IndexError: list assignment index out of range in Python
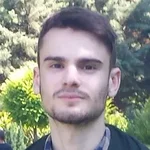
Last updated: Apr 8, 2024 Reading time · 9 min
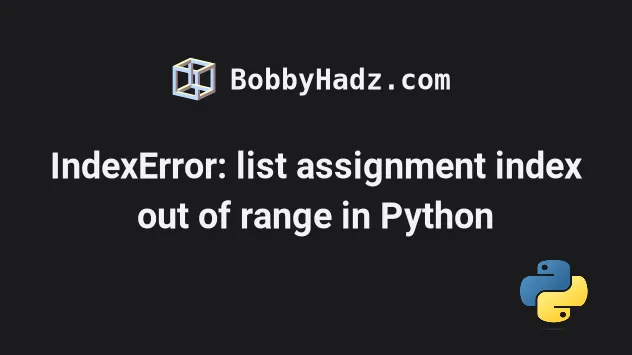

# Table of Contents
- IndexError: list assignment index out of range
- (CSV) IndexError: list index out of range
- sys.argv[1] IndexError: list index out of range
- IndexError: pop index out of range
Make sure to click on the correct subheading depending on your error message.
# IndexError: list assignment index out of range in Python
The Python "IndexError: list assignment index out of range" occurs when we try to assign a value at an index that doesn't exist in the list.
To solve the error, use the append() method to add an item to the end of the list, e.g. my_list.append('b') .

Here is an example of how the error occurs.
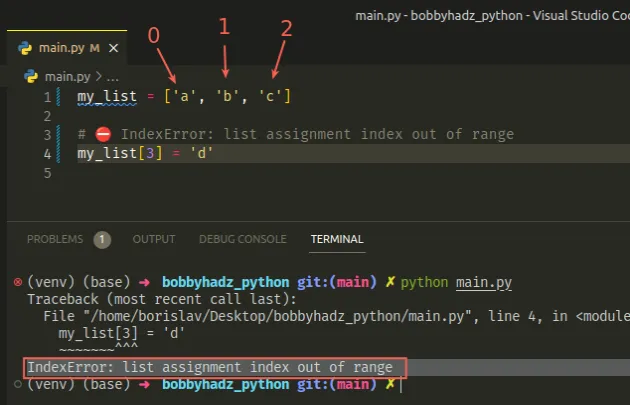
The list has a length of 3 . Since indexes in Python are zero-based, the first index in the list is 0 , and the last is 2 .
a | b | c |
---|---|---|
0 | 1 | 2 |
Trying to assign a value to any positive index outside the range of 0-2 would cause the IndexError .
# Adding an item to the end of the list with append()
If you need to add an item to the end of a list, use the list.append() method instead.
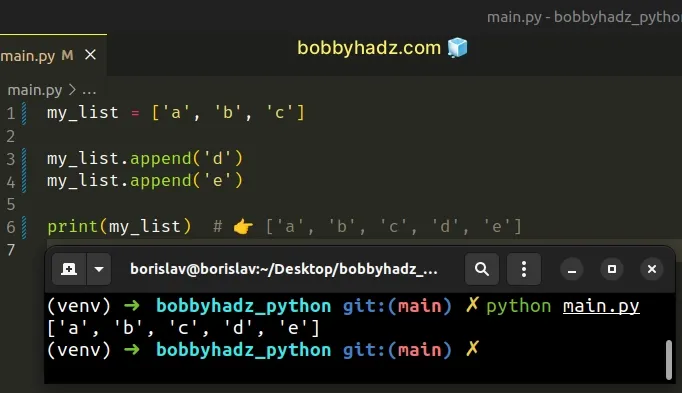
The list.append() method adds an item to the end of the list.
The method returns None as it mutates the original list.
# Changing the value of the element at the last index in the list
If you meant to change the value of the last index in the list, use -1 .
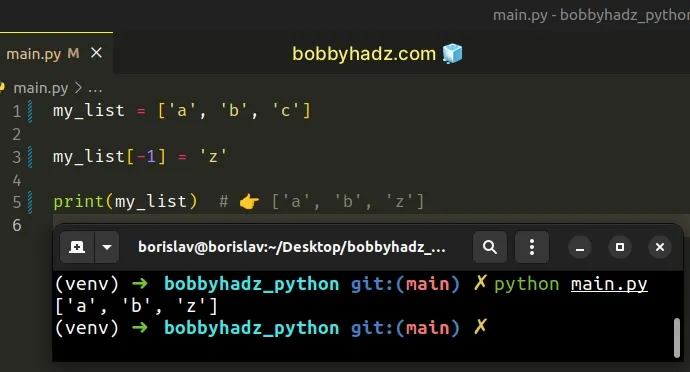
When the index starts with a minus, we start counting backward from the end of the list.
# Declaring a list that contains N elements and updating a certain index
Alternatively, you can declare a list that contains N elements with None values.
The item you specify in the list will be contained N times in the new list the operation returns.
Make sure to wrap the value you want to repeat in a list.
If the list contains a value at the specific index, then you are able to change it.
# Using a try/except statement to handle the error
If you need to handle the error if the specified list index doesn't exist, use a try/except statement.
The list in the example has 3 elements, so its last element has an index of 2 .
We wrapped the assignment in a try/except block, so the IndexError is handled by the except block.
You can also use a pass statement in the except block if you need to ignore the error.
The pass statement does nothing and is used when a statement is required syntactically but the program requires no action.
# Getting the length of a list
If you need to get the length of the list, use the len() function.
The len() function returns the length (the number of items) of an object.
The argument the function takes may be a sequence (a string, tuple, list, range or bytes) or a collection (a dictionary, set, or frozen set).
If you need to check if an index exists before assigning a value, use an if statement.
This means that you can check if the list's length is greater than the index you are trying to assign to.
# Trying to assign a value to an empty list at a specific index
Note that if you try to assign to an empty list at a specific index, you'd always get an IndexError .
You should print the list you are trying to access and its length to make sure the variable stores what you expect.
# Use the extend() method to add multiple items to the end of a list
If you need to add multiple items to the end of a list, use the extend() method.
The list.extend method takes an iterable (such as a list) and extends the list by appending all of the items from the iterable.
The list.extend method returns None as it mutates the original list.
# (CSV) IndexError: list index out of range in Python
The Python CSV "IndexError: list index out of range" occurs when we try to access a list at an index out of range, e.g. an empty row in a CSV file.
To solve the error, check if the row isn't empty before accessing it at an index, or check if the index exists in the list.

Assume we have the following CSV file.
And we are trying to read it as follows.
# Check if the list contains elements before accessing it
One way to solve the error is to check if the list contains any elements before accessing it at an index.
The if statement checks if the list is truthy on each iteration.
All values that are not truthy are considered falsy. The falsy values in Python are:
- constants defined to be falsy: None and False .
- 0 (zero) of any numeric type
- empty sequences and collections: "" (empty string), () (empty tuple), [] (empty list), {} (empty dictionary), set() (empty set), range(0) (empty range).
# Check if the index you are trying to access exists in the list
Alternatively, you can check whether the specific index you are trying to access exists in the list.
This means that you can check if the list's length is greater than the index you are trying to access.
# Use a try/except statement to handle the error
Alternatively, you can use a try/except block to handle the error.
