

1.4 — Variable assignment and initialization
In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we’ll explore how to actually put values into variables and use those values.
As a reminder, here’s a short snippet that first allocates a single integer variable named x , then allocates two more integer variables named y and z :
Variable assignment
After a variable has been defined, you can give it a value (in a separate statement) using the = operator . This process is called assignment , and the = operator is called the assignment operator .
By default, assignment copies the value on the right-hand side of the = operator to the variable on the left-hand side of the operator. This is called copy assignment .
Here’s an example where we use assignment twice:
This prints:
When we assign value 7 to variable width , the value 5 that was there previously is overwritten. Normal variables can only hold one value at a time.
One of the most common mistakes that new programmers make is to confuse the assignment operator ( = ) with the equality operator ( == ). Assignment ( = ) is used to assign a value to a variable. Equality ( == ) is used to test whether two operands are equal in value.
Initialization
One downside of assignment is that it requires at least two statements: one to define the variable, and another to assign the value.
These two steps can be combined. When an object is defined, you can optionally give it an initial value. The process of specifying an initial value for an object is called initialization , and the syntax used to initialize an object is called an initializer .
In the above initialization of variable width , { 5 } is the initializer, and 5 is the initial value.
Different forms of initialization
Initialization in C++ is surprisingly complex, so we’ll present a simplified view here.
There are 6 basic ways to initialize variables in C++:
You may see the above forms written with different spacing (e.g. int d{7}; ). Whether you use extra spaces for readability or not is a matter of personal preference.
Default initialization
When no initializer is provided (such as for variable a above), this is called default initialization . In most cases, default initialization performs no initialization, and leaves a variable with an indeterminate value.
We’ll discuss this case further in lesson ( 1.6 -- Uninitialized variables and undefined behavior ).
Copy initialization
When an initial value is provided after an equals sign, this is called copy initialization . This form of initialization was inherited from C.
Much like copy assignment, this copies the value on the right-hand side of the equals into the variable being created on the left-hand side. In the above snippet, variable width will be initialized with value 5 .
Copy initialization had fallen out of favor in modern C++ due to being less efficient than other forms of initialization for some complex types. However, C++17 remedied the bulk of these issues, and copy initialization is now finding new advocates. You will also find it used in older code (especially code ported from C), or by developers who simply think it looks more natural and is easier to read.
For advanced readers
Copy initialization is also used whenever values are implicitly copied or converted, such as when passing arguments to a function by value, returning from a function by value, or catching exceptions by value.
Direct initialization
When an initial value is provided inside parenthesis, this is called direct initialization .
Direct initialization was initially introduced to allow for more efficient initialization of complex objects (those with class types, which we’ll cover in a future chapter). Just like copy initialization, direct initialization had fallen out of favor in modern C++, largely due to being superseded by list initialization. However, we now know that list initialization has a few quirks of its own, and so direct initialization is once again finding use in certain cases.
Direct initialization is also used when values are explicitly cast to another type.
One of the reasons direct initialization had fallen out of favor is because it makes it hard to differentiate variables from functions. For example:
List initialization
The modern way to initialize objects in C++ is to use a form of initialization that makes use of curly braces. This is called list initialization (or uniform initialization or brace initialization ).
List initialization comes in three forms:
As an aside…
Prior to the introduction of list initialization, some types of initialization required using copy initialization, and other types of initialization required using direct initialization. List initialization was introduced to provide a more consistent initialization syntax (which is why it is sometimes called “uniform initialization”) that works in most cases.
Additionally, list initialization provides a way to initialize objects with a list of values (which is why it is called “list initialization”). We show an example of this in lesson 16.2 -- Introduction to std::vector and list constructors .
List initialization has an added benefit: “narrowing conversions” in list initialization are ill-formed. This means that if you try to brace initialize a variable using a value that the variable can not safely hold, the compiler is required to produce a diagnostic (usually an error). For example:
In the above snippet, we’re trying to assign a number (4.5) that has a fractional part (the .5 part) to an integer variable (which can only hold numbers without fractional parts).
