching lots of similar event listeners to many elements. There are a few ways to go about doing that more efficiently. We learned above that we can get a NodeList of all of the items matching a specific selector with querySelectorAll('selector') . In order to add a listener to each of them, we need to iterate through the whole list, like so:
This is just the tip of the iceberg when it comes to DOM manipulation and event handling, but it’s enough to get you started with some exercises. In our examples so far, we have been using the ‘click’ event exclusively, but there are many more available to you.
Some useful events include:
You can find a more complete list with explanations of each event on W3Schools JavaScript Event Reference page .
Manipulating web pages is the primary benefit of the JavaScript language! These techniques are things that you are likely to be messing with every day as a front-end developer, so let’s practice!
- Complete MDN’s Active Learning sections on DOM manipulation and test your skills!
Read the following sections from JavaScript Tutorial’s series on the DOM to get a broader idea of how events can be used in your pages. Note that some of the methods like getElementById are older and see less use today.
As you read, remember that the general ideas can be applied to any event, not only the ones used in examples - but information specific to a certain event type can always be found by checking documentation.
- JavaScript events
- Page load events
- Mouse events
- Keyboard events
- Event delegation
- The dispatchEvent method
- Custom events
Knowledge check
The following questions are an opportunity to reflect on key topics in this lesson. If you can’t answer a question, click on it to review the material, but keep in mind you are not expected to memorize or master this knowledge.
- What is the DOM?
- How do you target the nodes you want to work with?
- How do you create an element in the DOM?
- How do you add an element to the DOM?
- How do you remove an element from the DOM?
- How can you alter an element in the DOM?
- When adding text to a DOM element, should you use textContent or innerHTML? Why?
- Where should you include your JavaScript tag in your HTML file when working with DOM nodes?
- How do “events” and “listeners” work?
- What are three ways to use events in your code?
- Why are event listeners the preferred way to handle events?
- What are the benefits of using named functions in your listeners?
- How do you attach listeners to groups of nodes?
- What is the difference between the return values of querySelector and querySelectorAll ?
- What does a “NodeList” contain?
- Explain the difference between “capture” and “bubbling”.
Additional resources
This section contains helpful links to related content. It isn’t required, so consider it supplemental.
- Eloquent JS - DOM
- Eloquent JS - Handling Events
- DOM Enlightenment
- Plain JavaScript is a reference of JavaScript code snippets and explanations involving the DOM, as well as other aspects of JS. If you’ve already learned jQuery, it will help you figure out how to do things without it.
- This W3Schools article offers easy-to-understand lessons on the DOM.
- JS DOM Crash Course is an extensive and well explained 4 part video series on the DOM by Traversy Media.
- Understanding The Dom is an aptly named article-based tutorial series by DigitalOcean.
- Introduction to events by MDN covers the same topics you learned in this lesson on events.
- Wes Bos’s Drumkit JavaScript30 program reinforces the content covered in the assignment. In the video you will notice that a deprecated keycode keyboard event is used, replace it with the recommended code keyboard event and replace the data-key tags accordingly.
- Event Capture, Propagation and Bubbling video from Wes Bos’s JavaScript30 program.
- Understanding Callbacks in JavaScript for a more in-depth understanding of callbacks.
Attributes and properties
When the browser loads the page, it “reads” (another word: “parses”) the HTML and generates DOM objects from it. For element nodes, most standard HTML attributes automatically become properties of DOM objects.
For instance, if the tag is <body id="page"> , then the DOM object has body.id="page" .
But the attribute-property mapping is not one-to-one! In this chapter we’ll pay attention to separate these two notions, to see how to work with them, when they are the same, and when they are different.
DOM properties
We’ve already seen built-in DOM properties. There are a lot. But technically no one limits us, and if there aren’t enough, we can add our own.
DOM nodes are regular JavaScript objects. We can alter them.
For instance, let’s create a new property in document.body :
We can add a method as well:
We can also modify built-in prototypes like Element.prototype and add new methods to all elements:
So, DOM properties and methods behave just like those of regular JavaScript objects:
- They can have any value.
- They are case-sensitive (write elem.nodeType , not elem.NoDeTyPe ).
HTML attributes
In HTML, tags may have attributes. When the browser parses the HTML to create DOM objects for tags, it recognizes standard attributes and creates DOM properties from them.
So when an element has id or another standard attribute, the corresponding property gets created. But that doesn’t happen if the attribute is non-standard.
For instance:
Please note that a standard attribute for one element can be unknown for another one. For instance, "type" is standard for <input> ( HTMLInputElement ), but not for <body> ( HTMLBodyElement ). Standard attributes are described in the specification for the corresponding element class.
Here we can see it:
So, if an attribute is non-standard, there won’t be a DOM-property for it. Is there a way to access such attributes?
Sure. All attributes are accessible by using the following methods:
- elem.hasAttribute(name) – checks for existence.
- elem.getAttribute(name) – gets the value.
- elem.setAttribute(name, value) – sets the value.
- elem.removeAttribute(name) – removes the attribute.
These methods operate exactly with what’s written in HTML.
Also one can read all attributes using elem.attributes : a collection of objects that belong to a built-in Attr class, with name and value properties.
Here’s a demo of reading a non-standard property:
HTML attributes have the following features:
- Their name is case-insensitive ( id is same as ID ).
- Their values are always strings.
Here’s an extended demo of working with attributes:
Please note:
- getAttribute('About') – the first letter is uppercase here, and in HTML it’s all lowercase. But that doesn’t matter: attribute names are case-insensitive.
- We can assign anything to an attribute, but it becomes a string. So here we have "123" as the value.
- All attributes including ones that we set are visible in outerHTML .
- The attributes collection is iterable and has all the attributes of the element (standard and non-standard) as objects with name and value properties.
Property-attribute synchronization
When a standard attribute changes, the corresponding property is auto-updated, and (with some exceptions) vice versa.
In the example below id is modified as an attribute, and we can see the property changed too. And then the same backwards:
But there are exclusions, for instance input.value synchronizes only from attribute → property, but not back:
In the example above:
- Changing the attribute value updates the property.
- But the property change does not affect the attribute.
That “feature” may actually come in handy, because the user actions may lead to value changes, and then after them, if we want to recover the “original” value from HTML, it’s in the attribute.
DOM properties are typed
DOM properties are not always strings. For instance, the input.checked property (for checkboxes) is a boolean:
There are other examples. The style attribute is a string, but the style property is an object:
Most properties are strings though.
Quite rarely, even if a DOM property type is a string, it may differ from the attribute. For instance, the href DOM property is always a full URL, even if the attribute contains a relative URL or just a #hash .
Here’s an example:
If we need the value of href or any other attribute exactly as written in the HTML, we can use getAttribute .
Non-standard attributes, dataset
When writing HTML, we use a lot of standard attributes. But what about non-standard, custom ones? First, let’s see whether they are useful or not? What for?
Sometimes non-standard attributes are used to pass custom data from HTML to JavaScript, or to “mark” HTML-elements for JavaScript.
Also they can be used to style an element.
For instance, here for the order state the attribute order-state is used:
Why would using an attribute be preferable to having classes like .order-state-new , .order-state-pending , .order-state-canceled ?
Because an attribute is more convenient to manage. The state can be changed as easy as:
But there may be a possible problem with custom attributes. What if we use a non-standard attribute for our purposes and later the standard introduces it and makes it do something? The HTML language is alive, it grows, and more attributes appear to suit the needs of developers. There may be unexpected effects in such case.
To avoid conflicts, there exist data-* attributes.
All attributes starting with “data-” are reserved for programmers’ use. They are available in the dataset property.
For instance, if an elem has an attribute named "data-about" , it’s available as elem.dataset.about .
Multiword attributes like data-order-state become camel-cased: dataset.orderState .
Here’s a rewritten “order state” example:
Using data-* attributes is a valid, safe way to pass custom data.
Please note that we can not only read, but also modify data-attributes. Then CSS updates the view accordingly: in the example above the last line (*) changes the color to blue.
- Attributes – is what’s written in HTML.
- Properties – is what’s in DOM objects.
A small comparison:
Methods to work with attributes are:
- elem.hasAttribute(name) – to check for existence.
- elem.getAttribute(name) – to get the value.
- elem.setAttribute(name, value) – to set the value.
- elem.removeAttribute(name) – to remove the attribute.
- elem.attributes is a collection of all attributes.
For most situations using DOM properties is preferable. We should refer to attributes only when DOM properties do not suit us, when we need exactly attributes, for instance:
- We need a non-standard attribute. But if it starts with data- , then we should use dataset .
- We want to read the value “as written” in HTML. The value of the DOM property may be different, for instance the href property is always a full URL, and we may want to get the “original” value.
Get the attribute
Write the code to select the element with data-widget-name attribute from the document and to read its value.
Make external links orange
Make all external links orange by altering their style property.
A link is external if:
- Its href has :// in it
- But doesn’t start with http://internal.com .
The result should be:
Open a sandbox for the task.
First, we need to find all external references.
There are two ways.
The first is to find all links using document.querySelectorAll('a') and then filter out what we need:
Please note: we use link.getAttribute('href') . Not link.href , because we need the value from HTML.
…Another, simpler way would be to add the checks to CSS selector:
Open the solution in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)

Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
JavaScript ( JS ) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions . While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js , Apache CouchDB and Adobe Acrobat . JavaScript is a prototype-based , multi-paradigm, single-threaded , dynamic language, supporting object-oriented, imperative, and declarative (e.g. functional programming) styles.
JavaScript's dynamic capabilities include runtime object construction, variable parameter lists, function variables, dynamic script creation (via eval ), object introspection (via for...in and Object utilities ), and source-code recovery (JavaScript functions store their source text and can be retrieved through toString() ).
This section is dedicated to the JavaScript language itself, and not the parts that are specific to Web pages or other host environments. For information about APIs that are specific to Web pages, please see Web APIs and DOM .
The standards for JavaScript are the ECMAScript Language Specification (ECMA-262) and the ECMAScript Internationalization API specification (ECMA-402). As soon as one browser implements a feature, we try to document it. This means that cases where some proposals for new ECMAScript features have already been implemented in browsers, documentation and examples in MDN articles may use some of those new features. Most of the time, this happens between the stages 3 and 4, and is usually before the spec is officially published.
Do not confuse JavaScript with the Java programming language — JavaScript is not "Interpreted Java" . Both "Java" and "JavaScript" are trademarks or registered trademarks of Oracle in the U.S. and other countries. However, the two programming languages have very different syntax, semantics, and use.
JavaScript documentation of core language features (pure ECMAScript , for the most part) includes the following:
- The JavaScript guide
- The JavaScript reference
For more information about JavaScript specifications and related technologies, see JavaScript technologies overview .
Learn how to program in JavaScript with guides and tutorials.
For complete beginners
Head over to our Learning Area JavaScript topic if you want to learn JavaScript but have no previous experience with JavaScript or programming. The complete modules available there are as follows:
Answers some fundamental questions such as "what is JavaScript?", "what does it look like?", and "what can it do?", along with discussing key JavaScript features such as variables, strings, numbers, and arrays.
Continues our coverage of JavaScript's key fundamental features, turning our attention to commonly-encountered types of code blocks such as conditional statements, loops, functions, and events.
The object-oriented nature of JavaScript is important to understand if you want to go further with your knowledge of the language and write more efficient code, therefore we've provided this module to help you.
Discusses asynchronous JavaScript, why it is important, and how it can be used to effectively handle potential blocking operations such as fetching resources from a server.
Explores what APIs are, and how to use some of the most common APIs you'll come across often in your development work.
JavaScript guide
A much more detailed guide to the JavaScript language, aimed at those with previous programming experience either in JavaScript or another language.
Intermediate
JavaScript frameworks are an essential part of modern front-end web development, providing developers with proven tools for building scalable, interactive web applications. This module gives you some fundamental background knowledge about how client-side frameworks work and how they fit into your toolset, before moving on to a series of tutorials covering some of today's most popular ones.
An overview of the basic syntax and semantics of JavaScript for those coming from other programming languages to get up to speed.
Overview of available data structures in JavaScript.
JavaScript provides three different value comparison operations: strict equality using === , loose equality using == , and the Object.is() method.
How different methods that visit a group of object properties one-by-one handle the enumerability and ownership of properties.
A closure is the combination of a function and the lexical environment within which that function was declared.
Explanation of the widely misunderstood and underestimated prototype-based inheritance.
Memory life cycle and garbage collection in JavaScript.
JavaScript has a runtime model based on an "event loop".
Browse the complete JavaScript reference documentation.
Get to know standard built-in objects Array , Boolean , Date , Error , Function , JSON , Math , Number , Object , RegExp , String , Map , Set , WeakMap , WeakSet , and others.
Learn more about the behavior of JavaScript's operators instanceof , typeof , new , this , the operator precedence , and more.
Learn how do-while , for-in , for-of , try-catch , let , var , const , if-else , switch , and more JavaScript statements and keywords work.
Learn how to work with JavaScript's functions to develop your applications.
JavaScript classes are the most appropriate way to do object-oriented programming.
Home » React Tutorial » React API Call
React API Call
Summary : in this tutorial, you will learn to call API in a React app using the built-in Fetch API provided by web browsers.
Introduction to Wikipedia Search React App
We’ll create a new React app that allows you to search Wikipedia articles . To do that, the React app needs to call Wikipedia Search API:
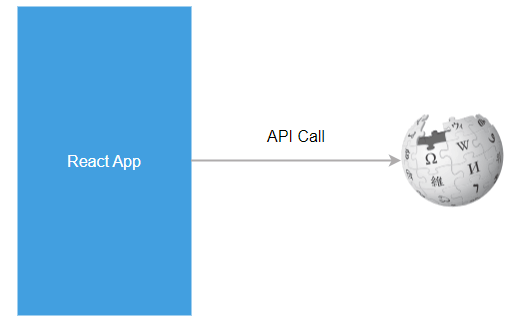
The React app will look like the following:
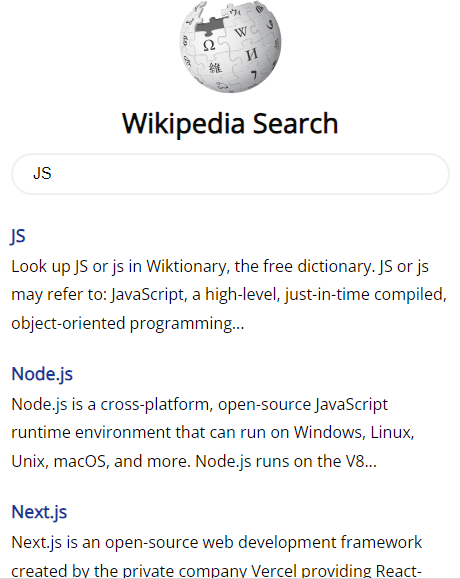
We can break down this React app into the following components:
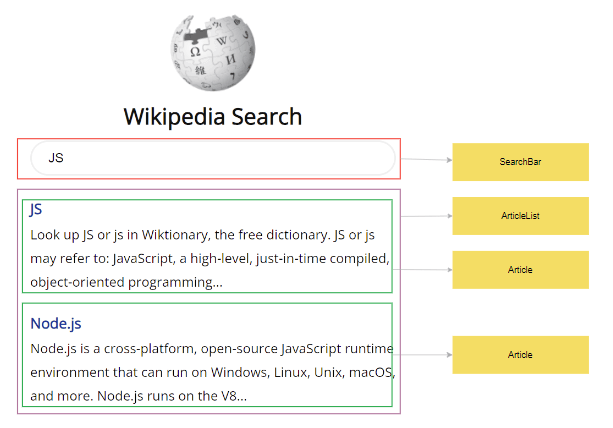
Here’s the component hierarchy:
In this component hierarchy:
- The App component is a parent component that includes other components.
- The SearchBar component allows you to type a search term and press the Enter key to submit it.
- The ArticleList component is responsible for rendering the search results, which are a list of articles.
- The Article component renders each article in the search results.
Props and State Design
There are some questions we need to answer before creating the app:
- What is the current state of the application?
- Which component should handle the API call?
- How should we design the props system?
The app displays a list of articles so it should have an array of articles as a state:
Since the App component uses the ArticleList component to render the article list, it should pass the articles array as a prop to the ArticleList .
Additionally, the ArticleList should pass each article as a prop to the Article component for rendering each article.
If we call Wikipedia in the SearchBar component, we can get a list of articles. But how do we pass the article list from the SearchBar component to the App component?
Typically, React only allows us to pass props from parent components to the child components, not the other way around.
In other words, React does not allow you to pass a prop from a child component to its parent component.
To overcome this limitation, you can pass a function from the App component to the SearchBar component as a prop.
When the user submits the form, we can call the function to update the articles state of the App component.
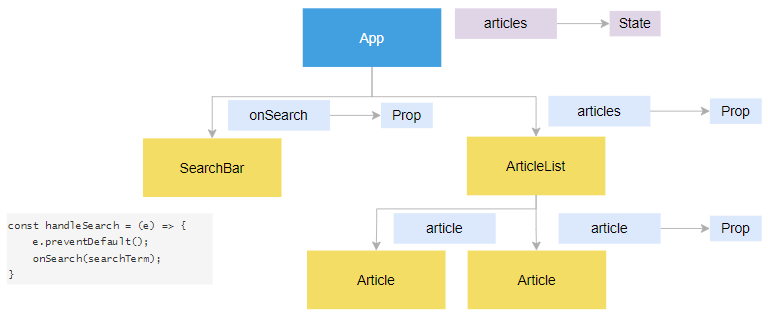
Create a new React app
First, open a terminal on your computer and create a new React app using the create-react-app command:
Next, delete all files in the src directory.
Then, create a new index.js file in the src directory and add the following code:
The index.js file renders the App component on the screen.
After that, create a new file named app.js in the src directory with the following code:
Finally, run the React app by running the following command in the terminal:
You’ll see the new React app on the web browser at https://localhost:3000.
Calling an API
There are several ways to call an API from a React app. The simplest way is to use the native Fetch API provided by web browsers because you don’t need to install a third-party package.
First, call the fetch() method by passing the API endpoint:
Second, call the json() method of the Response object to parse the JSON body contents:
Third, return the parsed JSON data:
To handle the error that may occur during the API call, you can use the try … catch statement:
Calling Wikipedia Search API
First, create a new file in the src directory named api.js :
Second, define a search() function that calls Wikipedia Search API for a specified search term and returns an array of articles:
How it works.
Step 1. Define a search function that accepts a search term:
Step 2. Construct the URL API endpoint by concatenating the API URL with the search term:
Step 3. Return JSON data from the API:
Step 4. Display an error in the catch block if it occurs:
The search results include pageid , title , and snippet . The title and snippet may contain HTML tags.
To strip the HTML tags, we can create a new file util.js in the src directory and define the stripHTML() function as follows:
We’ll use the stripHTML in the Article component to strip HTML from the title and snippet .
Create React components
We’ll create the components for the React app.
App component
The following App component includes the SearchBar and ArticleList components:
Step 1. Import the useState function from the react library because the App will hold some piece of state.
Step 2. Import the search function from the api.js module:
Step 3. Import the SearchBar and ArticleList components:
Step 4. Import the App.css and wikipedia-logo.png files:
Step 5. Define the App component:
Step 5. Define a piece of state ( articles ) which is an array of articles, and initialize its default value to an empty array:
Step 6. Define a handleSearch() function that calls the search function to get the search results and update the articles state with these results:
Step 7. Return JSX that includes a logo, a heading, a SearchBar component, and an ArticleList component.
In the JSX:
- Pass the handleSearch function to the onSearch prop of the SearchBar component.
- Pass the articles state as a prop to the ArticleList component.
SearchBar component
The SearchBar component will allow users to enter a search term and run a function to call the API for searching:
Step 1. Import the useState function from the react library:
Step 2. Define the SearchBar component that accepts a function onSearch as a prop:
Step 3. Define a searchTerm state for the SearchBar component and initialize its default value to an empty string:
Step 4. Create an event handler that handles the submit event:
In the submit event, we call the e.preventDefault() to prevent the whole page from reloading when users submit the form and call the onSearch function with the searchTerm state as the argument.
Step 5. Return JSX that includes a form and an input element:
In the JSX :
- Wire the handleSubmit event handler to the onSubmit prop of the form.
- Call the setSearchTerm to update the state in the change event handler of the input element. The e.target .value returns the current value of the input element. The setSearchTerm function will assign a new input value to the searchTerm state of the component.
Step 6. Export the SearchBar component using a default export:
ArticleList component
The ArticleList component displays a list of Article components:
Step 1. Import the Article component:
Step 2. Define the ArticleList component that accepts an array of articles as a prop and renders each article using the Article component:
Step 3. Export the ArticleList component as a default component:
Article component
The Article component renders an article:
Step 1. Import the stripHTML function from the util library:
Step 2. Create an Article component that renders the article prop:
In the Article component:
- Construct a URL to an article on Wikipedia.
- Strip HTML tags from the title and snippet of the article object.
- Return the <article> JSX element.
Step 3. Export the Article component as a default export:
Download the Wiki Search source code.
- Use native browser Fetch API to call an external API.
How to use useLoaderdata hook in React router
Free System Design Interview Course
Many candidates are rejected or down-leveled due to poor performance in their System Design Interview. Stand out in System Design Interviews and get hired in 2024 with this popular free course.
React adopts a component-based architecture that allows developers to create reusable code sections. Hooks are the latest addition to React, which enables state management. They make our code concise, readable, and maintainable by adding state and other features to functional components. One of the hooks we will be going over is the useLoaderData hook. This hook greatly increases the user experience by loading any data from your routes even before they are rendered, preventing any empty states.
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
40 JavaScript Projects for Beginners – Easy Ideas to Get Started Coding JS
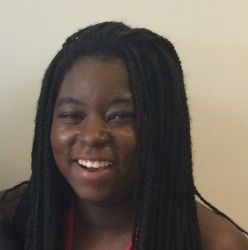
The best way to learn a new programming language is to build projects.
I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript.
My advice for tutorials would be to watch the video, build the project, break it apart and rebuild it your own way. Experiment with adding new features or using different methods.
That will test if you have really learned the concepts or not.
You can click on any of the projects listed below to jump to that section of the article.
Vanilla JavaScript Projects
How to create a color flipper.
- How to create a counter
- How to create a review carousel
- How to create a responsive navbar
- How to create a sidebar
- How to create a modal
How to create a FAQ page
How to create a restaurant menu page, how to create a video background, how to create a navigation bar on scroll, how to create tabs that display different content, how to create a countdown clock, how to create your own lorem ipsum, how to create a grocery list, how to create an image slider, how to create a rock paper scissors game, how to create a simon game, how to create a platformer game.
- How to create Doodle Jump
- How to create Flappy Bird
- How to create a Memory game
- How to create a Whack-a-mole game
- How to create Connect Four game
- How to create a Snake game
- How to create a Space Invaders game
- How to create a Frogger game
- How to create a Tetris game
React Projects
How to build a tic-tac-toe game using react hooks, how to build a tetris game using react hooks, how to create a birthday reminder app.
- How to create a tours page
How to create an accordion menu
How to create tabs for a portfolio page, how to create a review slider, how to create a color generator, how to create a stripe payment menu page, how to create a shopping cart page, how to create a cocktail search page, typescript projects, how to build a quiz app with react and typescript, how to create an arkanoid game with typescript.
If you have not learned JavaScript fundamentals, then I would suggest watching this course before proceeding with the projects.
Many of the screenshots below are from here .