We try to access the list of the current iteration at index 1 , and if an IndexError is raised, we can handle it in the except block or continue to the next iteration.
# sys.argv [1] IndexError: list index out of range in Python
The sys.argv "IndexError: list index out of range in Python" occurs when we run a Python script without specifying values for the required command line arguments.
To solve the error, provide values for the required arguments, e.g. python main.py first second .
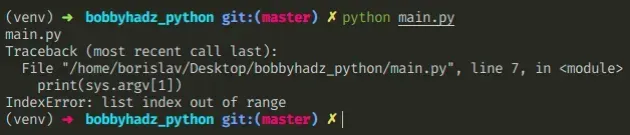
I ran the script with python main.py .
The sys.argv list contains the command line arguments that were passed to the Python script.
# Provide all of the required command line arguments
To solve the error, make sure to provide all of the required command line arguments when running the script, e.g. python main.py first second .
Notice that the first item in the list is always the name of the script.
It is operating system dependent if this is the full pathname or not.
# Check if the sys.argv list contains the index
If you don't have to always specify all of the command line arguments that your script tries to access, use an if statement to check if the sys.argv list contains the index that you are trying to access.
I ran the script as python main.py without providing any command line arguments, so the condition wasn't met and the else block ran.
We tried accessing the list item at index 1 which raised an IndexError exception.
You can handle the error or use the pass keyword in the except block.
# IndexError: pop index out of range in Python
The Python "IndexError: pop index out of range" occurs when we pass an index that doesn't exist in the list to the pop() method.
To solve the error, pass an index that exists to the method or call the pop() method without arguments to remove the last item from the list.

The list has a length of 3 . Since indexes in Python are zero-based, the first item in the list has an index of 0 , and the last an index of 2 .
If you need to remove the last item in the list, call the method without passing it an index.
The list.pop method removes the item at the given position in the list and returns it.
You can also use negative indices to count backward, e.g. my_list.pop(-1) removes the last item of the list, and my_list.pop(-2) removes the second-to-last item.
Alternatively, you can check if an item at the specified index exists before passing it to pop() .
This means that you can check if the list's length is greater than the index you are passing to pop() .
An alternative approach to handle the error is to use a try/except block.
If calling the pop() method with the provided index raises an IndexError , the except block is run, where we can handle the error or use the pass keyword to ignore it.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- IndexError: index 0 is out of bounds for axis 0 with size 0
- IndexError: invalid index to scalar variable in Python
- IndexError: pop from empty list in Python [Solved]
- Replacement index 1 out of range for positional args tuple
- IndexError: too many indices for array in Python [Solved]
- IndexError: tuple index out of range in Python [Solved]
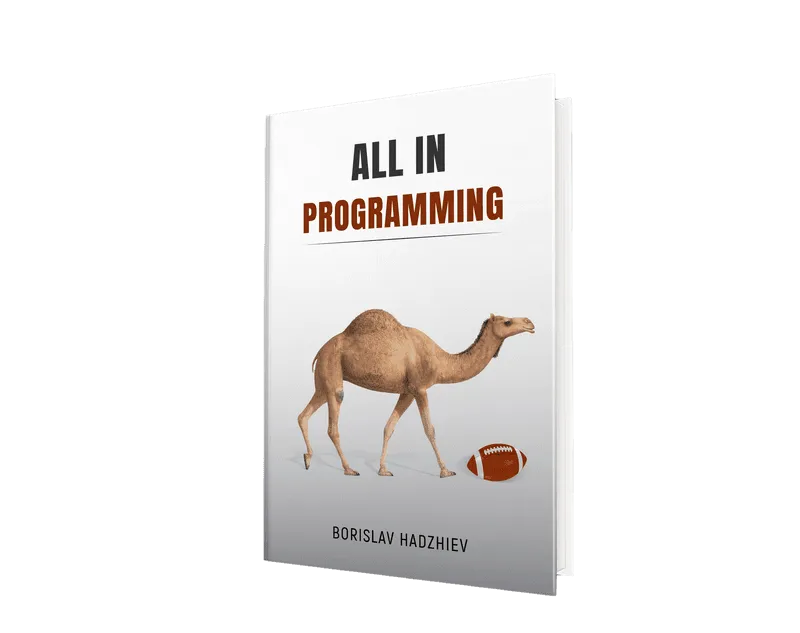
Borislav Hadzhiev
Web Developer
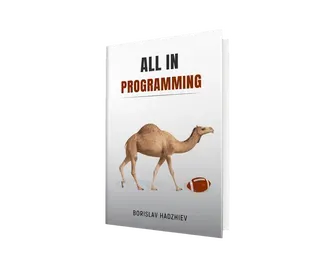
Copyright © 2024 Borislav Hadzhiev
How to fix IndexError: list assignment index out of range in Python
This tutorial will show you an example that causes this error and how to fix it in practice
How to reproduce this error
Because the list has two items, the index number ranges from 0 to 1. Assigning a value to any other index number will cause this error.
How to fix this error
Take your skills to the next level ⚡️.

- Documentation
- System Status
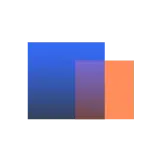
- Rollbar Academy
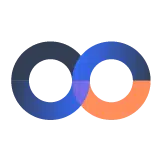
- Software Development
- Engineering Management
- Platform/Ops
- Customer Support
- Software Agency
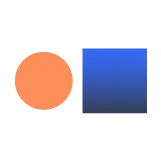
- Low-Risk Release
- Production Code Quality
- DevOps Bridge
- Effective Testing & QA
How to Fix “IndexError: List Assignment Index Out of Range” in Python
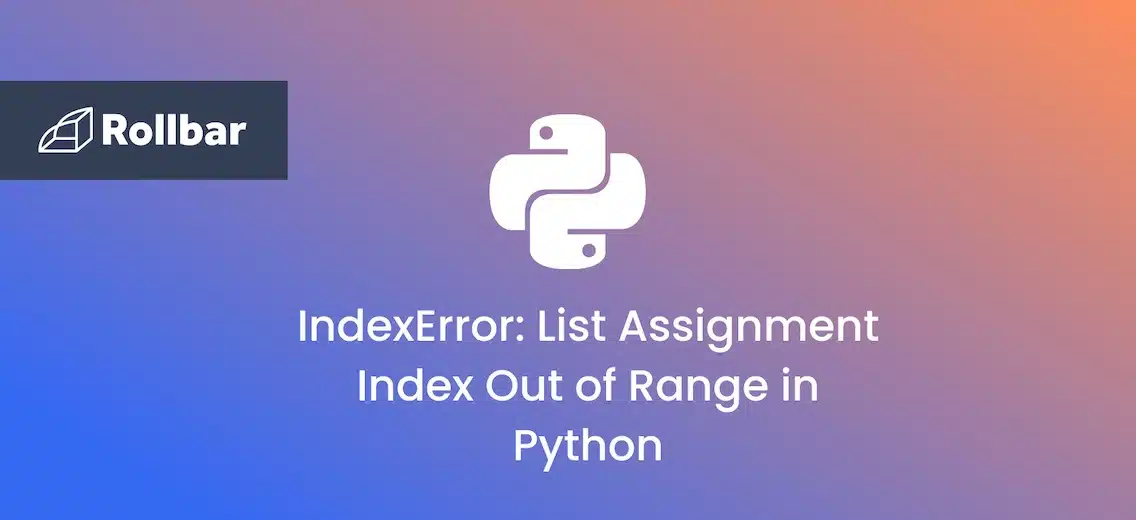
Table of Contents
The IndexError: List Assignment Index Out of Range error occurs when you assign a value to an index that is beyond the valid range of indices in the list. As Python uses zero-based indexing, when you try to access an element at an index less than 0 or greater than or equal to the list’s length, you trigger this error.