Copy and direct initialization would simply drop the fractional part, resulting in the initialization of value 4 into variable width . Your compiler may optionally warn you about this, since losing data is rarely desired. However, with list initialization, your compiler is required to generate a diagnostic in such cases.
Conversions that can be done without potential data loss are allowed.
To summarize, list initialization is generally preferred over the other initialization forms because it works in most cases (and is therefore most consistent), it disallows narrowing conversions, and it supports initialization with lists of values (something we’ll cover in a future lesson). While you are learning, we recommend sticking with list initialization (or value initialization).
Best practice
Prefer direct list initialization (or value initialization) for initializing your variables.
Author’s note
Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) also recommend using list initialization to initialize your variables.
In modern C++, there are some cases where list initialization does not work as expected. We cover one such case in lesson 16.2 -- Introduction to std::vector and list constructors .
Because of such quirks, some experienced developers now advocate for using a mix of copy, direct, and list initialization, depending on the circumstance. Once you are familiar enough with the language to understand the nuances of each initialization type and the reasoning behind such recommendations, you can evaluate on your own whether you find these arguments persuasive.
Value initialization and zero initialization
When a variable is initialized using empty braces, value initialization takes place. In most cases, value initialization will initialize the variable to zero (or empty, if that’s more appropriate for a given type). In such cases where zeroing occurs, this is called zero initialization .
Q: When should I initialize with { 0 } vs {}?
Use an explicit initialization value if you’re actually using that value.
Use value initialization if the value is temporary and will be replaced.
Initialize your variables
Initialize your variables upon creation. You may eventually find cases where you want to ignore this advice for a specific reason (e.g. a performance critical section of code that uses a lot of variables), and that’s okay, as long the choice is made deliberately.
Related content
For more discussion on this topic, Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) make this recommendation themselves here .
We explore what happens if you try to use a variable that doesn’t have a well-defined value in lesson 1.6 -- Uninitialized variables and undefined behavior .
Initialize your variables upon creation.
Initializing multiple variables
In the last section, we noted that it is possible to define multiple variables of the same type in a single statement by separating the names with a comma:
We also noted that best practice is to avoid this syntax altogether. However, since you may encounter other code that uses this style, it’s still useful to talk a little bit more about it, if for no other reason than to reinforce some of the reasons you should be avoiding it.
You can initialize multiple variables defined on the same line:
Unfortunately, there’s a common pitfall here that can occur when the programmer mistakenly tries to initialize both variables by using one initialization statement:
In the top statement, variable “a” will be left uninitialized, and the compiler may or may not complain. If it doesn’t, this is a great way to have your program intermittently crash or produce sporadic results. We’ll talk more about what happens if you use uninitialized variables shortly.
The best way to remember that this is wrong is to consider the case of direct initialization or brace initialization:
Because the parenthesis or braces are typically placed right next to the variable name, this makes it seem a little more clear that the value 5 is only being used to initialize variable b and d , not a or c .
Unused initialized variables warnings
Modern compilers will typically generate warnings if a variable is initialized but not used (since this is rarely desirable). And if “treat warnings as errors” is enabled, these warnings will be promoted to errors and cause the compilation to fail.
Consider the following innocent looking program:
When compiling this with the g++ compiler, the following error is generated:
and the program fails to compile.
There are a few easy ways to fix this.
- If the variable really is unused, then the easiest option is to remove the defintion of x (or comment it out). After all, if it’s not used, then removing it won’t affect anything.
- Another option is to simply use the variable somewhere:
But this requires some effort to write code that uses it, and has the downside of potentially changing your program’s behavior.
The [[maybe_unused]] attribute C++17
In some cases, neither of the above options are desirable. Consider the case where we have a bunch of math/physics values that we use in many different programs:
If we use these a lot, we probably have these saved somewhere and copy/paste/import them all together.