In this John Smilga tutorial , you will learn how to create a random background color changer. This is a good project to get you started working with the DOM.
In Leonardo Maldonado's article on why it is important to learn about the DOM, he states:
By manipulating the DOM, you have infinite possibilities. You can create applications that update the data of the page without needing a refresh. Also, you can create applications that are customizable by the user and then change the layout of the page without a refresh.
Key concepts covered:
- document.getElementById()
- document.querySelector()
- addEventListener()
- document.body.style.backgroundColor
- Math.floor()
- Math.random()
- array.length
Before you get started, I would suggest watching the introduction where John goes over how to access the setup files for all of his projects.
How to create a Counter
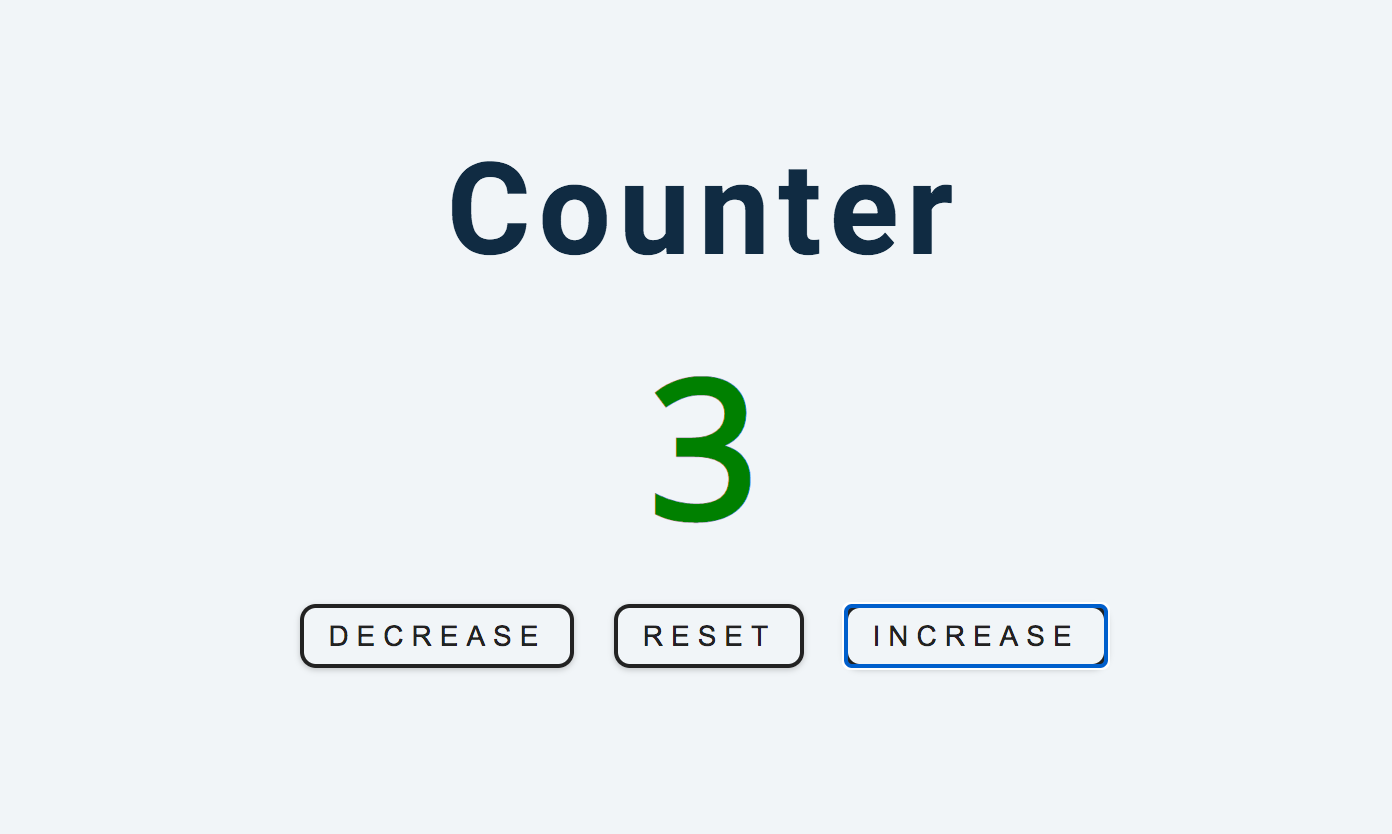
In this John Smilga tutorial , you will learn how to create a counter and write conditions that change the color based on positive or negative numbers displayed.
This project will give you more practice working with the DOM and you can use this simple counter in other projects like a pomodoro clock.
- document.querySelectorAll()
- currentTarget property
- textContent
How to create a Review carousel

In this tutorial , you will learn how to create a carousel of reviews with a button that generates random reviews.
This is a good feature to have on an ecommerce site to display customer reviews or a personal portfolio to display client reviews.
- DOMContentLoaded
How to create a responsive Navbar
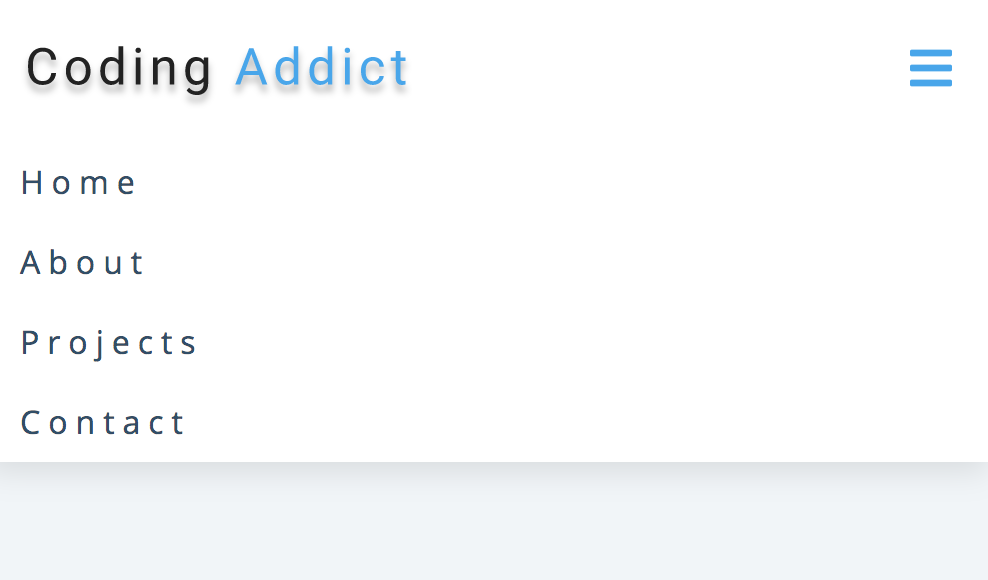
In this tutorial , you will learn how to create a responsive navbar that will show the hamburger menu for smaller devices.
Learning how to develop responsive websites is an important part of being a web developer. This is a popular feature used on a lot of websites.
- classList.toggle()
How to create a Sidebar

In this tutorial , you will learn how to create a sidebar with animation.
This is a cool feature that you can add to your personal website.
- classList.remove()
How to create a Modal
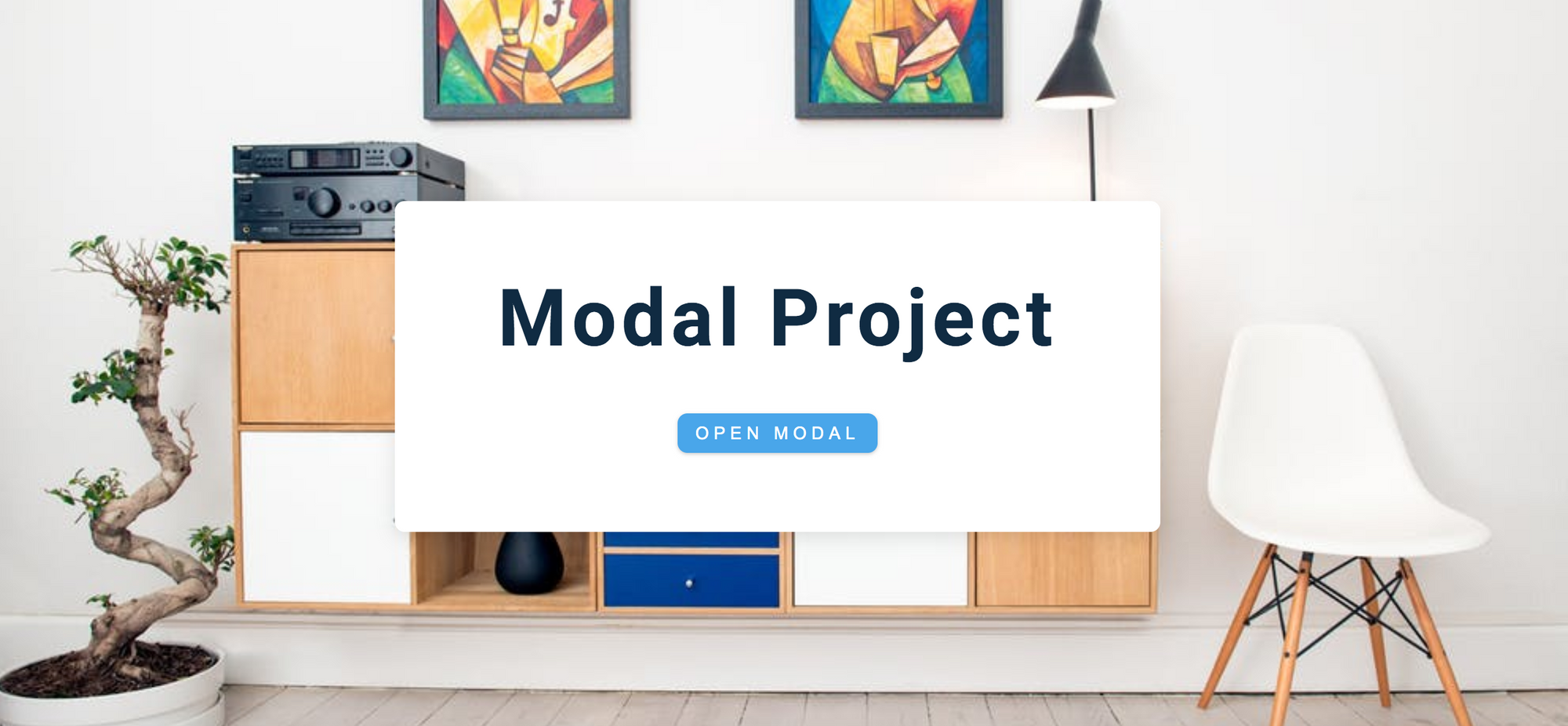
In this tutorial , you will learn how to create a modal window which is used on websites to get users to do or see something specific.
A good example of a modal window would be if a user made changes in a site without saving them and tried to go to another page. You can create a modal window that warns them to save their changes or else that information will be lost.
- classList.add()
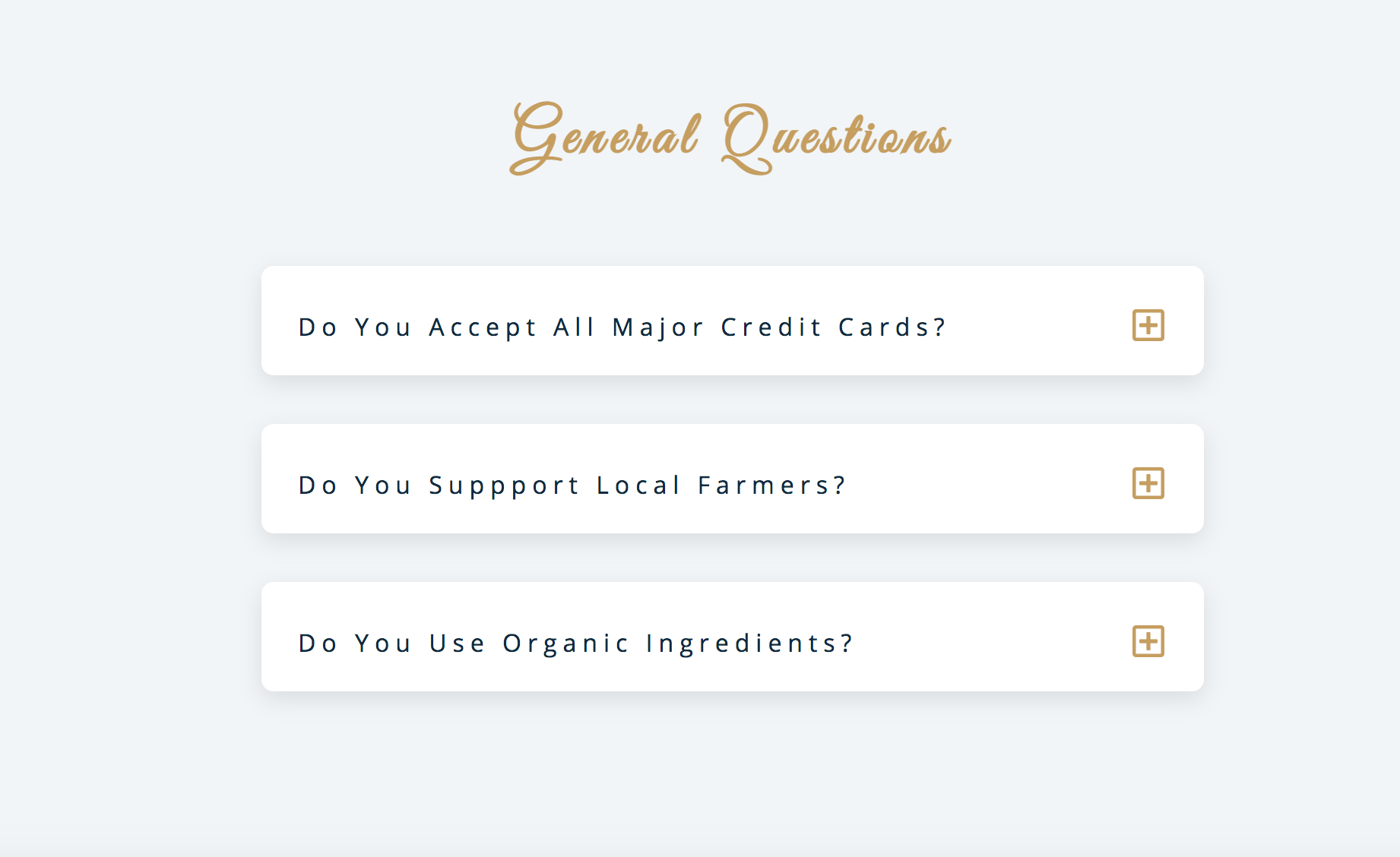
In this tutorial , you will learn how to create a frequently asked questions page which educates users about a business and drives traffic to the website through organic search results.