It’s not as complicated as it sounds. Think of it this way: you have a row of ten mailboxes, numbered from 0 to 9. These mailboxes represent the list in Python. Now, if you try to put a letter into mailbox number 10, which doesn't exist, you'll face a problem. Similarly, if you try to put a letter into any negative number mailbox, you'll face the same issue because those mailboxes don't exist either.
The IndexError: List Assignment Index Out of Range error in Python is like trying to put a letter into a mailbox that doesn't exist in our row of mailboxes. Just as you can't access a non-existent mailbox, you can't assign a value to an index in a list that doesn't exist.
Let’s take a look at example code that raises this error and some strategies to prevent it from occurring in the first place.
Example of “IndexError: List Assignment Index Out of Range”
Remember, assigning a value at an index that is negative or out of bounds of the valid range of indices of the list raises the error.
How to resolve “IndexError: List Assignment Index Out of Range”
You can use methods such as append() or insert() to insert a new element into the list.
How to use the append() method
Use the append() method to add elements to extend the list properly and avoid out-of-range assignments.
How to use the insert() method
Use the insert() method to insert elements at a specific position instead of direct assignment to avoid out-of-range assignments.
Now one big advantage of using insert() is even if you specify an index position which is way out of range it won’t give any error and it will just append the element at the end of the list.
Track, Analyze and Manage Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing Python errors easier than ever. Try it today !
Related Resources
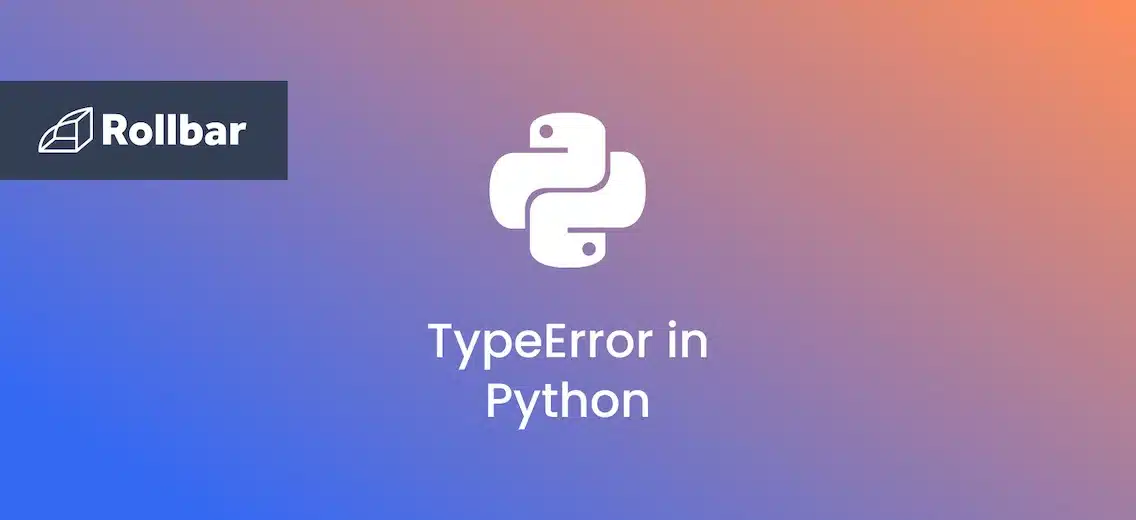
How to Handle TypeError: Cannot Unpack Non-iterable Nonetype Objects in Python
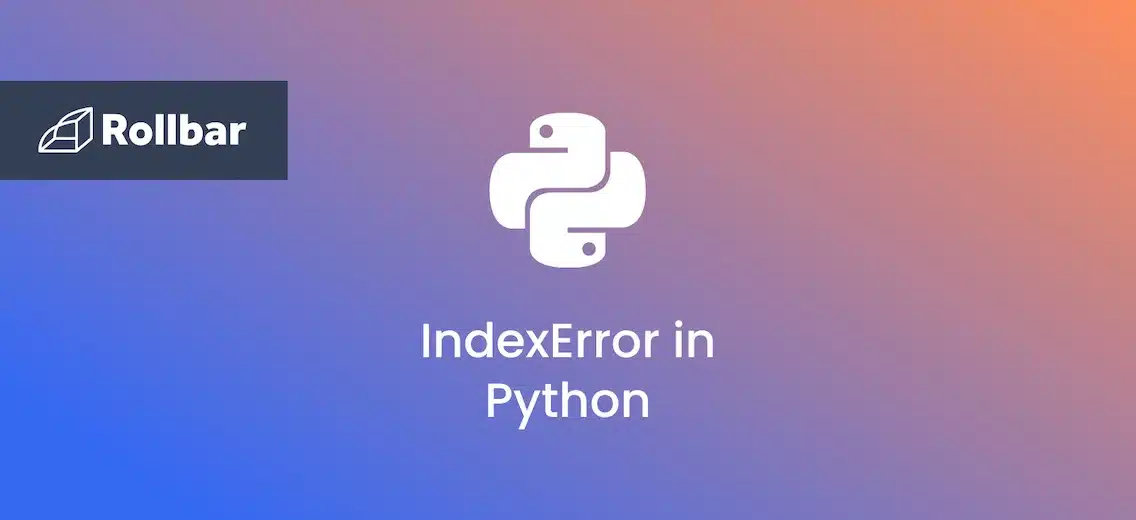
How to Fix IndexError: string index out of range in Python
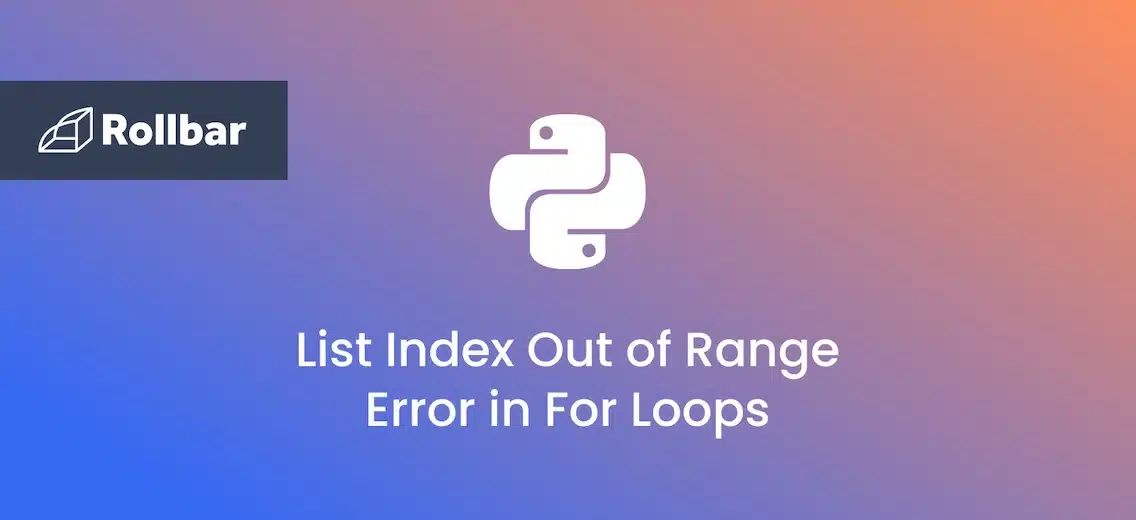
How to Fix Python’s “List Index Out of Range” Error in For Loops
"Rollbar allows us to go from alerting to impact analysis and resolution in a matter of minutes. Without it we would be flying blind."
