- Engineering & Technology
- Computer Science
- Data Structures

CSEC IT - A Guide to Problem Solving and Program Design
Related documents.
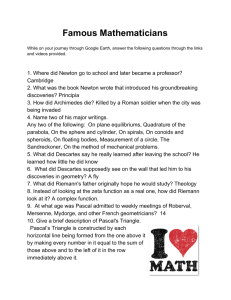
Add this document to collection(s)
You can add this document to your study collection(s)
Add this document to saved
You can add this document to your saved list
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Problem Solving & Program Design in C by Jeri R. Hanly, Elliot B. Koffman
nikhilnayak98/CSE2041
Folders and files.
Name | Name | |||
---|---|---|---|---|
154 Commits | ||||
Repository files navigation
Programming Practice - 1 C Codes
Problem Solving and Program Design in C
Contributors 2
- DSA Tutorial
- Data Structures
- Linked List
- Dynamic Programming
- Binary Tree
- Binary Search Tree
- Divide & Conquer
- Mathematical
- Backtracking
- Branch and Bound
- Pattern Searching
Data Structures and Algorithms (DSA) in C
Data Structures and Algorithms (DSA) are one of the most important concepts of programming. They form the foundation of problem solving in computer science providing different and efficient solutions to the given problem that suits the requirement. This tutorial guide will help you understand the basics of various data structures and algorithms using C programming language.
Why Learn DSA Using C?
C language is a perfect choice for learning data structures and algorithms due to the following reasons:
- Foundational Language: C is a low-level language with only a few of inbuilt functionalities. It makes the programmer to implement almost everything by himself which helps in understanding how data structures work at a fundamental level.
- Versatility: Knowledge of DSA in C can be transferred to other languages, as many modern languages are built on C-like syntax.
- Interview Preparation: Many technical interviews and competitive programming problems are best solved using C due to its speed and efficiency.
Data Structures and Algorithms Tutorial using C
This C tutorial provides a step-by-step guide to the key concepts of DSA, from basic to complex data structures along with essential algorithms like sorting, searching, graph traversal, etc. Whether you’re a beginner or experienced looking to expand your knowledge, this tutorial has something for you.
Data Structures and Algorithms Complete Course using C – We’ve got a course for you – DSA Self-Paced which can help you to upskill yourself, be able to solve competitive programming questions, and get you placement-ready. No matter whether you’ve complete knowledge of DSA or not, no matter whether you’re a beginner at DSA, these factors can never be a hurdle if you’re someone who really wants to upskill and build a career in DSA.
An array is a fixed size data structure that stores a collection of elements in contiguous memory locations. Each element is of same type and can be accessed by its index.
Based on the number of dimensions, there are two types of arrays in C:
- 1D Array in C
- 2D Array in C
- 3D Array in C
Linked Lists
A linked list is a linear data structure where each element (called a node) contains a data field and a pointer to the next node in the list. It does not store the data in contagious memory, and we can only access the elements sequentially.
Types of linked lists and their implementation in C:
- Singly Linked List in C
- Doubly Linked List in C
- Circular Linked List in C
A stack is a linear data structure that follows the Last-In First-Out (LIFO) principle of element insertion and deletion. It means that insertion and deletion can only happen at one end.
Stack can be implemented over different data structure. The most popular implementations of stack in C are:
- Stack Implementation Using Array in C
- Stack Implementation Using Linked List in C
A queue is a linear data structure like stack, but it follows the First-In, First-Out (FIFO) principle of element insertion and deletion. Elements are added at one end and removed from the other end.
There are 4 types of queues:
- Queue Implementation Using Arrays in C
- Queue Implementation Using Linked Lists in C
- Circular Queue in C
- Double-Ended Queue (Deque) in C
- Priority Queue in C (need the knowledge of heap)
Searching Algorithms
Searching algorithms are the methods used to find a specific element efficiently within the given data structure, such as arrays, lists, etc.
There are mainly two types of searching algorithms:
- Sentinel Linear Search in C
- Binary Search in C
Sorting Algorithms
Sorting algorithms are the methods used to arrange elements in a particular order, typically in ascending or descending order.
Following are the popular sorting algorithms:
- Selection Sort in C
- Bubble Sort in C
- Insertion Sort in C
- In-place Merge Sort in C
- Iterative Quick Sort in C
- Counting Sort in C
- Radix Sort in C
- Heap Sort in C (need the knowledge of Heaps)
A tree is a hierarchical data structure consisting of nodes, where each node has a data field and pointer to its child nodes. It can have multiple children and based on the number of children, trees are classified.
Unlike linear data structure, trees can be traverse in multiple ways:
- Level Order Traversal in C
- Pre-Order Traversal in C
- In-Order Traversal in C
- Post-Order Traversal in C
Trees are also classified on the basis of the type of structure they form:
- Binary Tree in C
- Binary Search Tree (BST) in C
- AVL Tree in C
- Red-Black Tree in C
- B-Tree in C
- B+ Tree in C
- Segment Tree in C
- Trie (Prefix Tree) in C
- K-D Tree in C
A heap is a specialized tree-based data structure that satisfies the heap property: in a max-heap, every parent node is greater than or equal to its child nodes, and in a min-heap, every parent node is less than or equal to its child nodes.