In this tutorial , you will learn how to make a restaurant menu page that filters through the different food menus. This is a fun project that will teach you higher order functions like map, reduce, and filter.
In Yazeed Bzadough's article on higher order functions, he states:
the greatest benefit of HOFs is greater reusability.
- map, reduce, and filter
- includes method

In this tutorial , you will learn how to make a video background with a play and pause feature. This is a common feature found in a lot of websites.
- classList.contains()
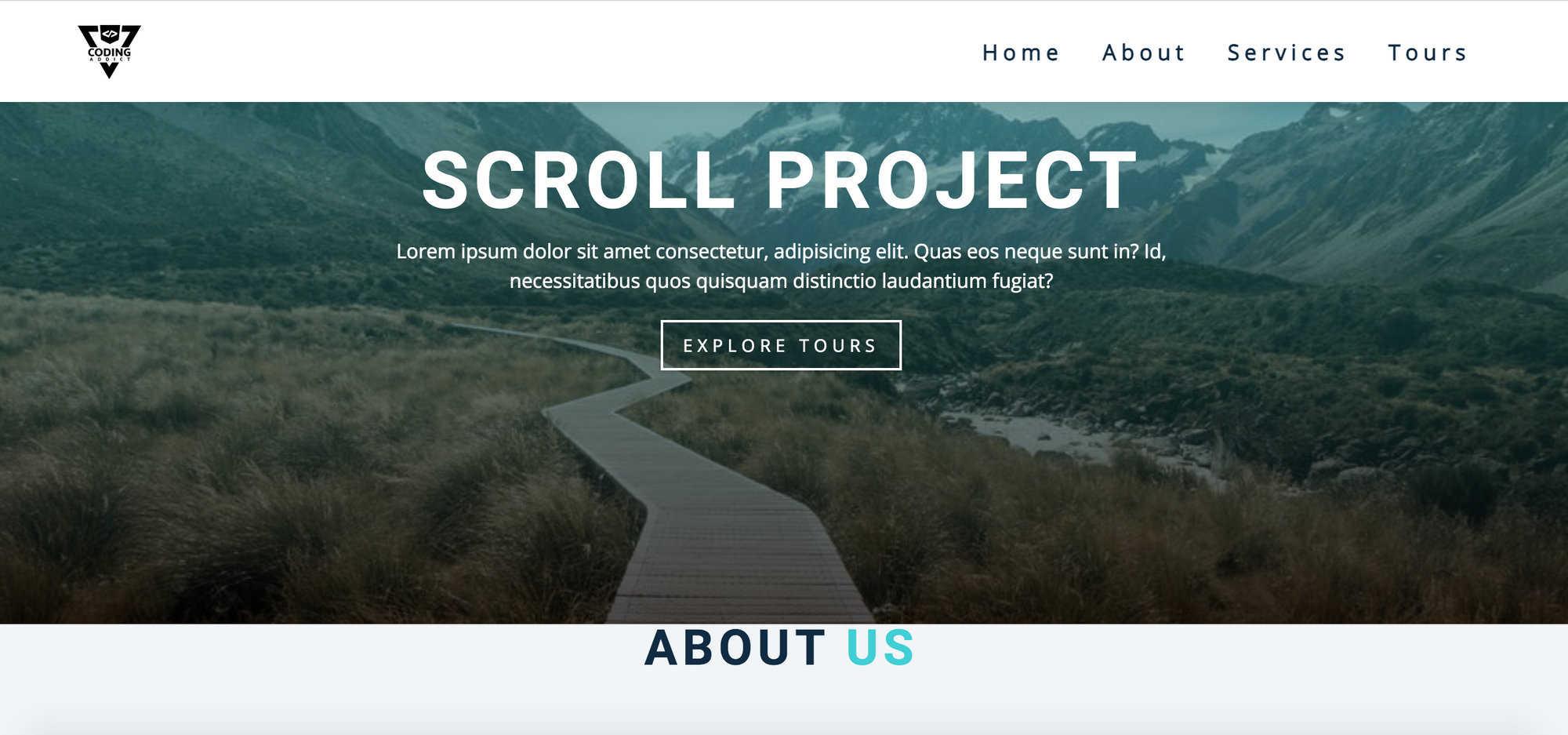
In this tutorial , you will learn how to create a navbar that slides down when scrolling and then stays at a fixed position at a certain height.
This is a popular feature found on many professional websites.
- getFullYear()
- getBoundingClientRect()
- slice method
- window.scrollTo()

In this tutorial , you will learn how to create tabs that will display different content which is useful when creating single page applications.
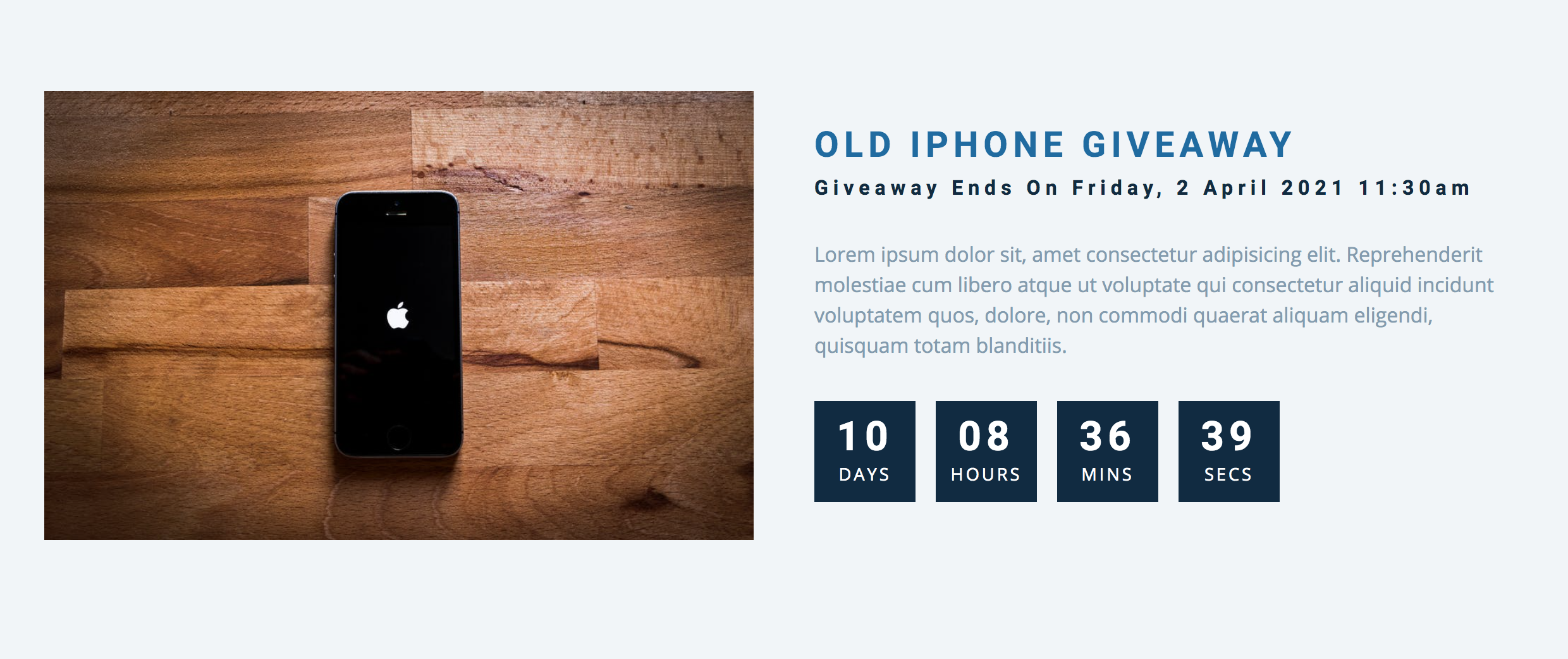
In this tutorial , you will learn how to make a countdown clock which can be used when a new product is coming out or a sale is about to end on an ecommerce site.
- setInterval()
- clearInterval()
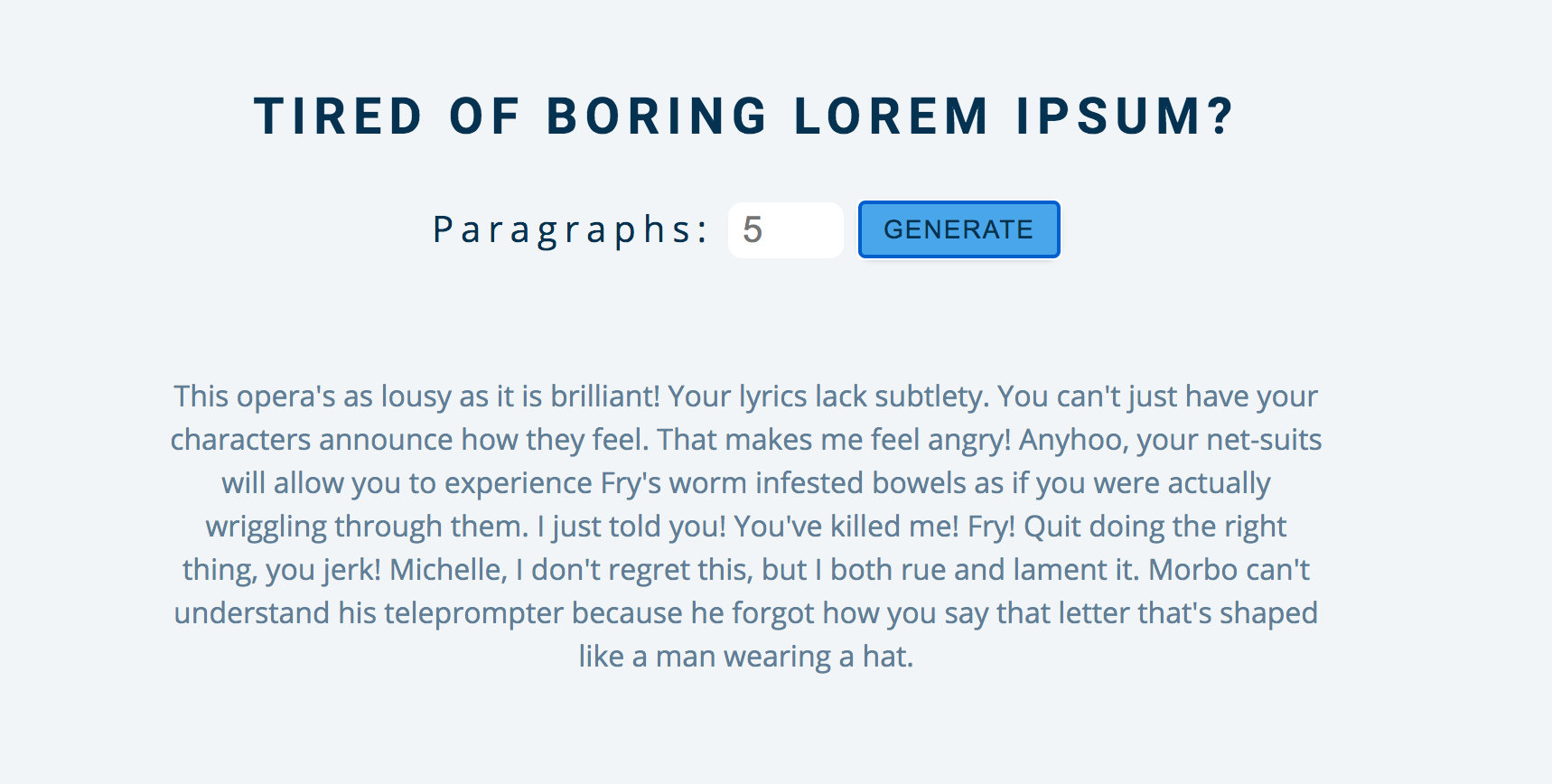
In this tutorial , you will learn how to create your own Lorem ipsum generator.
Lorem ipsum is the go to placeholder text for websites. This is a fun project to show off your creativity and create your own text.
- event.preventDefault()

