How to Use the Ternary Operator in JavaScript – JS Conditional Example
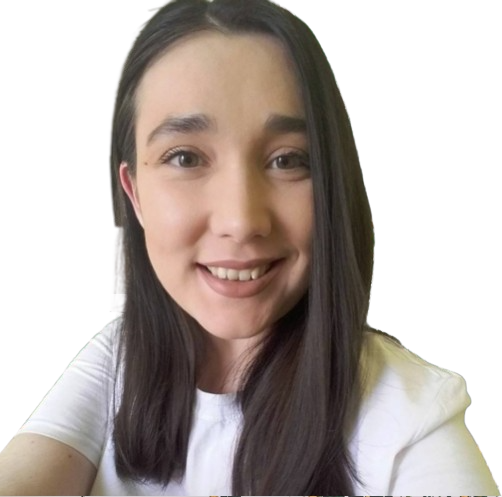
The ternary operator is a helpful feature in JavaScript that allows you to write concise and readable expressions that perform conditional operations on only one line.
In this article, you will learn why you may want to use the ternary operator, and you will see an example of how to use it. You will also learn some of the best practices to keep in mind when you do use it.
Let's get into it!

What Is The Ternary Operator in JavaScript?
The ternary operator ( ?: ), also known as the conditional operator, is a shorthand way of writing conditional statements in JavaScript – you can use a ternary operator instead of an if..else statement.
A ternary operator evaluates a given condition, also known as a Boolean expression, and returns a result that depends on whether that condition evaluates to true or false .
Why Use the Ternary Operator in JavaScript?
You may want to use the ternary operator for a few reasons:
- Your code will be more concise : The ternary operator has minimal syntax. You will write short conditional statements and fewer lines of code, which makes your code easier to read and understand.
- Your code will be more readable : When writing simple conditions, the ternary operator makes your code easier to understand in comparison to an if..else statement.
- Your code will be more organized : The ternary operator will make your code more organized and easier to maintain. This comes in handy when writing multiple conditional statements. The ternary operator will reduce the amount of nesting that occurs when using if..else statements.
- It provides flexibility : The ternary operator has many use cases, some of which include: assigning a value to a variable, rendering dynamic content on a web page, handling function arguments, validating data and handling errors, and creating complex expressions.
- It enhances performance : In some cases, the ternary operator can perform better than an if..else statement because the ternary operator gets evaluated in a single step.
- It always returns a value : The ternary operator always has to return something.
How to Use the Ternary Operator in JavaScript – a Syntax Overview
The operator is called "ternary" because it is composed of three parts: one condition and two expressions.
The general syntax for the ternary operator looks something similar to the following:
Let's break it down:
- condition is the Boolean expression you want to evaluate and determine whether it is true or false . The condition is followed by a question mark, ? .
- ifTrueExpression is executed if the condition evaluates to true .
- ifFalseExpression is executed if the condition evaluates to false .
- The two expressions are separated by a colon, . .
The ternary operator always returns a value that you assign to a variable:
Next, let's look at an example of how the ternary operator works.
How to Use the Ternary Operator in JavaScript
Say that you want to check whether a user is an adult:
In this example, I used the ternary operator to determine whether a user's age is greater than or equal to 18 .
Firstly, I used the prompt() built-in JavaScript function.
This function opens a dialog box with the message What is your age? and the user can enter a value.
I store the user's input in the age variable.
Next, the condition ( age >= 18 ) gets evaluated.
If the condition is true , the first expression, You are an adult , gets executed.
Say the user enters the value 18 .
The condition age >= 18 evaluates to true :
If the condition is false , the second expression, You are not an adult yet , gets executed.
Say the user enters the value 17 .
The condition age >= 18 now evaluates to false :
As mentioned earlier, you can use the ternary operator instead of an if..else statement.
Here is how you would write the same code used in the example above using an if..else statement:
Ternary Operator Best Practices in JavaScript
Something to keep in mind when using the ternary operator is to keep it simple and don't overcomplicate it.
The main goal is for your code to be readable and easily understandable for the rest of the developers on your team.
So, consider using the ternary operator for simple statements and as a concise alternative to if..else statements that can be written in one line.
If you do too much, it can quickly become unreadable.
For example, in some cases, using nested ternary operators can make your code hard to read:
If you find yourself nesting too many ternary operators, consider using if...else statements instead.
Wrapping Up
Overall, the ternary operator is a useful feature in JavaScript as it helps make your code more readable and concise.
Use it when a conditional statement can be written on only one line and keep code readability in mind.
Thanks for reading, and happy coding! :)
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Home » JavaScript Tutorial » JavaScript Ternary Operator
JavaScript Ternary Operator
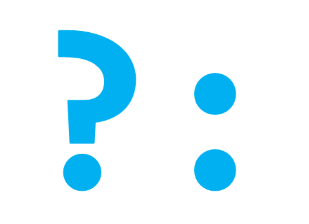
Summary : in this tutorial, you will learn how to use the JavaScript ternary operator to make your code more concise.