However, in any program where we don’t use all of these values, the compiler will complain about each variable that isn’t actually used. While we could go through and remove/comment out the unused ones for each program, this takes time and energy. And later if we need one that we’ve previously removed, we’ll have to go back and re-add it.
To address such cases, C++17 introduced the [[maybe_unused]] attribute, which allows us to tell the compiler that we’re okay with a variable being unused. The compiler will not generate unused variable warnings for such variables.
The following program should generate no warnings/errors:
Additionally, the compiler will likely optimize these variables out of the program, so they have no performance impact.
In future lessons, we’ll often define variables we don’t use again, in order to demonstrate certain concepts. Making use of [[maybe_unused]] allows us to do so without compilation warnings/errors.
Question #1
What is the difference between initialization and assignment?
Show Solution
Initialization gives a variable an initial value at the point when it is created. Assignment gives a variable a value at some point after the variable is created.
Question #2
What form of initialization should you prefer when you want to initialize a variable with a specific value?
Direct list initialization (aka. direct brace initialization).
Question #3
What are default initialization and value initialization? What is the behavior of each? Which should you prefer?
Default initialization is when a variable initialization has no initializer (e.g. int x; ). In most cases, the variable is left with an indeterminate value.
Value initialization is when a variable initialization has an empty brace (e.g. int x{}; ). In most cases this will perform zero-initialization.
You should prefer value initialization to default initialization.
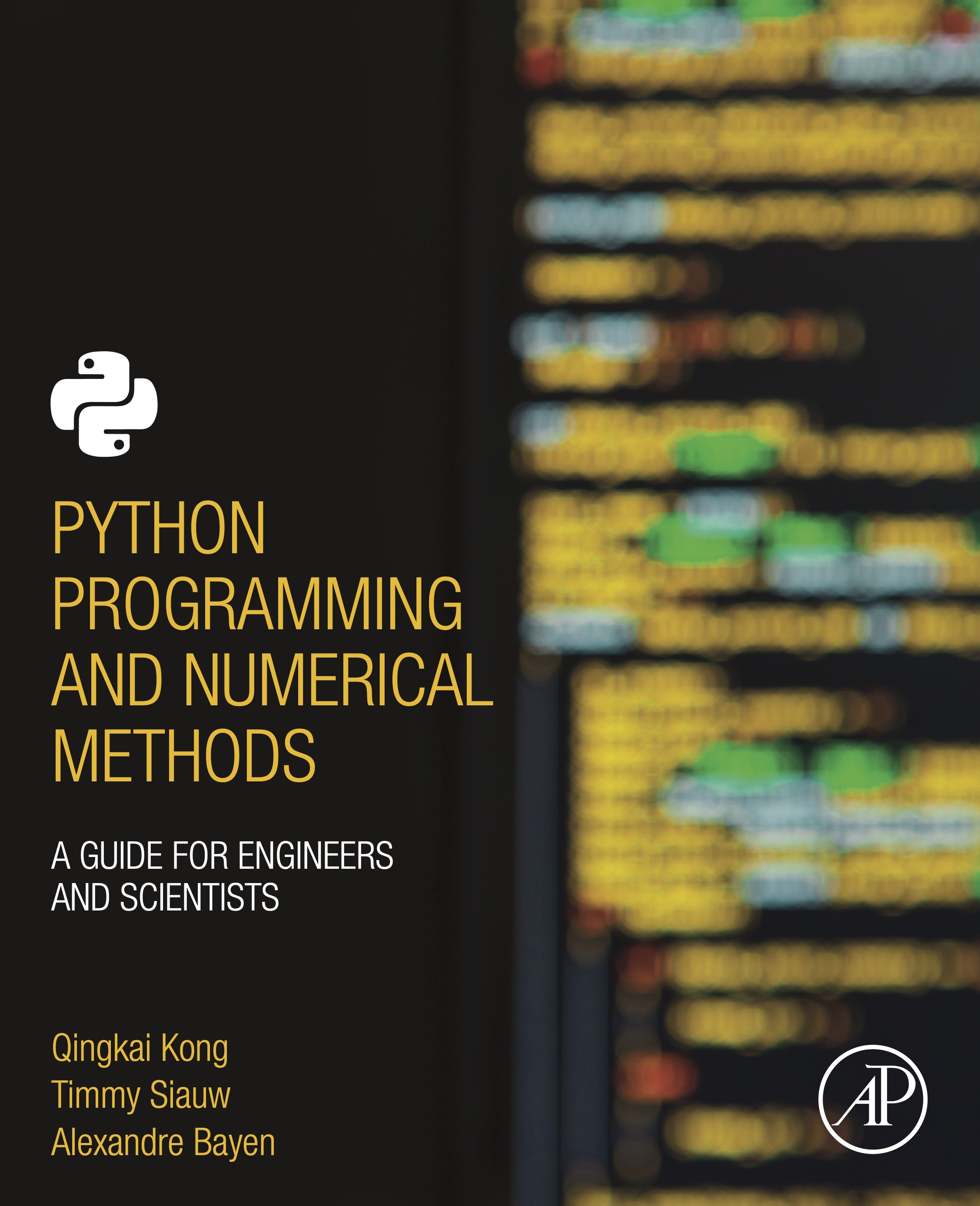
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
How to assign a variable in Python

Sign in to change your settings
Sign in to your Python Morsels account to save your screencast settings.
Don't have an account yet? Sign up here .
How can you assign a variable in Python?
An equals sign assigns in Python
In Python, the equal sign ( = ) assigns a variable to a value :
This is called an assignment statement . We've pointed the variable count to the value 4 .
We don't have declarations or initializations
Some programming languages have an idea of declaring variables .
Declaring a variable says a variable exists with this name, but it doesn't have a value yet . After you've declared the variable, you then have to initialize it with a value.