In this tutorial , you will learn how to update and delete items from a grocery list and create a simple CRUD (Create, Read, Update, and Delete) application.
CRUD plays a very important role in developing full stack applications. Without it, you wouldn't be able to do things like edit or delete posts on your favorite social media platform.
- createAttribute()
- setAttributeNode()
- appendChild()
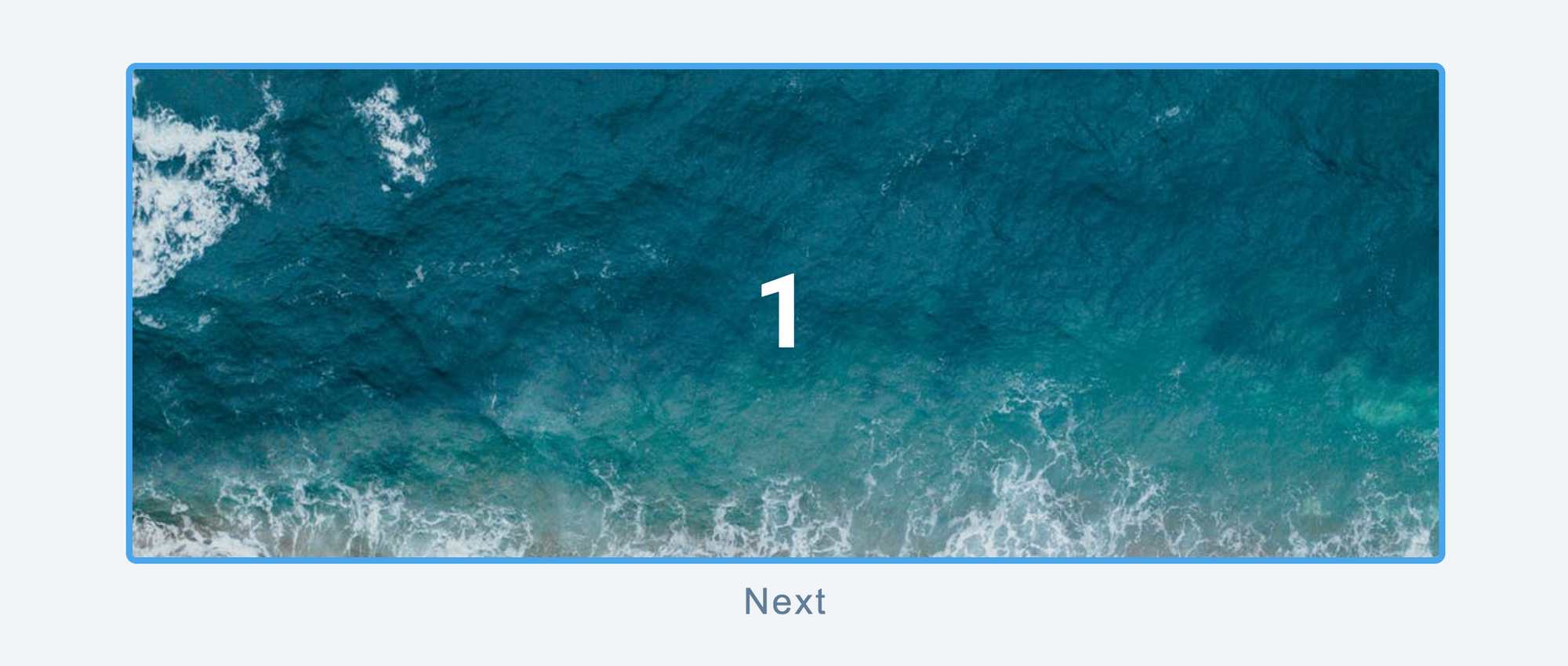
In this tutorial , you will learn how to build an image slider that you can add to any website.
- querySelectorAll()
- if/else statements
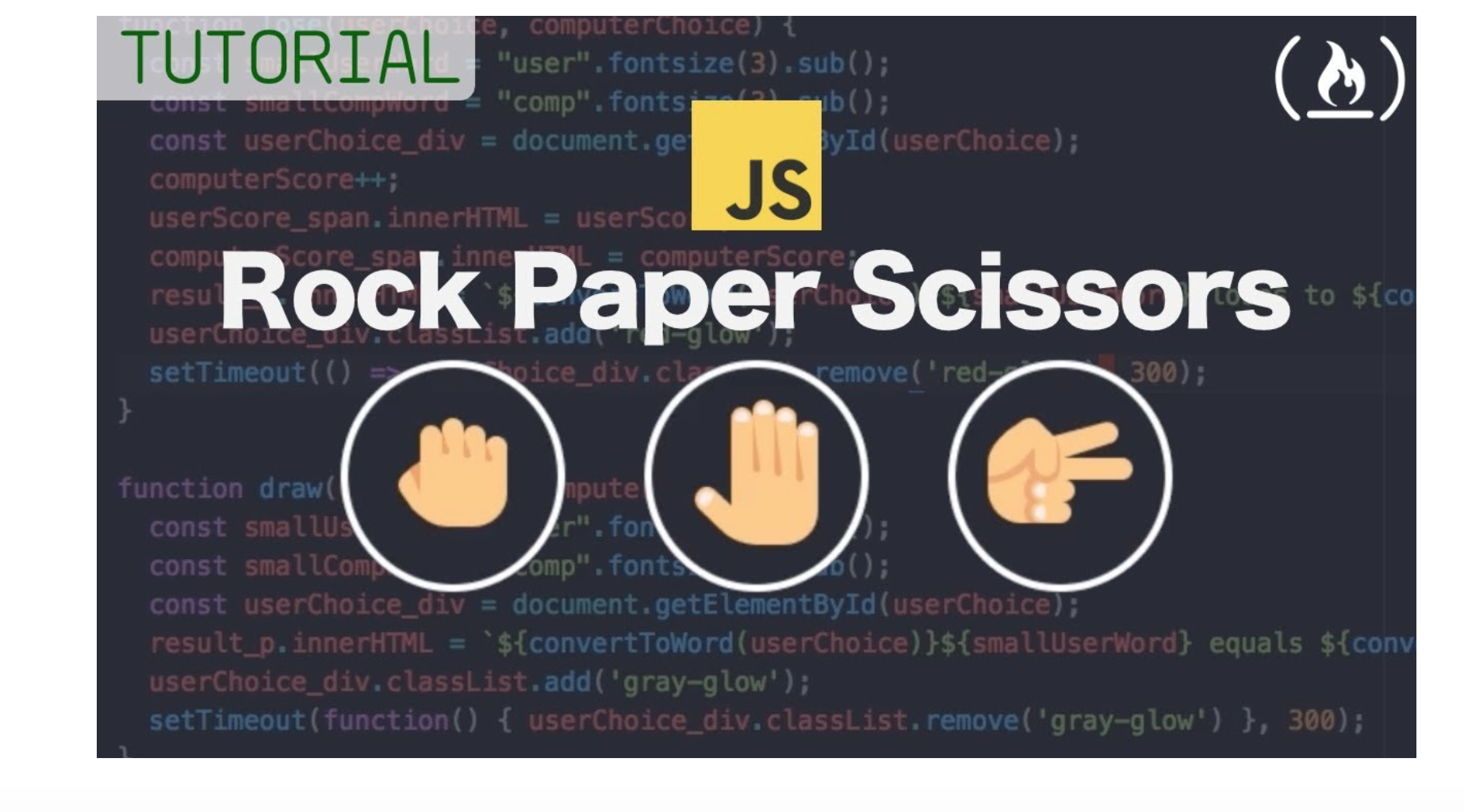
In this tutorial , Tenzin will teach you how to create a Rock Paper Scissors game. This is a fun project that will give more practice working with the DOM.
- switch statements