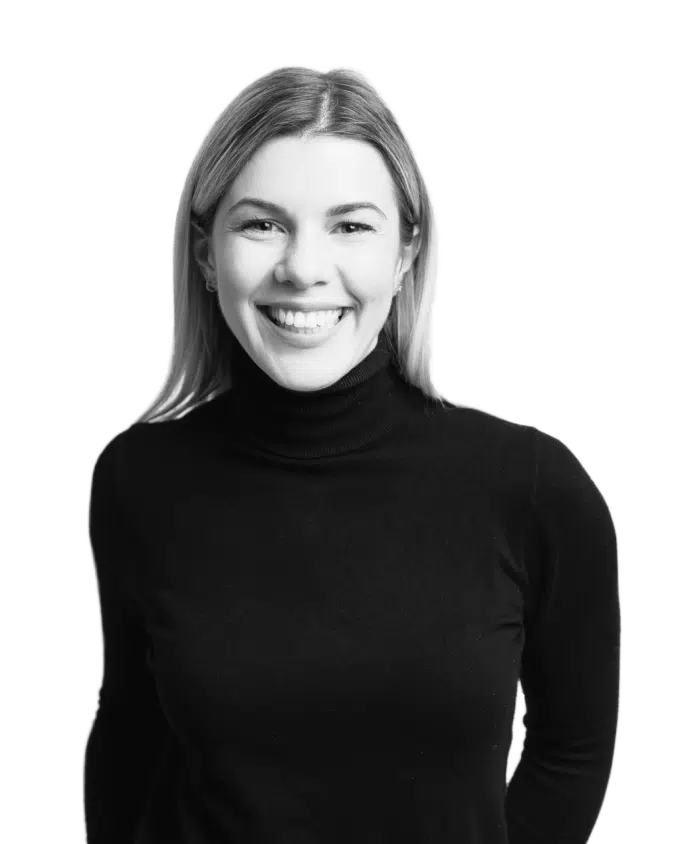
Start continuously improving your code today.
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
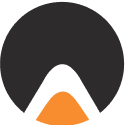
- Resource Center
- Bachelor’s Degree
- Master’s Degree
Python indexerror: list assignment index out of range Solution
An IndexError is nothing to worry about. It’s an error that is raised when you try to access an index that is outside of the size of a list. How do you solve this issue? Where can it be raised?
In this article, we’re going to answer those questions. We will discuss what IndexErrors are and how you can solve the “list assignment index out of range” error. We’ll walk through an example to help you see exactly what causes this error.
Find your bootcamp match
Without further ado, let’s begin!
The Problem: indexerror: list assignment index out of range
When you receive an error message, the first thing you should do is read it. An error message can tell you a lot about the nature of an error.
Our error message is: indexerror: list assignment index out of range.
IndexError tells us that there is a problem with how we are accessing an index . An index is a value inside an iterable object, such as a list or a string.
The message “list assignment index out of range” tells us that we are trying to assign an item to an index that does not exist.
In order to use indexing on a list, you need to initialize the list. If you try to assign an item into a list at an index position that does not exist, this error will be raised.
An Example Scenario
The list assignment error is commonly raised in for and while loops .
We’re going to write a program that adds all the cakes containing the word “Strawberry” into a new array. Let’s start by declaring two variables:
The first variable stores our list of cakes. The second variable is an empty list that will store all of the strawberry cakes. Next, we’re going to write a loop that checks if each value in “cakes” contains the word “Strawberry”.
If a value contains “Strawberry”, it should be added to our new array. Otherwise, nothing will happen. Once our for loop has executed, the “strawberry” array should be printed to the console. Let’s run our code and see what happens:
As we expected, an error has been raised. Now we get to solve it!
The Solution
Our error message tells us the line of code at which our program fails:
The problem with this code is that we are trying to assign a value inside our “strawberry” list to a position that does not exist.
When we create our strawberry array, it has no values. This means that it has no index numbers. The following values do not exist:
We are trying to assign values to these positions in our for loop. Because these positions contain no values, an error is returned.
We can solve this problem in two ways.
Solution with append()
First, we can add an item to the “strawberry” array using append() :
The append() method adds an item to an array and creates an index position for that item. Let’s run our code: [‘Strawberry Tart’, ‘Strawberry Cheesecake’].
Our code works!
Solution with Initializing an Array
Alternatively, we can initialize our array with some values when we declare it. This will create the index positions at which we can store values inside our “strawberry” array.
To initialize an array, you can use this code:
This will create an array with 10 empty values. Our code now looks like this:
Let’s try to run our code:
Our code successfully returns an array with all the strawberry cakes.
This method is best to use when you know exactly how many values you’re going to store in an array.

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our above code is somewhat inefficient because we have initialized “strawberry” with 10 empty values. There are only a total of three cakes in our “cakes” array that could possibly contain “Strawberry”. In most cases, using the append() method is both more elegant and more efficient.
IndexErrors are raised when you try to use an item at an index value that does not exist. The “indexerror: list assignment index out of range” is raised when you try to assign an item to an index position that does not exist.
To solve this error, you can use append() to add an item to a list. You can also initialize a list before you start inserting values to avoid this error.
Now you’re ready to start solving the list assignment error like a professional Python developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
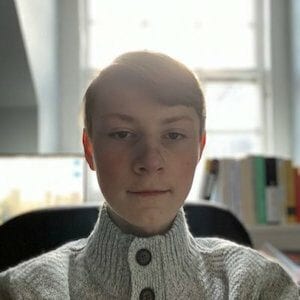
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
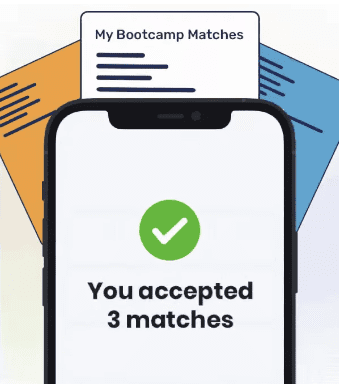
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Indexerror: list assignment index out of range Solution
In python, lists are mutable as the elements of a list can be modified. But if you try to modify a value whose index is greater than or equal to the length of the list then you will encounter an Indexerror: list assignment index out of range.
Python Indexerror: list assignment index out of range Example
If ‘fruits’ is a list, fruits=[‘Apple’,’ Banana’,’ Guava’]and you try to modify fruits[5] then you will get an index error since the length of fruits list=3 which is less than index asked to modify for which is 5.