- Min Heap in C
- Max Heap in C
- Binomial Heap in C
- Fibonacci Heap in C
- Priority Queue in C
- Heap Sort in C

Greedy Algorithms
Greedy algorithmic approach instructs to make the local optimal decision at every step i.e. it will choose the current best option without of considering its effects in the future.
Following are some standard Greedy algorithms:
- Fractional Knapsack Problem in C
- Activity Selection Problem in C
- Job Sequencing Problem in C
- Huffman Coding Problem in C
Divide and Conquer Algorithms
In divide and conquer approach, the problem is divided into smaller subproblems, solved and then recombined to give the complete solution.
Following are some standard Divide and Conquer algorithms:
- Tower of Hanoi in C
- Convex Hull in C
- Karatsuba Algorithm for Fast Multiplication in C
- Strassen’s Algorithm for Matrix Multiplication in C
Dynamic Programming Algorithms
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems and storing the solutions to avoid redundant calculations.
Following are some standard Dynamic Programming algorithms:
- Rod Cutting Problem in C
- Coin Change Problem in C
- Subset Sum Problem in C
- 0-1 Knapsack in C
- Edit Distance in C
- Longest Palindromic Subsequence in C
- Minimum Cost Path in C
- Maximum Subarray Sum in C
- Partition Equal Subset Sum in C
- Maximum Product Subarray in C
A graph is a non-linear collection of nodes (called vertices) connected by edges. They are generally represented as Adjacency List and Adjacency Matrix.
Implementation of Graphs in C
- Graph using Adjacency Matrix in C
- Graph Using Adjacency List in C
Classification of Graphs
- Directed Graph in C
- Undirected Graph in C
- Weighted Graph in C
- Unweighted Graph in C
Graph Algorithms
Graph algorithms are a set of methods used to solve problems related to graphs:
- Graph Cycle Detection in C
- Prim’s Algorithm in C
- Kruskal’s Algorithm in C
- Dijkstra’s Algorithm in C
- Bellman-Ford Algorithm in C
- Floyd-Warshall Algorithm in C
- Johnson Algorithm in C
- Kosaraju’s Algorithm in C
- Tarjan’s Algorithm in C
- Kahn’s Algorithm in C
String Algorithms
String algorithms are techniques designed to process and manipulate strings for tasks such as pattern matching, substring searching, and text processing.
- Rabin Karp in C
- Knuth-Morris-Pratt in C
- Boyer-Moore in C
- Longest Common Subsequence in C
- Longest Increasing Subsequence in C
Bit Manipulation
Bit manipulation involves performing operations directly on binary digits. These operations are used to optimize performance and memory usage.
There are 5 major bit operations:
- Extract bits in C
- Setting bits in C
- Clearing bits in C
- Toggling bits in C
- Counting Set bits in C
Backtracking Algorithms
Backtracking algorithms are a systematic way of solving constraint satisfaction problems by trying out different possibilities and eliminating those that fail to satisfy the problem’s conditions.
Following are some standard backtracking algorithms:
- Knight’s Tour Problem in C
- Rat in a Maze in C
- N-Queen Problem in C
- Sudoku in C
Geometric Algorithm
Geometric algorithms involve computations related to geometric objects such as points, lines, and polygons.
- Closest Pair of Points in C
- Line Intersection in C
- Point in Polygon in C
Data Structure and Algorithms in C – FAQs
What is a data structure.
A data structure is way of organizing data such that it optimizes some operations which we need to perform on it. For example, the array data structure is optimized for fast access, while linked list is optimized for fast insertion and deletion. Similarly, heaps are optimized for fast minimum and maximum retrieval.
What is an Algorithm?
An algorithm is a way of solving a problem. It is a step-by-step procedure to perform a specific task or to solve a particular problem. It consists of a finite sequence of well-defined instructions that take an input, process it, and produce an output. Algorithms are important because they provide a clear and efficient way to accomplish tasks that optimize performance of the program.
Is C a good language for learning Data Structures and Algorithms?
Yes, C is an excellent language for learning Data Structures and Algorithms because it provides a clear understanding of memory management and low-level data manipulation, which are important for implementing efficient algorithms.
How do you choose the right Data Structure in C?
The choice of data structure depends on the requirements of the task, such as the type of operations needed (e.g., search, insert, delete), memory constraints, and the complexity of those operations.
Please Login to comment...
Similar reads.
- How to Delete Discord Servers: Step by Step Guide
- Google increases YouTube Premium price in India: Check our the latest plans
- California Lawmakers Pass Bill to Limit AI Replicas
- Best 10 IPTV Service Providers in Germany
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Problem Solving and Program Design Chapter 1. Computer. Click the card to flip 👆. a machine that can receive, store, transform, and output data of all kinds. Click the card to flip 👆. 1 / 64.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Chapter 1 - 2 Problem Solving and Program Design. Flashcards. Learn. Test. Match. Term. 1 / 82. Millennials. ... is the combination of mechanical engineering, electronic engineering, and software engineering in order to design advanced hybrid systems. Other sets by this creator ...