In this tutorial , Beau Carnes will teach you how to create the classic Simon Game. This is a good project that will get you thinking about the different components behind the game and how you would build out each of those functionalities.
- querySelector()
- setTimeout()
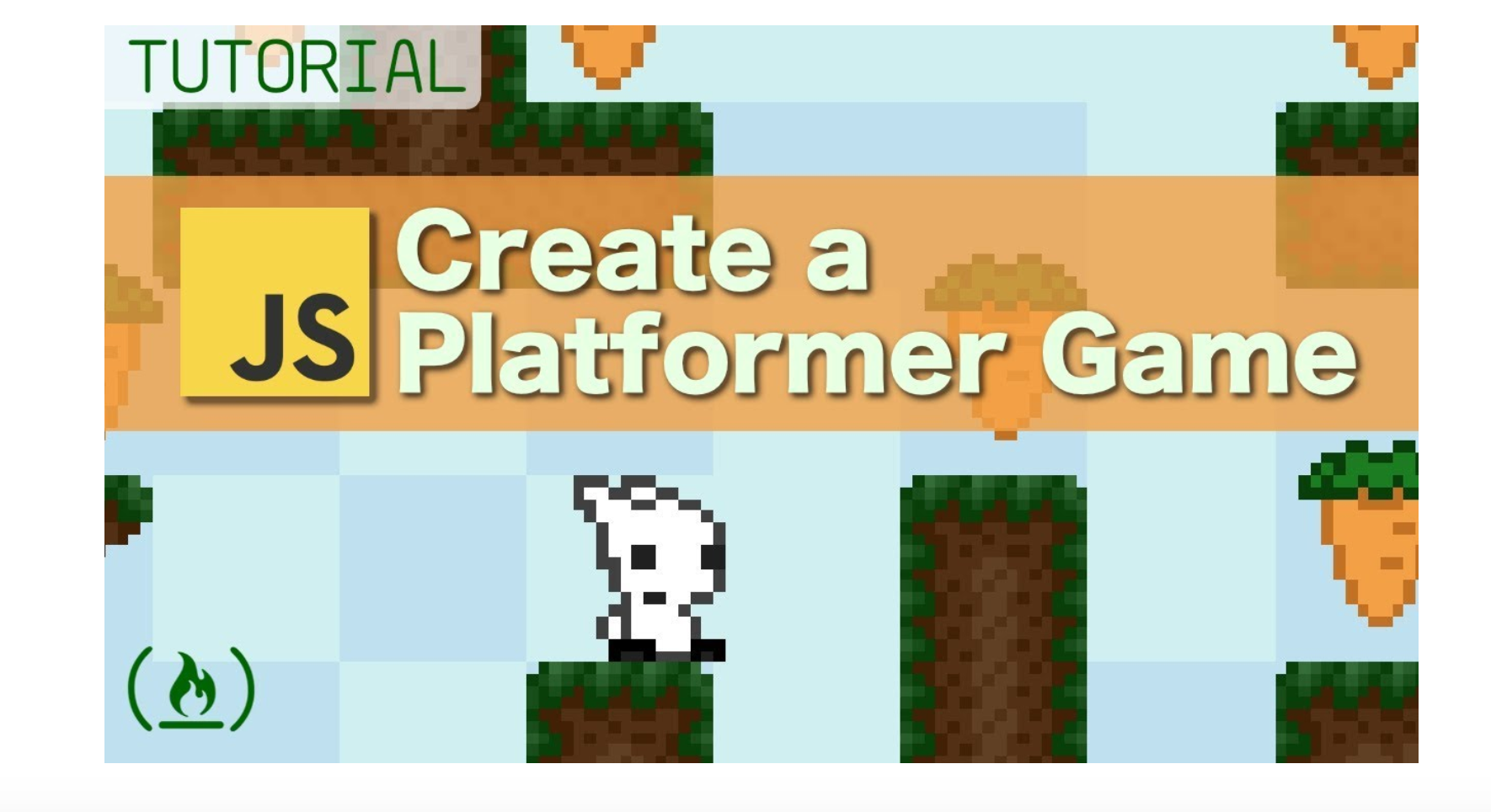
In this tutorial , Frank Poth will teach you how to build a platformer game. This project will introduce you to Object Oriented Programming principles and the Model, View, Controller software pattern.
- this keyword
- OOP principles
- MVC pattern
How to create Doodle Jump and Flappy Bird

In this video series , Ania Kubow will teach you how to build Doodle Jump and Flappy Bird .
Building games are a fun way to learn more about JavaScript and will cover many popular JavaScript methods.
- createElement()
- removeChild()
- removeEventListener()
How to create seven classic games with Ania Kubow

You will have a lot of fun creating seven games in this course by Ania Kubow:
- Memory Game
- Whack-a-mole
- Connect Four
- Space Invaders
- onclick event
- arrow functions
If you are not familiar with React fundamentals, then I would suggest taking this course before proceeding with the projects.
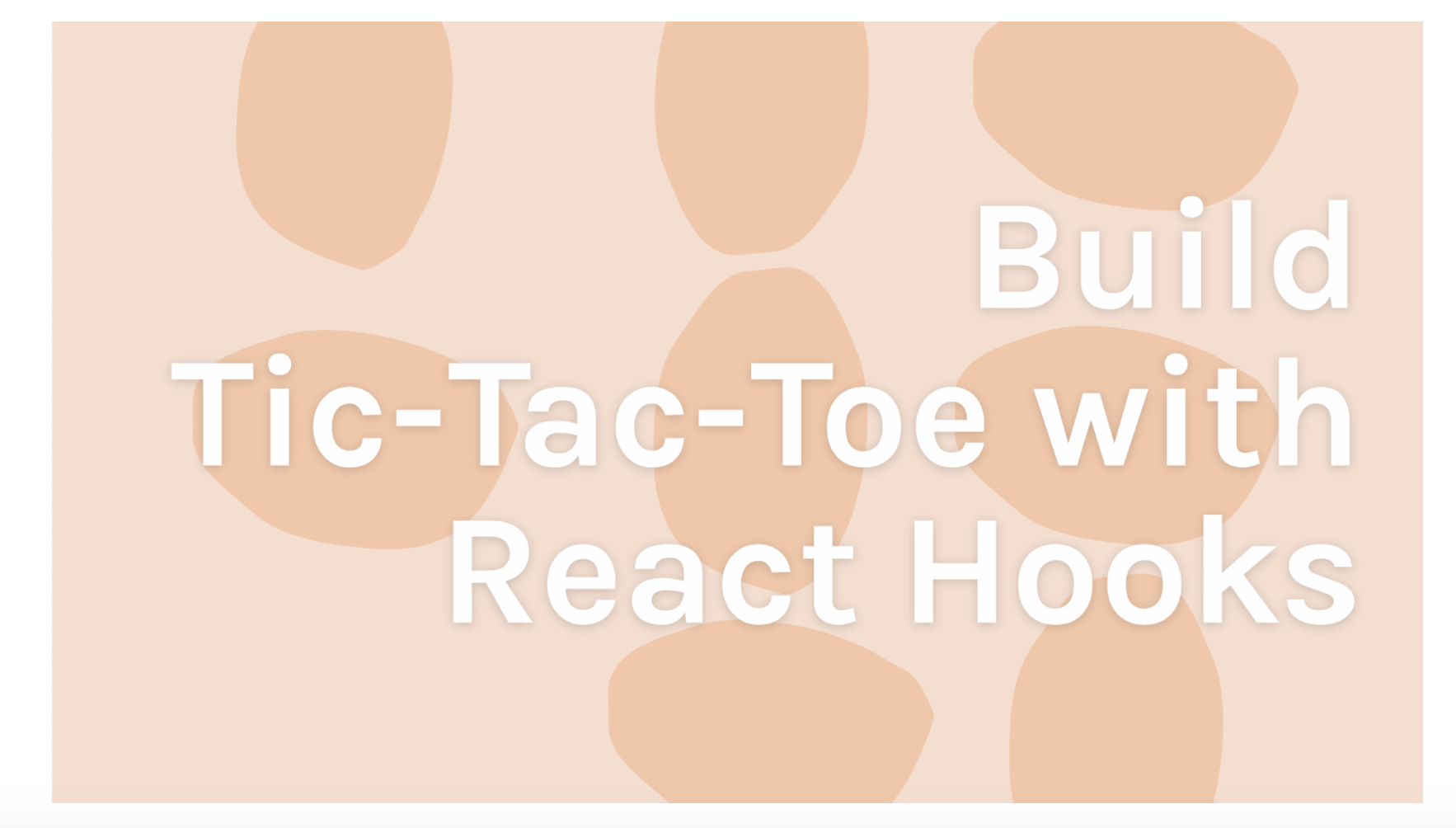
In this freeCodeCamp article , Per Harald Borgen talks about Scrimba's Tic-Tac-Toe game tutorial led by Thomas Weibenfalk. You can view the video course on Scimba's YouTube Channel.
This is a good project to start getting comfortable with React basics and working with hooks.
- import / export
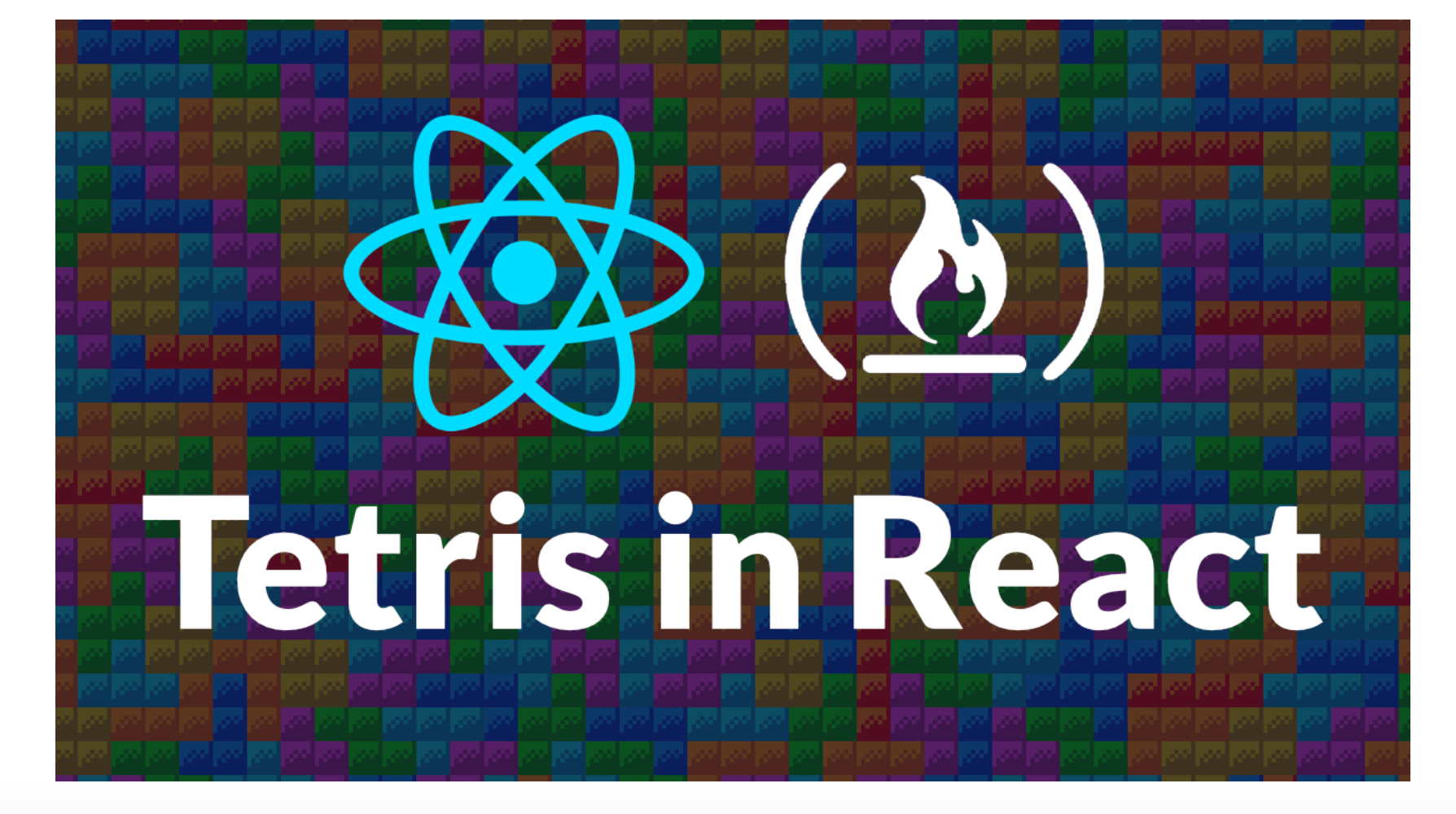
In this tutorial , Thomas Weibenfalk will teach you how to build a Tetris game using React Hooks and styled components.
- useEffect()
- useCallback()
- styled components
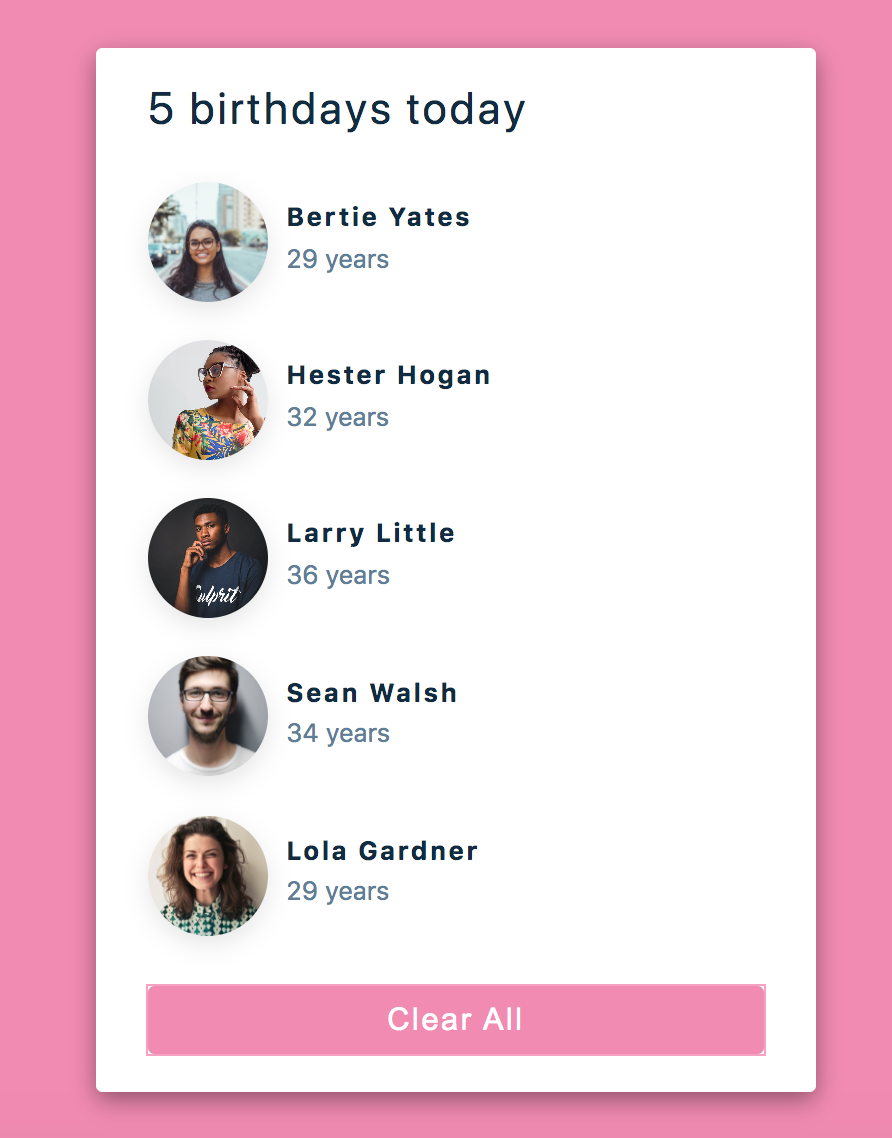
In this John Smilga course, you will learn how to create a birthday reminder app. This is a good project to start getting comfortable with React basics and working with hooks.
I would also suggest watching John's video on the startup files for this project.
How to create a Tours Page