So, as you can see in the above example, we get an error when we try to modify an index that is not present in the list of fruits.
Method 1: Using insert() function
The insert(index, element) function takes two arguments, index and element, and adds a new element at the specified index.
Let’s see how you can add Mango to the list of fruits on index 1.
It is necessary to specify the index in the insert(index, element) function, otherwise, you will an error that the insert(index, element) function needed two arguments.

Method 2: Using append()
The append(element) function takes one argument element and adds a new element at the end of the list.
Let’s see how you can add Mango to the end of the list using the append(element) function.
Python IndexError FAQ
Q: what is an indexerror in python.
A: An IndexError is a common error that occurs when you try to access an element in a list, tuple, or other sequence using an index that is out of range. It means that the index you provided is either negative or greater than or equal to the length of the sequence.
Q: How can I fix an IndexError in Python?
A: To fix an IndexError, you can take the following steps:
- Check the index value: Make sure the index you’re using is within the valid range for the sequence. Remember that indexing starts from 0, so the first element is at index 0, the second at index 1, and so on.
- Verify the sequence length: Ensure that the sequence you’re working with has enough elements. If the sequence is empty, trying to access any index will result in an IndexError.
- Review loop conditions: If the IndexError occurs within a loop, check the loop conditions to ensure they are correctly set. Make sure the loop is not running more times than expected or trying to access an element beyond the sequence’s length.
- Use try-except: Wrap the code block that might raise an IndexError within a try-except block. This allows you to catch the exception and handle it gracefully, preventing your program from crashing.
Please Login to comment...
Similar reads.
- Python How-to-fix
- python-list
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
How to Fix Python IndexError: list assignment index out of range
- Python How-To's
- How to Fix Python IndexError: list …
Python IndexError: list assignment index out of range
Fix the indexerror: list assignment index out of range in python, fix indexerror: list assignment index out of range using append() function, fix indexerror: list assignment index out of range using insert() function.
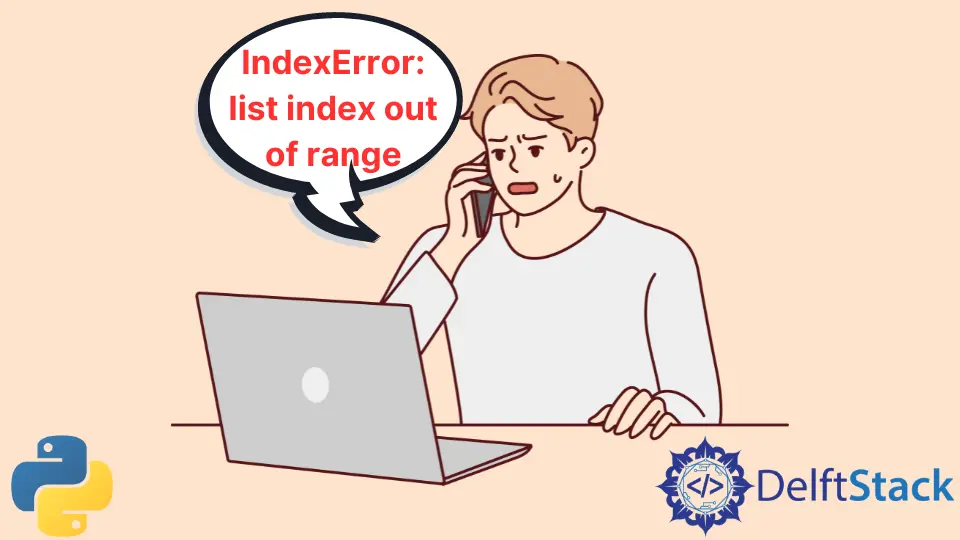
In Python, the IndexError: list assignment index out of range is raised when you try to access an index of a list that doesn’t even exist. An index is the location of values inside an iterable such as a string, list, or array.
In this article, we’ll learn how to fix the Index Error list assignment index out-of-range error in Python.
Let’s see an example of the error to understand and solve it.
Code Example:
The reason behind the IndexError: list assignment index out of range in the above code is that we’re trying to access the value at the index 3 , which is not available in list j .
To fix this error, we need to adjust the indexing of iterables in this case list. Let’s say we have two lists, and you want to replace list a with list b .
You cannot assign values to list b because the length of it is 0 , and you are trying to add values at kth index b[k] = I , so it is raising the Index Error. You can fix it using the append() and insert() .
The append() function adds items (values, strings, objects, etc.) at the end of the list. It is helpful because you don’t have to manage the index headache.
The insert() function can directly insert values to the k'th position in the list. It takes two arguments, insert(index, value) .
In addition to the above two solutions, if you want to treat Python lists like normal arrays in other languages, you can pre-defined your list size with None values.
Once you have defined your list with dummy values None , you can use it accordingly.
There could be a few more manual techniques and logic to handle the IndexError: list assignment index out of range in Python. This article overviews the two common list functions that help us handle the Index Error in Python while replacing two lists.
We have also discussed an alternative solution to pre-defined the list and treat it as an array similar to the arrays of other programming languages.
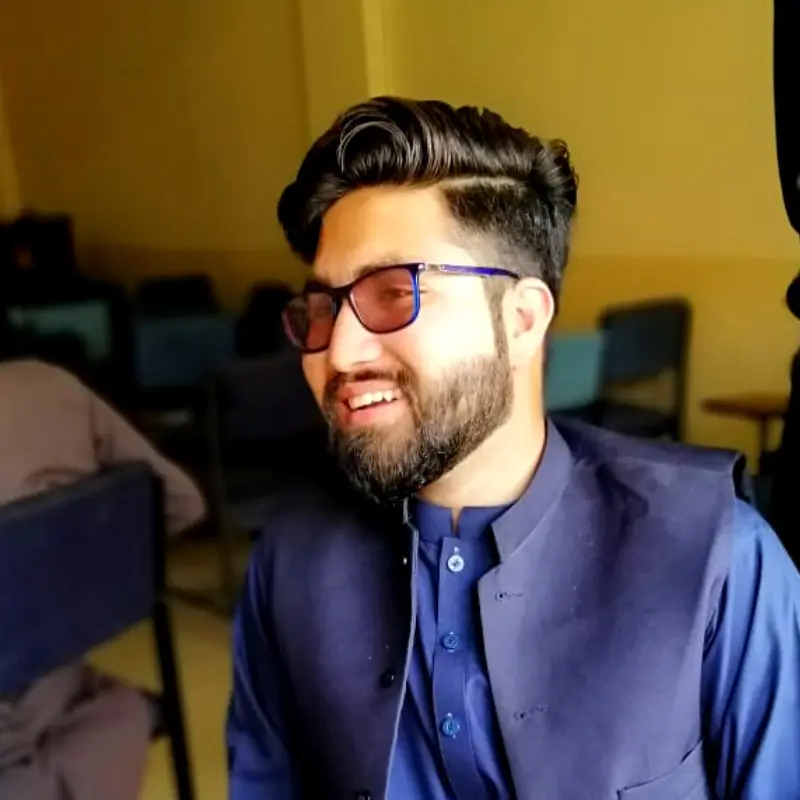
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
List Index Out of Range – Python Error Message Solved
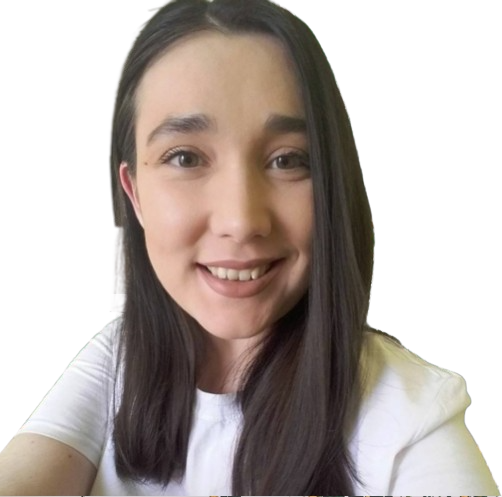
In this article you'll see a few of the reasons that cause the list index out of range Python error.