Introduction to JavaScript ternary operator
When you want to execute a block if a condition evaluates to true , you often use an if…else statement. For example:
In this example, we show a message that a person can drive if the age is greater than or equal to 16. Alternatively, you can use a ternary operator instead of the if-else statement like this:
Or you can use the ternary operator in an expression as follows:
Here’s the syntax of the ternary operator:
In this syntax, the condition is an expression that evaluates to a Boolean value, either true or false .
If the condition is true , the first expression ( expresionIfTrue ) executes. If it is false, the second expression ( expressionIfFalse ) executes.
The following shows the syntax of the ternary operator used in an expression:
In this syntax, if the condition is true , the variableName will take the result of the first expression ( expressionIfTrue ) or expressionIfFalse otherwise.
JavaScript ternary operator examples
Let’s take some examples of using the ternary operator.
1) Using the JavaScript ternary operator to perform multiple statements
The following example uses the ternary operator to perform multiple operations, where each operation is separated by a comma. For example:
In this example, the returned value of the ternary operator is the last value in the comma-separated list.
2) Simplifying ternary operator example
See the following example:
If the locked is 1, then the canChange variable is set to false , otherwise, it is set to true . In this case, you can simplify it by using a Boolean expression as follows:
Description
Specifications, browser compatibility.
The conditional (ternary) operator is the only JavaScript operator that takes three operands. This operator is frequently used as a shortcut for the if statement.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
Besides false , possible falsy expressions are: null , NaN , 0 , the empty string ( "" ), and undefined . If condition is any of these, the result of the conditional expression will be exprIfFalse .
A simple example:
One common usage is to handle a value that may be null :
Conditional chains
The ternary operator is right-associative, which means it can be "chained" in the following way, similar to an if … else if … else if … else chain:
- if statement
Document Tags and Contributors
- Conditional
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Continue Statement
- Garbage Collection in JavaScript
- Introduction to Three.js
- Synchronous and Asynchronous in JavaScript
- JavaScript Label Statement
- JavaScript | DataView()
- Javascript Error and Exceptional Handling With Examples
- Dynamic User Interfaces in JavaScript
- Control Statements in JavaScript
- JavaScript Loops
- Global and Local variables in JavaScript
- Static Methods in JavaScript
- JavaScript Versions
- JavaScript Variables
- Difference Between Variables and Objects in JavaScript
- Interesting Facts About Javascript
- Top 7 One liners of JavaScript
- JavaScript Code Execution
- JavaScript SyntaxError - A declaration in the head of a for-of loop can't have an initializer
Conditional Statements in JavaScript
JavaScript Conditional statements allow you to execute specific blocks of code based on conditions. If the condition meets then a particular block of action will be executed otherwise it will execute another block of action that satisfies that particular condition.
There are several methods that can be used to perform Conditional Statements in JavaScript.
- if statement : Executes a block of code if a specified condition is true.
- else statement : Executes a block of code if the same condition of the preceding if statement is false.
- else if statement : Adds more conditions to the if statement, allowing for multiple alternative conditions to be tested.
- switch statement : Evaluates an expression, then executes the case statement that matches the expression’s value.
- ternary operator (conditional operator) : Provides a concise way to write if-else statements in a single line.
JavaScript Conditional statements Examples:
1. using if statement.
The if statement is used to evaluate a particular condition. If the condition holds true, the associated code block is executed.
Example: In this example, we are using the if statement to find given number is even or odd.
Explanation: This JavaScript code determines if the variable `num` is even or odd using the modulo operator `%`. If `num` is divisible by 2 without a remainder, it logs “Given number is even number.” Otherwise, it logs “Given number is odd number.”
2. Using if-else Statement
The if-else statement will perform some action for a specific condition. Here we are using the else statement in which the else statement is written after the if statement and it has no condition in their code block.
Example: In this example, we are using if-else conditional statement to check the driving licence eligibility date.
Explanation: This JavaScript code checks if the variable `age` is greater than or equal to 18. If true, it logs “You are eligible for a driving license.” Otherwise, it logs “You are not eligible for a driving license.” This indicates eligibility for driving based on age.
3. else if Statement
The else if statement in JavaScript allows handling multiple possible conditions and outputs, evaluating more than two options based on whether the conditions are true or false.
Example: In this example, we are using the above-explained approach.
Explanation: This JavaScript code determines whether the constant `num` is positive, negative, or zero. If `num` is greater than 0, it logs “Given number is positive.” If `num` is less than 0, it logs “Given number is negative.” If neither condition is met (i.e., `num` is zero), it logs “Given number is zero.”
4. Using Switch Statement (JavaScript Switch Case)
As the number of conditions increases, you can use multiple else-if statements in JavaScript. but when we dealing with many conditions, the switch statement may be a more preferred option.