In this tutorial , you will learn how to create a tours page where the user can delete which tours they are not interested in.
This will give you practice with React hooks and the async/await pattern.
- try...catch statement
- async/await pattern

In this tutorial , you will learn how to create a questions and answers accordion menu. These menus can be helpful in revealing content to users in a progressive way.
- React icons

In this tutorial , you will learn how to create tabs for a mock portfolio page. Tabs are useful when you want to display different content in single page applications.

In this tutorial , you will learn how to create a review slider that changes to a new review every few seconds.
This is a cool feature that you can incorporate into an ecommerce site or portfolio.

In this tutorial , you will learn how to create a color generator. This is a good project to continue practicing working with hooks and setTimeout.
- clearTimeout()

In this tutorial , you will learn how to create a Stripe payment menu page. This project will give you good practice on how to design a product landing page using React components.
- useContext()

In this tutorial , you will learn how to create a shopping cart page that updates and deletes items. This project will also be a good introduction to the useReducer hook.
- <svg> elements
- useReducer()
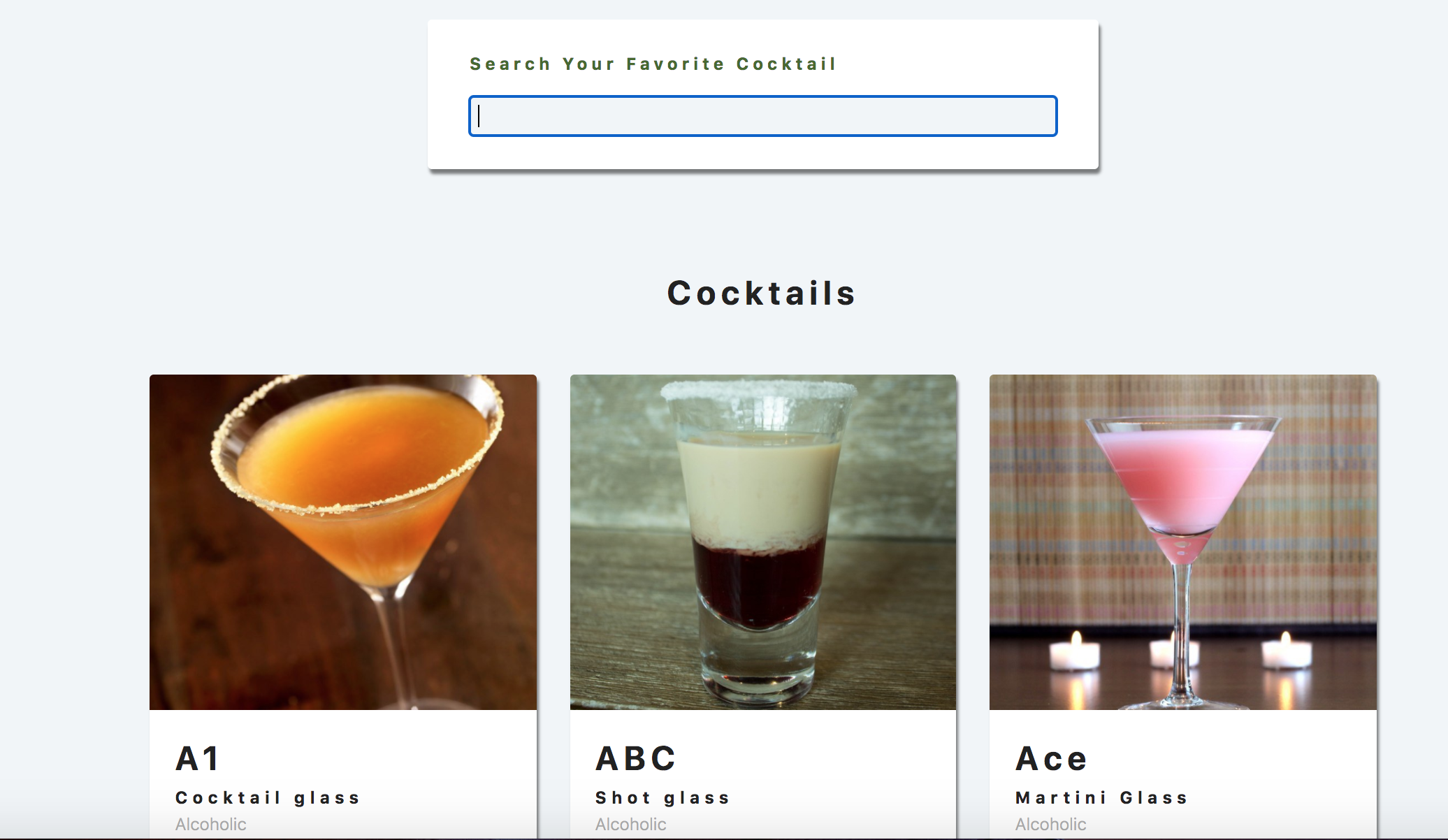
In this tutorial , you will learn how to create a cocktail search page. This project will give you an introduction to how to use React router.
React router gives you the ability to create a navigation on your website and change views to different components like an about or contact page.
- <Router>
- <Switch>
If you are unfamiliar with TypeScript, then I would suggest watching this course before proceeding with this project.
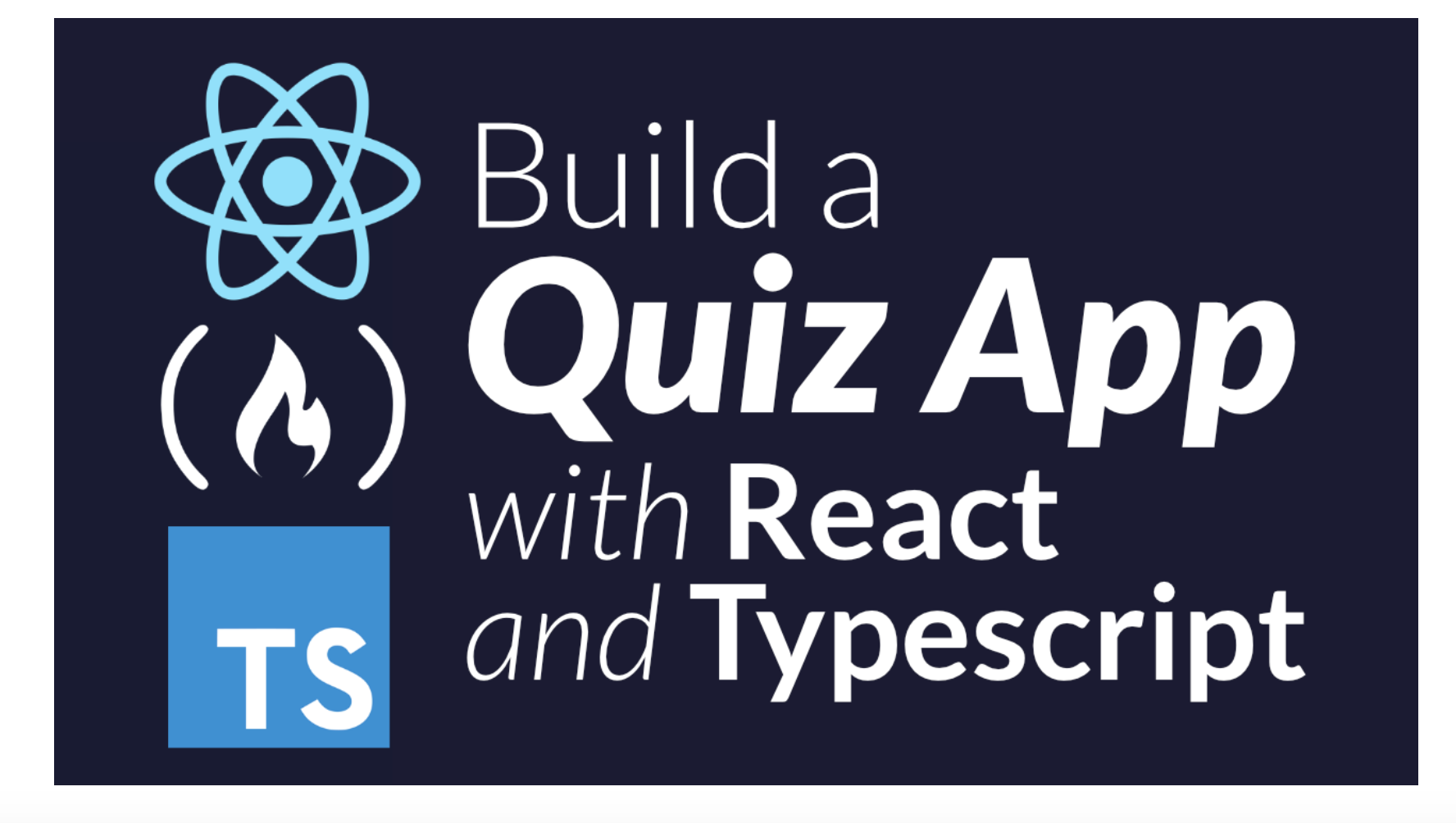
In this tutorial , Thomas Weibenfalk will teach you how to build a quiz app with React and TypeScript. This is a good opportunity to practice the basics of TypeScript.
- dangerouslySetInnerHTML