Besides knowing why this error occurs in the first place, you'll also learn some ways to avoid it.
Let's get started!
How to Create a List in Python
To create a list object in Python, you need to:
- Give the list a name,
- Use the assignment operator, = ,
- and include 0 or more list items inside square brackets, [] . Each list item needs to be separated by a comma.
For example, to create a list of names you would do the following:
The code above created a list called names that has four values: Kelly, Nelly, Jimmy, Lenny .
How to Check the Length of a List in Python
To check the length of a list in Python, use Python's build-in len() method.
len() will return an integer, which will be the number of items stored in the list.
There are four items stored in the list, therefore the length of the list will be four.
How to Access Individual List Items in Python
Each item in a list has its own index number .
Indexing in Python, and most modern programming languages, starts at 0.
This means that the first item in a list has an index of 0, the second item has an index of 1, and so on.
You can use the index number to access the individual item.
To access an item in a list using its index number, first write the name of the list. Then, inside square brackets, include the intiger that corresponds with the item's index number.
Taking the example from earlier, this is how you would access each item inside the list using its index number:
You can also use negative indexing to access items inside lists in Python.
To access the last item, you use the index value of -1. To acces the second to last item, you use the index value of -2.
Here is how you would access each item inside a list using negative indexing:
Why does the Indexerror: list index out of range error occur in Python?
Using an index number that is out of the range of the list.
You'll get the Indexerror: list index out of range error when you try and access an item using a value that is out of the index range of the list and does not exist.
This is quite common when you try to access the last item of a list, or the first one if you're using negative indexing.
Let's go back to the list we've used so far.
Say I want to access the last item, "Lenny", and try to do so by using the following code:
Generally, the index range of a list is 0 to n-1 , with n being the total number of values in the list.
With the total values of the list above being 4 , the index range is 0 to 3 .
Now, let's try to access an item using negative indexing.
Say I want to access the first item in the list, "Kelly", by using negative indexing.
When using negative indexing, the index range of a list is -1 to -n , where -n the total number of items contained in the list.
With the total number of items in the list being 4 , the index range is -1 to -4 .
Using the wrong value in the range() function in a Python for loop
You'll get the Indexerror: list index out of range error when iterating through a list and trying to access an item that doesn't exist.
One common instance where this can occur is when you use the wrong integer in Python's range() function.
The range() function typically takes in one integer number, which indicates where the counting will stop.
For example, range(5) indicates that the counting will start from 0 and end at 4 .
So, by default, the counting starts at position 0 , is incremented by 1 each time, and the number is up to – but not including – the position where the counting will stop.
Let's take the following example:
Here, the list names has four values.
I wanted to loop through the list and print out each value.
When I used range(5) I was telling the Python interpreter to print the values that are at the positions 0 to 4 .
However, there is no item in position 4.
You can see this by first printing out the number of the position and then the value at that position.
You see that at position 0 is "Kelly", at position 1 is "Nelly", at position 2 is "Jimmy" and at position 3 is "Lenny".
When it comes to position four, which was specified with range(5) which indicates positions of 0 to 4 , there is nothing to print out and therefore the interpreter throws an error.
One way to fix this is to lower the integer in range() :
Another way to fix this when using a for loop is to pass the length of the list as an argument to the range() function. You do this by using the len() built-in Python function, as shown in an earlier section:
When passing len() as an argument to range() , make sure that you don't make the following mistake:
After running the code, you'll again get an IndexError: list index out of range error:
Hopefully this article gave you some insight into why the IndexError: list index out of range error occurs and some ways you can avoid it.
If you want to learn more about Python, check out freeCodeCamp's Python Certification . You'll start learning in an interacitve and beginner-friendly way. You'll also build five projects at the end to put into practice and help reinforce what you learned.
Thanks for reading and happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
How to solve IndexError list index out of range
IndexError list index out of range is one of the most popular errors in python. In this tutorial, I'll show you the reasons for this issue and how to solve it.
Let's get started.
Reasons for IndexError list index out of range
As you know, a list starts at index 0 . And, my_list has 3 items that mean:
But in the above example, I want to get index 5 which does not exist in my_list . So, if you already know the number of items, you need just set the correct index.
In this example, we've attempted to get index 0 from the empty list. And therefore, we've got the error.
Solutions for IndexError list index out of range error
To solve IndexError list index out of range , you need to follow these solutions:
Solution 1: Check if the list is empty
For more details about this method, visit Python Check if Value Exists in List .
Solution 2: Compare between the number of items in the list and the index
"If the number of list items is bigger than the index, that means the index exists. Otherwise, the index doesn't exist."
As you can see, we've used the len() function to get the number of items in my_list . Then, we've compared my_list and my_index .
Solution 3: Use try and except statement
Related tutorials:, recent tutorials:.

Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Using Update Cursor gives IndexError: list assignment index out of range?
I am trying to use an update cursor and receiving an error. I'm not very familiar with using cursors, so please bear with me. I am trying to write a 1 or 0 to a field, based on a condition. The error I am receiving is:
IndexError: list assignment index out of range.
I have googled around and have seen solutions, but I'm still not understanding why its throwing an error.
- 3 Python Array Use 101: indexes start at zero, and you only provide four field names, so row[4] is out of range. – Vince Commented Dec 1, 2018 at 13:05
Your update cursor has four values in its row: ["Base", "Top",rastername, fieldname] corresponding to row[0],row[1],row[2],row[3] respectively. row[4] is out of range because it is the fifth of four items.

- Thanks for the help.I'm not sure how I missed that, as I accounted for row[0].. I adjusted the script and it ran as expected. Thanks again for looking it over! – phead Commented Dec 2, 2018 at 4:39
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged arcpy cursor update indexerror boolean or ask your own question .
- Featured on Meta
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- We spent a sprint addressing your requests — here’s how it went
Hot Network Questions
- How can I get the value of the f(1/2) is 11/4 not 2.75 when I use \pgfmathprintnumber\pgfmathresult?
- Is this a Hadamard matrix?
- Why do jet aircraft need chocks when they have parking brakes?
- Decomposing the homology of a finite-index subgroup into isotypic components
- Animate multiple material transitions smoothly
- How is 11:22 four minutes slow if it's actually 11:29?
- Is ElGamal homomorphic encryption using additive groups works only for Discrete Log ElGamal? What about EC ElGamal?
- What caused the builder to change plans midstream on this 1905 library in New England?
- How are GameManagers created in Unity?
- Are there countries where only voters affected by a given policy get to vote on it?