Problem-solving on the Computer The design of any computer program involves two major phases: 1. 2. The Problem-Solving Phase The Implementation Phase The problem-solving phase comprises the following steps: Step 1:Define the problem Step 2:Find a solution to the problem Step 3:Evaluate alternative solutions Step 4:Represent the most efficient ...
Chapter 1, Problem 1RQ is solved. ... Unlike static PDF Problem Solving and Program Design in C 8th Edition solution manuals or printed answer keys, our experts show you how to solve each problem step-by-step. No need to wait for office hours or assignments to be graded to find out where you took a wrong turn. You can check your reasoning as ...
Chapter 1 Introduction to Computers and Programming. 1.1 Electronic Computers Then and Now 1.2 Components of a Computer 1.3 Problem Solving and Programming 1.4 The Software Development Method 1.5 Programming Languages 1.6 Processing a High-Level Language Program 1.7 Using an Operating System Chapter Review. Chapter 2 Introduction to Ada 95. 2.1 ...
Step-by-step solution. Step 1 of 2. A computer stores the information in different formats which are given below. • Number format. • Text format. • Image format. • Graphical format. • Sound format. Step 2 of 2.
First, lay out your starting node, as every one of your programs will have these. Start. Next, begin adding your program elements sequentially, in the order that your problem description indicated. Connect the elements of your flowchart by uni-directional arrows that indicate the flow of your program.
Chapter 3: Top-Down Design with Functions 3.1. Building Programs from Existing Information Case Study: Finding the Area and Circumference of a Circle ... Problem Solving and Program Design in C teaches introductory students to program with ANSI-C, a standardised, industrial-strength programming language known for its power and probability. The ...
BiBTeX EndNote RefMan. Problem Solving and Program Design in C is one of the best-selling introductory programming textbook using the C programming language for beginning programmers. It embraces a balanced approach to program development and an introduction to ANSI C. and provides a gradual introduction to pointers and covers programming with ...
CHAPTER 1. ew of Programming and Problem SolvingKnowled. e Goals To understand what a comput. r program is. To understand what an algorithm is. . To learn what a high-level programming language is. . o understand the compilation and execution pr. cesses. To learn the history of the C++ language. To learn what the major components of a computer ...
to apply problem solving techniques. Problem solving begins with the precise identification of the problem and ends with a complete working solution in terms of a program or software. Key steps required for solving a problem using a computer are shown in Figure 4.1 and are discussed in following subsections. 4.2.1 Analysing the problem
/* Write a program to assist the design of a hydroelectric dam. Prompt the user for the height of the dam and for the number of cubic meters of water * that are projected to flow from the top to the bottom of the dam of each second. * Predict how many megawatts (1MW = 10^6W) of power will be produced if 90% of the work done on the water by gravity is converted to electrical energy.
This new edition includes thoroughly updated end-of-chapter exercises, more than 30 new programming exercises, and many new examples created by Dr. Malik to further strengthen student understanding of problem solving and program design. New features of the C++ 11 Standard are discussed, ensuring this text best meets the needs of the modern CS1 ...
Objectives. To explain what problem solving is, and why it is important (0.1). To understand how to write algorithms (0.1-0.5). To describe how a program can be designed (0.2-0.3). To describe algorithms in different forms (0.4). To understand the difference between algorithms and pseudocode (0.4). To draw program flowcharts (0.4-0.5).
Problem Solving & Program Design in C by Jeri R. Hanly, Elliot B. Koffman Topics. c data-structures dynamic-programming programming-exercises Resources. Readme License. MIT license Activity. Stars. 18 stars Watchers. 6 watching Forks. 15 forks Report repository Releases 1.
otes) Chapter: 2.1 Algorithm design and problem-solvingTop down/modular designAdvantage. iques, including structure diagrams, showing stepwise refinementUDefinition:UA Top-down design is when a problem is split into smaller sub-problems, which themselves are split int. final program. UBenefitsand drawbacks of modular programsStructure.
Access Problem Solving and Program Design in C 8th Edition Chapter 1.3 Problem 3SC solution now. Our solutions are written by Chegg experts so you can be assured of the highest quality! ... Problem Solving and Program Design in C | 8th Edition. ISBN-13: 9780134014890 ISBN: 0134014898 Authors: ...
peaxxhes. Objectives of this chapter: -Outline the steps of problem-solving -Use a divide and conquer approach to decompose large everyday problems into smaller tasks -Define a problem by decomposing into significant components -Distinguish between variables and constants -Explain the concepts of algorithms -Represent algorithms in the form of ...
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems and storing the solutions to avoid redundant calculations. Following are some standard Dynamic Programming algorithms: Rod Cutting Problem in C; Coin Change Problem in C; Subset Sum Problem in C; 0-1 Knapsack in C; Edit Distance in C