Python doesn't have the concept of declaring a variable or initializing variables . Other programming languages sometimes do, but we don't.
In Python, a variable either exists or it doesn't:
If it doesn't exist, assigning to that variable will make it exist:
Valid variable names in Python
Variables in Python can be made up of letters, numbers, and underscores:
The first character in a variable cannot be a number :
And a small handful of names are reserved , so they can't be used as variable names (as noted in SyntaxError: invalid syntax ):
Reassigning a variable in Python
What if you want to change the value of a variable ?
The equal sign is used to assign a variable to a value, but it's also used to reassign a variable:
In Python, there's no distinction between assignment and reassignment .
Whenever you assign a variable in Python, if a variable with that name doesn't exist yet, Python makes a new variable with that name . But if a variable does exist with that name, Python points that variable to the value that we're assigning it to .
Variables don't have types in Python
Note that in Python, variables don't care about the type of an object .
Our amount variable currently points to an integer:
But there's nothing stopping us from pointing it to a string instead:
Variables in Python don't have types associated with them. Objects have types but variables don't . You can point a variable to any object that you'd like.
Type annotations are really type hints
You might have seen a variable that seems to have a type. This is called a type annotation (a.k.a. a "type hint"):
But when you run code like this, Python pretty much ignores these type hints. These are useful as documentation , and they can be introspected at runtime. But type annotations are not enforced by Python . Meaning, if we were to assign this variable to a different type, Python won't care:
What's the point of that?
Well, there's a number of code analysis tools that will check type annotations and show errors if our annotations don't match.
One of these tools is called MyPy .
Type annotations are something that you can opt into in Python, but Python won't do type-checking for you . If you want to enforce type annotations in your code, you'll need to specifically run a type-checker (like MyPy) before your code runs.
Use = to assign a variable in Python
Assignment in Python is pretty simple on its face, but there's a bit of complexity below the surface.
For example, Python's variables are not buckets that contain objects : Python's variables are pointers . Also you can assign into data structures in Python.
Also, it's actually possible to assign without using an equal sign in Python. But the equal sign ( = ) is the quick and easy way to assign a variable in Python.
What comes after Intro to Python?