- Real-life problems involving solving triangles
- Intelligence vs Wisdom in D&D
- Using "Delight" Without a Preposition
- Ants on a Stick Probability Question
- Does the damage from Thunderwave occur before or after the target is moved
- What is the difference between 居る and 要る?
- Why does King Aegon speak to his dragon in the Common Tongue (English)?
- Big zeros in block diagonal matrix
- The rear wheel from my new Road Bike vibrates strongly
- What scientifically plausible apocalypse scenario, if any, meets my criteria?
- Is it a security issue to expose PII on any publically accessible URL?
- Why seperating a sphere gives different shades?
- Is taping behind tile necessary when drywall seams are not easily accessible?
- Is it possible with modern-day technology to expand an already built bunker further below without the risk of collapsing the entire bunker?
Python IndexError: List Index Out of Range [Easy Fix]
Key Points:
- To solve the “IndexError: list index out of range” , avoid do not access a non-existing list index. For example, my_list[5] causes an error for a list with three elements.
- If you access list elements in a loop, keep in mind that Python uses zero-based indexing : For a list with n elements, the first element has index 0 and the last index n-1 .
- A common cause of the error is trying to access indices 1, 2, ..., n instead of using the correct indices 0,1, ..., (n-1) .
![indexerror list assignment index out of range stack overflow Python IndexError: List Index Out of Range [Easy Fix]](https://blog.finxter.com/wp-content/plugins/wp-youtube-lyte/lyteCache.php?origThumbUrl=https%3A%2F%2Fi.ytimg.com%2Fvi%2Ffe3nwCR6r2o%2F0.jpg)
If you’re like me, you try things first in your code and fix the bugs as they come.
One frequent bug in Python is the IndexError: list index out of range . So, what does this error message mean?
The error “ list index out of range ” arises if you access invalid indices in your Python list. For example, if you try to access the list element with index 100 but your lists consist only of three elements, Python will throw an IndexError telling you that the list index is out of range.
Minimal Example
Here’s a screenshot of this happening on my Windows machine:
Let’s have a look at an example where this error arises:
The element with index 3 doesn’t exist in the list with three elements. Why is that?
The following graphic shows that the maximal index in your list is 2. The call lst[2] would retrieve the third list element 'Carl' .
- lst[0] --> Alice
- lst[1] --> Bob
- lst[2] --> Carl
- lst[3] --> ??? Error ???
Did you try to access the third element with index 3?
It’s a common mistake: The index of the third element is 2 because the index of the first list element is 0 .
How to Fix the IndexError in a For Loop? [General Strategy]
So, how can you fix the code? Python tells you in which line and on which list the error occurs.
To pin down the exact problem, check the value of the index just before the error occurs.
To achieve this, you can print the index that causes the error before you use it on the list. This way, you’ll have your wrong index in the shell right before the error message.
Here’s an example of wrong code that will cause the error to appear:
The error message tells you that the error appears in line 5.
So, let’s insert a print statement before that line:
The result of this code snippet is still an error.
But there’s more:
You can now see all indices used to retrieve an element.
The final one is the index i=4 which points to the fifth element in the list (remember zero-based indexing : Python starts indexing at index 0! ).
But the list has only four elements, so you need to reduce the number of indices you’re iterating over.
The correct code is, therefore:
Note that this is a minimal example and it doesn’t make a lot of sense. But the general debugging strategy remains even for advanced code projects:
- Figure out the faulty index just before the error is thrown.
- Eliminate the source of the faulty index.
IndexError When Modifying a List as You Iterate Over It
The IndexError also frequently occurs if you iterate over a list but you remove elemen t s as you iterate over the list:
This code snippet is from a StackOverflow question.
The source is simply that the list.pop() method removes the element with value 0 .

All subsequent elements now have a smaller index.
But you iterate over all indices up to len(l)-1 = 6-1 = 5 and the index 5 does not exist in the list after removing elements in a previous iteration.
You can fix this with a simple list comprehension statement that accomplishes the same thing:
Only non-zero elements are included in the list.
String IndexError: List Index Out of Range
The error can occur when accessing strings as well.
Check out this example:
Genius! Here’s what it looks like on my machine:
To fix the error for strings, make sure that the index falls between the range 0 ... len(s)-1 (included):
Tuple IndexError: List Index Out of Range
In fact, the IndexError can occur for all ordered collections where you can use indexing to retrieve certain elements.
Thus, it also occurs when accessing tuple indices that do not exist:
No. You didn’t? 😅
Again, start counting with index 0 to get rid of this:
Note : The index of the last element in any sequence is len(sequence)-1 .
Programmer Humor
Where to go from here.
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
🚀 If your answer is YES! , consider becoming a Python freelance developer ! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
Join the free webinar now!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
IndexError: list index out of range
I'm implementing a sequence-2-sequence model with RNN-VAE architecture, and I use an attention mechanism. I have problem in the decoder part. I'm struggling with this error: IndexError: list index out of range When I run this code:
The error is raised at the last line, and the traceback given:
in AttentionLayer class , build function id defined by:
If someone can help me I'll be so thankful, I cannot understant where the problem is, and how to resolve it.
Thanks in advance
- autoencoder
- sequence-to-sequence

- $\begingroup$ In my case, input tensors didn't have a shape. That means, shape=() . I reshaped via: tf.reshape(x["time"], (1,)) $\endgroup$ – iedmrc Commented Jul 23, 2020 at 13:23
Maybe you are not using the attention.py you think you are using. The error states that the problematic line in Attention build is:
shape=tf.TensorShape((input_shape[0][3], input_shape[0][3])),
but the code of attention.py you shared with us does not seem to contain such line. It is possible you also just forgot to save your changes to the new attention.py file.
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python keras tensorflow autoencoder sequence-to-sequence or ask your own question .
- Featured on Meta
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- We spent a sprint addressing your requests — here’s how it went
Hot Network Questions
- Generate filesystem usage report using Awk
- How can I break an alignedat/gathered environment between pages?
- If someone clearly believes that he has witnessed something extraordinary very clearly, why is it more reasonable to believe that they hallucinated?
- Has the Journal of Fluid Mechanics really published more than 800 volumes?
- Should I apologise to a professor after a gift authorship attempt, which they refused?
- I want to pick my flight route. I can either get 2 round trip tickets (SRC<->MID, MID<->DST) or 3rd party booking (SRC<->DST). Which is more reliable?
- MOSFET Datasheet Confusion
- Infinity is not a number
- 「今日伺うお店」translated as "go-to restuarant"
- Mathematical Induction over two numbers
- Why do jet aircraft need chocks when they have parking brakes?
- What type of airfoil should be used for horizontal tailplanes?
- Canon PowerShot A80 keeps shutting down and asking to change the battery pack. What should I do?
- Does the cosmological constant entail a mass for the graviton?
- What is the difference between 居る and 要る?
- How to reference a picture with two images?
- Equivalence of first/second choice with naive probability - I don't buy it
- Time integration of first-order ODE with higher-order information
- Interesting structure: ".....ever go to do... ! --- "If you ever go to sell this place, let me know."
- Can Black Lotus alone enable the Turn 1 Channel-Fireball combo?
- Installation error (MySQL)
- Schematic component
- Submitting 2 manuscripts explaining the same scientific fact but by two different methods
- Can I set QGIS to summarize icons above a certain scale and display only the number of icons in an area?