Example: In this example, we find a branch name Based on the student’s marks, this switch statement assigns a specific engineering branch to the variable Branch. The output displays the student’s branch name,
Explanation:
This JavaScript code assigns a branch of engineering to a student based on their marks. It uses a switch statement with cases for different mark ranges. The student’s branch is determined according to their marks and logged to the console.

5. Using Ternary Operator ( ?: )
The conditional operator, also referred to as the ternary operator (?:), is a shortcut for expressing conditional statements in JavaScript.
Example: In this example, we use the ternary operator to check if the user’s age is 18 or older. It prints eligibility for voting based on the condition.
Explanation: This JavaScript code checks if the variable `age` is greater than or equal to 18. If true, it assigns the string “You are eligible to vote.” to the variable `result`. Otherwise, it assigns “You are not eligible to vote.” The value of `result` is then logged to the console.
Conditional Statements in JavaScript Use-Cases:
1. javascript if-else.
JavaScript if-else or conditional statements will perform some action for a specific condition.
2. Control Statements in JavaScript
Control Statements are used to control the flow of the program with the help of conditional statements.
Please Login to comment...
Similar reads.
- javascript-basics
- Web Technologies
- Google Introduces New AI-powered Vids App
- Dolly Chaiwala: The Microsoft Windows 12 Brand Ambassador
- 10 Best Free Remote Desktop apps for Android in 2024
- 10 Best Free Internet Speed Test apps for Android in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

COMMENTS
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...
Inside the function, we use the ternary operator to check if the number is even or odd. If the number modulo 2 equals 0 (meaning it's divisible by 2 with no remainder), then the condition evaluates to true, and the string "even" is assigned to the result variable. If the condition evaluates to false (meaning the number is odd), the string "odd ...
Note that there is no elseif syntax in JavaScript. ... (x > 50) { /* do the right thing */ } else { /* do the right thing */ } Assignment within the conditional expression. It is advisable to not use simple assignments in a conditional expression, because the assignment can be confused with equality when glancing over the code. For example, do ...
Overview: Building blocks Next. In any programming language, code needs to make decisions and carry out actions accordingly depending on different inputs. For example, in a game, if the player's number of lives is 0, then it's game over. In a weather app, if it is being looked at in the morning, show a sunrise graphic; show stars and a moon if ...
Here we've got: The keyword switch, followed by a set of parentheses.; An expression or value inside the parentheses. The keyword case, followed by a choice that the expression/value could be, followed by a colon.; Some code to run if the choice matches the expression. A break statement, followed by a semicolon. If the previous choice matches the expression/value, the browser stops executing ...
In this example, I used the ternary operator to determine whether a user's age is greater than or equal to 18. Firstly, I used the prompt() built-in JavaScript function. This function opens a dialog box with the message What is your age? and the user can enter a value. I store the user's input in the age variable.
Assignment in a conditional statement is valid in javascript, because your just asking "if assignment is valid, do something which possibly includes the result of the assignment". But indeed, assigning before the conditional is also valid, not too verbose, and more commonly used. - okdewit.
console .log(message); Code language: JavaScript (javascript) Here's the syntax of the ternary operator: In this syntax, the condition is an expression that evaluates to a Boolean value, either true or false. If the condition is true, the first expression ( expresionIfTrue) executes. If it is false, the second expression ( expressionIfFalse ...
Learn how to write and use expressions and operators in JavaScript, such as assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. This chapter provides detailed explanations and examples for each type of expression and operator, as well as links to related topics and references.
Syntax. Description. Specifications. Browser compatibility. See also. The conditional (ternary) operator is the only JavaScript operator that takes three operands. This operator is frequently used as a shortcut for the if statement. JavaScript Demo: Expressions - Conditional operator.
Actually, this is somewhere between incorrect and misleading. An assignment simply evaluates to its RHS. For instance, if geo is a property, it might have a getter, but that getter will never be called when evaluating the if condition. Then you say geo will then be coerced to a boolean.This is also incorrect.
switch statement: Evaluates an expression, then executes the case statement that matches the expression's value. ternary operator (conditional operator): Provides a concise way to write if-else statements in a single line. JavaScript Conditional statements Examples: 1. Using if Statement. The if statement is used to evaluate a particular ...
Assignment in conditional expression in JavaScript. Ask Question Asked 9 years, 8 months ago. Modified 9 years, 8 months ago. Viewed 179 times ... IF statement as assignment expression in JavaScript. 2. Assigning the value of a variable inside the condition of an if statemment. 0.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
It means you try to assign a value whereas,in the same time, you try to compare these values. To compares two values it's '==' or '===' . To assign a value to a variable it's '='. It means you assign a value where you're meant to compare two values.
javascript: is this a conditional assignment? 2. ... Javascript - Weird if syntax. 5. IF statement as assignment expression in JavaScript. 1. Syntax matter. Conditional statement. 0. javascript: If-statement - Expected an assignment or function call and instead saw an expression. 3.