Intro to Python courses often skip over some fundamental Python concepts .
Sign up below and I'll explain concepts that new Python programmers often overlook .
Series: Assignment and Mutation
Python's variables aren't buckets that contain things; they're pointers that reference objects.
The way Python's variables work can often confuse folks new to Python, both new programmers and folks moving from other languages like C++ or Java.
To track your progress on this Python Morsels topic trail, sign in or sign up .
Sign up below and I'll share ideas new Pythonistas often overlook .
Python's variables are not buckets that contain objects; they're pointers. Assignment statements don't copy: they point a variable to a value (and multiple variables can "point" to the same value).
- Module 2: The Essentials of Python »
- Variables & Assignment
- View page source
Variables & Assignment
There are reading-comprehension exercises included throughout the text. These are meant to help you put your reading to practice. Solutions for the exercises are included at the bottom of this page.
Variables permit us to write code that is flexible and amendable to repurpose. Suppose we want to write code that logs a student’s grade on an exam. The logic behind this process should not depend on whether we are logging Brian’s score of 92% versus Ashley’s score of 94%. As such, we can utilize variables, say name and grade , to serve as placeholders for this information. In this subsection, we will demonstrate how to define variables in Python.
In Python, the = symbol represents the “assignment” operator. The variable goes to the left of = , and the object that is being assigned to the variable goes to the right:
Attempting to reverse the assignment order (e.g. 92 = name ) will result in a syntax error. When a variable is assigned an object (like a number or a string), it is common to say that the variable is a reference to that object. For example, the variable name references the string "Brian" . This means that, once a variable is assigned an object, it can be used elsewhere in your code as a reference to (or placeholder for) that object:
Valid Names for Variables
A variable name may consist of alphanumeric characters ( a-z , A-Z , 0-9 ) and the underscore symbol ( _ ); a valid name cannot begin with a numerical value.
var : valid
_var2 : valid
ApplePie_Yum_Yum : valid
2cool : invalid (begins with a numerical character)
I.am.the.best : invalid (contains . )
They also cannot conflict with character sequences that are reserved by the Python language. As such, the following cannot be used as variable names:
for , while , break , pass , continue
in , is , not
if , else , elif
def , class , return , yield , raises
import , from , as , with
try , except , finally
There are other unicode characters that are permitted as valid characters in a Python variable name, but it is not worthwhile to delve into those details here.
Mutable and Immutable Objects
The mutability of an object refers to its ability to have its state changed. A mutable object can have its state changed, whereas an immutable object cannot. For instance, a list is an example of a mutable object. Once formed, we are able to update the contents of a list - replacing, adding to, and removing its elements.
To spell out what is transpiring here, we:
Create (initialize) a list with the state [1, 2, 3] .
Assign this list to the variable x ; x is now a reference to that list.
Using our referencing variable, x , update element-0 of the list to store the integer -4 .
This does not create a new list object, rather it mutates our original list. This is why printing x in the console displays [-4, 2, 3] and not [1, 2, 3] .
A tuple is an example of an immutable object. Once formed, there is no mechanism by which one can change of the state of a tuple; and any code that appears to be updating a tuple is in fact creating an entirely new tuple.
Mutable & Immutable Types of Objects
The following are some common immutable and mutable objects in Python. These will be important to have in mind as we start to work with dictionaries and sets.
Some immutable objects
numbers (integers, floating-point numbers, complex numbers)
“frozen”-sets
Some mutable objects
dictionaries
NumPy arrays
Referencing a Mutable Object with Multiple Variables
It is possible to assign variables to other, existing variables. Doing so will cause the variables to reference the same object:
What this entails is that these common variables will reference the same instance of the list. Meaning that if the list changes, all of the variables referencing that list will reflect this change:
We can see that list2 is still assigned to reference the same, updated list as list1 :
In general, assigning a variable b to a variable a will cause the variables to reference the same object in the system’s memory, and assigning c to a or b will simply have a third variable reference this same object. Then any change (a.k.a mutation ) of the object will be reflected in all of the variables that reference it ( a , b , and c ).