Get the Reddit app
Subreddit for posting questions and asking for general advice about your python code.
IndexError: list index out of range Error??
I have 30 minutes to submit this assignment and I am fucking crying. I don't know why this is happening and I've got another program I have to work on. It keeps saying that the first if statement is out of range of my list but it literally isn't. Here's my code:
Edit: Fixed that part, but now the last for loop isn't wroking, nothing is being printed onto the document.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Sorting with AlgoBuild. Why do I get an index is out of range error?
I am using AlgoBuild to make a program that sorts numbers.
This is my attempt:

AlgoBuild translates this chart to the following pseudo code:
When running it, I got the following error on the statement IF V[k] > V[j] :
IF ERROR: Array index out of range IN V[k] > V[j]
I didn't expect this error.
I can't understand why it is out of range if I made the assignment k is equal to j . What is my mistake?

- 3 "if I don't write some more lines it won't make me post the question" - never just write waffle in order to satisfy a quality filter. Instead, think about what would actually provide more useful information... such as the size of the array, and the precise error message you're seeing. (I'm not familiar with matlab, but does the error tell you which of the steps is failing, for example?) I'd be astonished if there was really nothing else you could add to the question that would provide useful information. – Jon Skeet Commented 10 hours ago
- I'm kinda new to this and I was in a hurry, next time I will add more detail. Thanks for the answer btw – giuamato50 Commented 3 hours ago
Your second and third loop allow their loop variables to become equal to D , but you have not defined V[D] , so that will be out of range.
Array indices run from 0 onwards, so if you have D number of values in your array V , the first one is V[0] and the last one is V[D-1] , not V[D] .
So change <= D in your middle loops to < D , like this:
With those two fixes it should work.
- 1 I didn’t even realize that flow chart was input to some software. Thanks for correcting me. – Cris Luengo Commented 9 hours ago
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged arrays algorithm sorting indexing or ask your own question .
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- How to reference a picture with two images?
- Is taping behind tile necessary when drywall seams are not easily accessible?
- Equivalence of first/second choice with naive probability - I don't buy it
- Spotlight SAR image formation
- Submitting 2 manuscripts explaining the same scientific fact but by two different methods
- Senior citizen ladies solve crimes
- Big zeros in block diagonal matrix
- How are Boggarts created?
- DHCP assigned addresses following devices/users and routing
- Integrated Portal to seek public transport connections in the UK, preferably with prices
- How can one count how many pixels a GIF image has via command line?
- Has the Journal of Fluid Mechanics really published more than 800 volumes?
- Interesting structure: ".....ever go to do... ! --- "If you ever go to sell this place, let me know."
- Animate multiple material transitions smoothly
- Can Black Lotus alone enable the Turn 1 Channel-Fireball combo?
- Generate filesystem usage report using Awk
- What does this mean in RISC-V opcode table
- How does \if:w work?
- MOSFET Datasheet Confusion
- If someone clearly believes that he has witnessed something extraordinary very clearly, why is it more reasonable to believe that they hallucinated?
- The rear wheel from my new Road Bike vibrates strongly
- Understanding top memory bar
- Can computer components be damaged if they stay off for a long time?
- Is "sinnate" a word? What does it mean?

IMAGES
VIDEO
COMMENTS
Your list starts out empty because of this: a = [] then you add 2 elements to it, with this code: a.append(3) a.append(7) this makes the size of the list just big enough to hold 2 elements, the two you added, which has an index of 0 and 1 (python lists are 0-based). In your code, further down, you then specify the contents of element j which ...
2. Your problem is that del l[min(l)] is trying to reference the list item at index min(l). Let's assume that your list has 3 items in it: l = [22,31,17] Trying to delete the item at the index min(l) is referencing the index 17, which doesn't exist. Only indices 0, 1, and 2 exist. I think what you want to do is remove the smallest item from ...
Ask questions, find answers and collaborate at work with Stack Overflow for Teams. Explore Teams Create a free Team
The list.extend method returns None as it mutates the original list. # (CSV) IndexError: list index out of range in Python. The Python CSV "IndexError: list index out of range" occurs when we try to access a list at an index out of range, e.g. an empty row in a CSV file.
As you can see, now the 'bird' value is added to the list successfully. Adding the value using the append() method increases the list index range, which enables you to modify the item at the new index using the list assignment syntax.. To summarize, use the append() method when you're adding a new element and increasing the size of the list, and use the list assignment index when you ...
Python Indexerror: list assignment index out of range Solution In python, lists are mutable as the elements of a list can be modified. But if you try to modify a value whose index is greater than or equal to the length of the list then you will encounter an Indexerror: list assignment index out of range.
How to use the insert() method. Use the insert() method to insert elements at a specific position instead of direct assignment to avoid out-of-range assignments. Example: my_list = [ 10, 20, 30 ] my_list.insert( 3, 987) #Inserting element at index 3 print (my_list) Output: [10, 20, 30, 987] Now one big advantage of using insert() is even if you ...
The append() method adds an item to an array and creates an index position for that item. Let's run our code: ['Strawberry Tart', 'Strawberry Cheesecake']. Our code works! Solution with Initializing an Array. Alternatively, we can initialize our array with some values when we declare it. This will create the index positions at which we can store values inside our "strawberry" array.
Python Indexerror: list assignment index out of range Solution. Method 1: Using insert () function. The insert (index, element) function takes two arguments, index and element, and adds a new element at the specified index. Let's see how you can add Mango to the list of fruits on index 1. Python3. fruits = ['Apple', 'Banana', 'Guava']
The following code raises an IndexError, can anyone explain why the logic of this code does not work? midterms = [90, 80, 89, 87, 97, 100] for mark in midterms: newMark = mark + 2 midterms...
The reason behind the IndexError: list assignment index out of range in the above code is that we're trying to access the value at the index 3, which is not available in list j. Fix the IndexError: list assignment index out of range in Python. To fix this error, we need to adjust the indexing of iterables in this case list.
freeCodeCamp is a donor-supported tax-exempt 501(c)(3) charity organization (United States Federal Tax Identification Number: 82-0779546) Our mission: to help people learn to code for free.
Solution 2: Compare between the number of items in the list and the index "If the number of list items is bigger than the index, that means the index exists.
Stack Exchange Network. Stack Exchange network consists of 183 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers. Visit Stack Exchange
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students. To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He's the author of the best-selling programming books Python One-Liners (NoStarch ...
Stack Exchange network consists of 183 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers. Visit Stack Exchange. ... IndexError: list index out of range ...
1. When asking about code that produces an Exception, always include the complete Traceback in the question. Copy the Traceback and paste it in the question, then format it as code (select it and type ctrl-k) - wwii. Jul 3, 2019 at 2:03. When you're indexing temp with ord(str1[i]) - ord('a'), you probably go out of the bounds of the list.
I have 30 minutes to submit this assignment and I am fucking crying. I don't know why this is happening and I've got another program I have to work on. It keeps saying that the first if statement is out of range of my list but it literally isn't. Here's my code:
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question. Provide details and share your research! But avoid … Asking for help, clarification, or responding to other answers. Making statements based on opinion; back them up with references or personal experience. To learn more, see our tips on writing great ...