In this tutorial , Thomas Weibenfalk will teach you how to build the classic Arkanoid game in TypeScript. This is a good project that will give you practice working with the basic concepts for TypeScript.
- HTMLCanvasElement
I hope you enjoy this list of 40 project tutorials in Vanilla JavaScript, React and TypeScript.
Happy coding!
I am a musician and a programmer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Developing Oracle JET Apps Using Virtual DOM Architecture
- Use Oracle JET Components and Data Providers
5 Use Oracle JET Components and Data Providers
Review the following recommendations to make effective use of Oracle JET components and associated APIs, such as data providers, so that the app that you develop is performant and provides an optimal user experience.
Access Subproperties of Oracle JET Component Properties
JSX does not support the dot notation that allows you to access a subproperty of a component property. You cannot, for example, use the following syntax to access the max or count-by subproperties of the Input Text element’s length property.
To access these subproperties using JSX, first access the element’s top-level property and set values for the subproperties you want to specify. For example, for the countBy and max subproperties of the Oracle JET oj-input-text element’s length property, import the component props that the Input Text element uses. Then define a length object, based on the type InputTextProps["length"] , and assign it values for the countBy and max subproperties. Finally, pass the length object to the oj-input-text element as the value of its length property.
Mutate Properties on Oracle JET Custom Element Events
Unlike typical Preact components, mutating properties on JET custom elements invokes property-changed callbacks. As a result, you can end up with unexpected behavior, such as infinite loops, if you:
- Have a property-changed callback
- The property-changed callback triggers a state update
- The state update creates a new property value (for example, copies values into a new array)
- The new property value is routed back into the same property
Typically, Preact (and React components) invoke callbacks in response to user interaction, and not in response to a new property value being passed in by the parent component. You can simulate the Preact componnent-type behavior when you use JET custom elements if you check the value of the event.detail.updatedFrom field to determine if the property change is due to user interaction ( internal ) instead of your app programmatically mutating the property value ( external ). For example, the following event callback is only invoked in response to user interaction:
Avoid Repeated Data Provider Creation
Do not re-create a data provider each time that a VComponent renders.
For example, do not do the following in a VComponent using an oj-list-view component, as you re-create the MutableArrayDataProvider instance each time the VComponent renders:
Instead, consider using Preact's useMemo hook to ensure that a data provider instance is re-created only if the data in the data provider actually changes. The following example demonstrates how you include the useMemo hook to ensure that the data provider providing data to an oj-list-view component is not re-created, even if the VComponent that includes this code re-renders.
The reason for this recommendation is that a VComponent can re-render for any number of reasons, but as long as the data provided by the data provider does not change, there is no need to incur the cost of re-creating the data provider instance. Re-creating a data provider can affect your app's performance, due to unnecessary rendering, and usability as collection components may flash and lose scroll position as they re-render.
Avoid Data Provider Re-creation When Data Changes
Previously, we described how the useMemo hook avoids re-creating a data provider unless data changes. When data does change, we still end up re-creating the data provider instance, and this can result in collection components, such as list view, re-rendering all data displayed by the component and losing scroll position.
Here, we'll try to illustrate how you can opitimize the user experience by implementing fine-grained updates and maintaining scroll position for collection components, even if the data referenced by the data provider changes. To accomplish this, the data provider uses a MutableArrayDataProvider instance. With a MutableArrayDataProvider instance, you can mutate an existing instance by setting a new array value into the MutableArrayDataProvider 's data field. The collection component is notified of the specific change (create, update, or delete) that occurs, which allows it to make a fine-grained update and maintain scroll position.
In the following example, an app displays a list of tasks in a list view component and a Done button that allows a user to remove a completed task. These components render in the Content component of a virtual DOM app created with the basic template ( appRootDir/src/components/content/index.tsx ).

To implement fine-grained updates and maintain scroll position for the oj-list-view component, we store the list view's MutableArrayDataProvider in local state using Preact’s useState hook and never re-create it, and we also use Preact’s useEffect hook to update the data field of the MutableArrayDataProvider when a change to the list of tasks is detected. The user experience is that a click on Done for an item in the tasks list removes the item (with removal animation). No refresh of the oj-list-view component or scroll position loss occurs.
Use Oracle JET Popup and Dialog Components
To use Oracle JET's popup or dialog components (popup content) in a VComponent or a virtual DOM app, you need to create a reference to the popup content. We recommend too that you place the popup content on its own within a div element so that it continues to work when used with Preact's reconciliation logic.
Currently, to launch popup content from within JSX, you must create a reference to the custom element and manually call open() , as in the following example for a VComponent class component that uses Preact's createRef function:
As a side effect of the open() call, Oracle JET relocates the popup content DOM to a JET-managed popup container, outside of the popup-launching component. This works and the user can see and interact with the popup.
If, while the popup is open, the popup-launching component is re-rendered by, for example, a state change, Preact's reconciliation logic detects that the popup content element is no longer in its original location and will reparent the still-open popup content back to its original parent. This interferes with JET's popup service, and unfortunately leads to non-functional popup content. To avoid this issue, we recommend that you ensure that the popup content is the only child of its parent element. In the following functional component example, we illustrate one way to accomplish this by placing the oj-dialog component within its own div element.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript - html dom methods.
HTML DOM methods are actions you can perform (on HTML Elements).
HTML DOM properties are values (of HTML Elements) that you can set or change.
The DOM Programming Interface
The HTML DOM can be accessed with JavaScript (and with other programming languages).
In the DOM, all HTML elements are defined as objects .
The programming interface is the properties and methods of each object.
A property is a value that you can get or set (like changing the content of an HTML element).
A method is an action you can do (like add or deleting an HTML element).
The following example changes the content (the innerHTML ) of the <p> element with id="demo" :
In the example above, getElementById is a method , while innerHTML is a property .
The getElementById Method
The most common way to access an HTML element is to use the id of the element.
In the example above the getElementById method used id="demo" to find the element.
The innerHTML Property
The easiest way to get the content of an element is by using the innerHTML property.
The innerHTML property is useful for getting or replacing the content of HTML elements.
The innerHTML property can be used to get or change any HTML element, including <html> and <body> .

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

IMAGES
VIDEO
COMMENTS
JavaScript DOM [13 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.Go to the editor] . 1. Here is a sample html file with a submit button. Now modify the style of the paragraph text through javascript code.
The HTML DOM is a standard object model and programming interface for HTML. It defines: The HTML elements as objects. The properties of all HTML elements. The methods to access all HTML elements. The events for all HTML elements. In other words: The HTML DOM is a standard for how to get, change, add, or delete HTML elements.
Video 3: Exercise 2. See the code and full exercise on Codepen. To make the ordering of the plans more logical, using JavaScript, move the basic plan to be before (to the left) of the pro plan. In Video 3 we had a simple pricing table with two products, a basic and pro plan and this was an exercise in moving elements around in the DOM.
The Document Object Model (DOM) connects web pages to scripts or programming languages by representing the structure of a document—such as the HTML representing a web page—in memory. Usually it refers to JavaScript, even though modeling HTML, SVG, or XML documents as objects are not part of the core JavaScript language.
The JavaScript DOM Manipulation Handbook. DOM Manipulation is one of the most exciting topics to learn about in JavaScript. This is because one of JavaScript's main uses is to make web pages interactive - and the Document Object Model (DOM) plays a major role in this. The DOM is a powerful tool that allows you to interact with and manipulate ...
There are different ways to assign event handlers in JavaScript: HTML Attribute: You can set an event handler directly in the HTML code using an attribute like onclick, onmouseover, etc. <button onclick="alert('Button clicked!')">Click me</button> 2. DOM Property: You can assign a handler using a DOM property like onclick, onmouseover, and so on.
The DOM is a Web API that allows developers to use programming logic to make changes to their HTML code. It's a reliable way to make changes that turn static websites into dynamic ones. It's an important topic in web development because the DOM serves as the initial use of JavaScript in the browser. HTML code isn't considered part of the DOM ...
Manipulating documents. When writing web pages and apps, one of the most common things you'll want to do is manipulate the document structure in some way. This is usually done by using the Document Object Model (DOM), a set of APIs for controlling HTML and styling information that makes heavy use of the Document object.
This section covers the JavaScript Document Object Model (DOM) and shows you how to manipulate DOM elements effectively. Section 1. Getting started. Understanding the Document Object Model in JavaScript. Section 2. Selecting elements. getElementById () - select an element by id.
The Javascript DOM (Document Object Model) is an interface that allows developers to manipulate the content, structure and style of a website. In this article, we will learn what the DOM is and how you can manipulate it using Javascript. This article can also be used as a reference for the basic DOM operations.
Reacting to Events. A JavaScript can be executed when an event occurs, like when a user clicks on an HTML element. To execute code when a user clicks on an element, add JavaScript code to an HTML event attribute: onclick= JavaScript. Examples of HTML events: When a user clicks the mouse. When a web page has loaded. When an image has been loaded.
The Modern JavaScript DOM Cheat Sheet. Updated: March 2, 2023 By: Goodman Post a comment. This article provides a comprehensive cheat sheet for modern JavaScript DOM, covering a wide range of methods and properties that you can use to interact with the Document Object Model in your web applications. Table Of Contents.
Learn how to manipulate the Document Object Model (DOM) and handle user events with JavaScript in this lesson from The Odin Project. You will learn how to select, create, modify, and delete elements, as well as how to listen and respond to various types of events. This lesson will help you build dynamic and interactive web pages with ease.
JavaScript DOM - Code Exercises. It's really hard to test your programming knowledge after you have completed a tutorial or a lecture. We have prepared some exercises to help out beginner devs to solidify their understanding of the DOM. Every exercise has a brief description of the problem, starting code, links to relevant MDN docs, and ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
All attributes are accessible by using the following methods: elem.hasAttribute(name) - checks for existence. elem.getAttribute(name) - gets the value. elem.setAttribute(name, value) - sets the value. elem.removeAttribute(name) - removes the attribute. These methods operate exactly with what's written in HTML.
The className way. This is the simple way, storing all classes in a string. The string can easily be changed or appended. // Create a div and add a class. var new_row = document.createElement("div"); new_row.className = "aClassName"; // Add another class. A space ' ' separates class names.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
In "How To Access Elements in the DOM," we reviewed the DOM methods for grabbing and working with nodes in vanilla JavaScript. To review, document.querySelector() and document.getElementById() are the methods that are used to access a single element. Using a div with an id attribute in the example below, we can access that element either way.
Let's recreate the countdown timer from the tutorial with Alpine.js but with vainilla JavaScript. See it live and get the code ... or completing assignments, enhancing productivity and focus. Medical and Healthcare: Countdown timers are ... This is the event listener that will be triggered when the DOM is fully loaded. const countdownContainer ...
JavaScript (JS) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions. While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js, Apache CouchDB and Adobe Acrobat. JavaScript is a prototype-based, multi-paradigm, single-threaded, dynamic language, supporting object-oriented ...
The setSearchTerm function will assign a new input value to the searchTerm state of the component. Step 6. Export the SearchBar component using a default export: export default SearchBar; Code language: JavaScript (javascript) ArticleList component. The ArticleList component displays a list of Article components:
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Code explanation . The following is a line-by-line explanation of the code above: In the DataEducative.jsx file:. Line 1: We import the useLoaderData hook from the react-router-dom library. Lines 2-12: We define DataEducative, a functional component that will preload data from the router using the useLoaderData hook and assign its value to educative_dog_object_url.
40 JavaScript Projects for Beginners - Easy Ideas to Get Started Coding JS. The best way to learn a new programming language is to build projects. I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript. My advice for tutorials would be to watch the video, build the project, break it apart ...
Previous Next JavaScript must be enabled to correctly display this content Developing Oracle JET Apps Using Virtual DOM Architecture; Use Oracle JET Components and Data Providers ... based on the type InputTextProps["length"], and assign it values for the countBy and max subproperties. Finally, pass the length object to the oj-input-text ...
Minnesota Twins Royce Lewis looks on from the dugout during the sixth inning against the Chicago White Sox at Target Field on April 25.
JavaScript Objects HTML DOM Objects. JavaScript Destructuring Previous Next Destructuring Assignment Syntax. The destructuring assignment syntax unpack object properties into variables: let {firstName, lastName} = person; It can also unpack arrays and any other iterables:
The HTML DOM can be accessed with JavaScript (and with other programming languages). In the DOM, all HTML elements are defined as objects. The programming interface is the properties and methods of each object. A property is a value that you can get or set (like changing the content of an HTML element). A method is an action you can do (like ...