Of course, assigning two variables to identical but distinct lists means that a change to one list will not affect the other:
Reading Comprehension: Does slicing a list produce a reference to that list?
Suppose x is assigned a list, and that y is assigned a “slice” of x . Do x and y reference the same list? That is, if you update part of the subsequence common to x and y , does that change show up in both of them? Write some simple code to investigate this.
Reading Comprehension: Understanding References
Based on our discussion of mutable and immutable objects, predict what the value of y will be in the following circumstance:
Reading Comprehension Exercise Solutions:
Does slicing a list produce a reference to that list?: Solution
Based on the following behavior, we can conclude that slicing a list does not produce a reference to the original list. Rather, slicing a list produces a copy of the appropriate subsequence of the list:
Understanding References: Solutions
Integers are immutable, thus x must reference an entirely new object ( 9 ), and y still references 3 .
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python variables, varia b l e s.
Variables are containers for storing data values.
Creating Variables
Python has no command for declaring a variable.
A variable is created the moment you first assign a value to it.
Variables do not need to be declared with any particular type , and can even change type after they have been set.
If you want to specify the data type of a variable, this can be done with casting.
Advertisement
Get the Type
You can get the data type of a variable with the type() function.
Single or Double Quotes?
String variables can be declared either by using single or double quotes:
Case-Sensitive
Variable names are case-sensitive.
This will create two variables:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- CBSE Class 11 Informatics Practices Syllabus 2023-24
- CBSE Class 11 Information Practices Syllabus 2023-24 Distribution of Marks
- CBSE Class 11 Informatics Practices Unit-wise Syllabus
- Unit 1:Introduction to Computer System
- Introduction to Computer System
- Evolution of Computer
- Computer Memory
- Unit 2:Introduction to Python
- Python Keywords
- Identifiers
- Expressions
- Input and Output
- if else Statements
- Nested Loops
- Working with Lists and Dictionaries
- Introduction to List
- List Operations
- Traversing a List
- List Methods and Built in Functions
- Introduction to Dictionaries
- Traversing a Dictionary
- Dictionary Methods and Built-in Functions
- Unit 3 Data Handling using NumPy
- Introduction
- NumPy Array
- Indexing and Slicing
- Operations on Arrays
- Concatenating Arrays
- Reshaping Arrays
- Splitting Arrays
- Saving NumPy Arrays in Files on Disk
- Unit 4: Database Concepts and the Structured Query Language
- Understanding Data
- Introduction to Data
- Data Collection
- Data Storage
- Data Processing
- Statistical Techniques for Data Processing
- Measures of Central Tendency
- Database Concepts
- Introduction to Structured Query Language
- Structured Query Language
- Data Types and Constraints in MySQL
- CREATE Database
- CREATE Table
- DESCRIBE Table
- ALTER Table
- SQL for Data Manipulation
- SQL for Data Query
- Data Updation and Deletion
- Unit 5 Introduction to Emerging Trends
- Artificial Intelligence
- Internet of Things
- Cloud Computing
- Grid Computing
- Blockchains
- Class 11 IP Syllabus Practical and Theory Components
Python Variables
Python Variable is containers that store values. Python is not “statically typed”. We do not need to declare variables before using them or declare their type. A variable is created the moment we first assign a value to it. A Python variable is a name given to a memory location. It is the basic unit of storage in a program. In this article, we will see how to define a variable in Python .
Example of Variable in Python
An Example of a Variable in Python is a representational name that serves as a pointer to an object. Once an object is assigned to a variable, it can be referred to by that name. In layman’s terms, we can say that Variable in Python is containers that store values.
Here we have stored “ Geeksforgeeks ” in a variable var , and when we call its name the stored information will get printed.
Notes: The value stored in a variable can be changed during program execution. A Variables in Python is only a name given to a memory location, all the operations done on the variable effects that memory location.
Rules for Python variables
- A Python variable name must start with a letter or the underscore character.
- A Python variable name cannot start with a number.
- A Python variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ).
- Variable in Python names are case-sensitive (name, Name, and NAME are three different variables).
- The reserved words(keywords) in Python cannot be used to name the variable in Python.
Variables Assignment in Python
Here, we will define a variable in python. Here, clearly we have assigned a number, a floating point number, and a string to a variable such as age, salary, and name.
Declaration and Initialization of Variables
Let’s see how to declare a variable and how to define a variable and print the variable.
Redeclaring variables in Python
We can re-declare the Python variable once we have declared the variable and define variable in python already.
Python Assign Values to Multiple Variables
Also, Python allows assigning a single value to several variables simultaneously with “=” operators. For example:
Assigning different values to multiple variables
Python allows adding different values in a single line with “,” operators.
Can We Use the S ame Name for Different Types?
If we use the same name, the variable starts referring to a new value and type.
How does + operator work with variables?
The Python plus operator + provides a convenient way to add a value if it is a number and concatenate if it is a string. If a variable is already created it assigns the new value back to the same variable.
Can we use + for different Datatypes also?
No use for different types would produce an error.
Global and Local Python Variables
Local variables in Python are the ones that are defined and declared inside a function. We can not call this variable outside the function.
Global variables in Python are the ones that are defined and declared outside a function, and we need to use them inside a function.
Global keyword in Python
Python global is a keyword that allows a user to modify a variable outside of the current scope. It is used to create global variables from a non-global scope i.e inside a function. Global keyword is used inside a function only when we want to do assignments or when we want to change a variable. Global is not needed for printing and accessing.
Rules of global keyword
- If a variable is assigned a value anywhere within the function’s body, it’s assumed to be local unless explicitly declared as global.
- Variables that are only referenced inside a function are implicitly global.
- We use a global in Python to use a global variable inside a function.
- There is no need to use a global keyword in Python outside a function.
Python program to modify a global value inside a function.
Variable Types in Python
Data types are the classification or categorization of data items. It represents the kind of value that tells what operations can be performed on a particular data. Since everything is an object in Python programming, data types are actually classes and variables are instances (object) of these classes.
Built-in Python Data types are:
- Sequence Type ( Python list , Python tuple , Python range )
In this example, we have shown different examples of Built-in data types in Python.
Object Reference in Python
Let us assign a variable x to value 5.
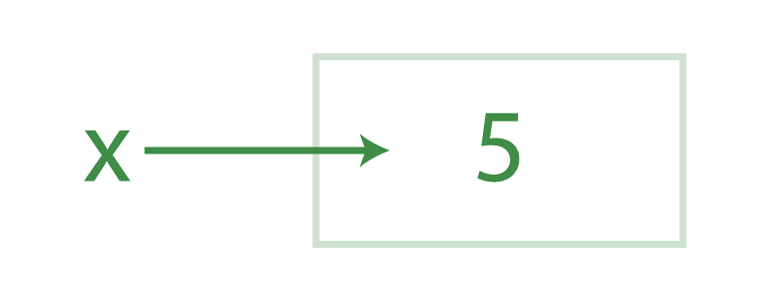
Another variable is y to the variable x.
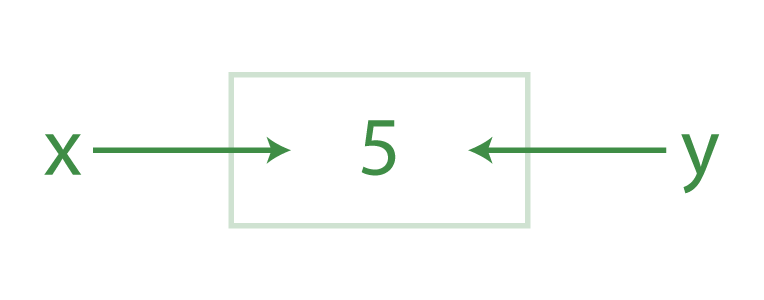
When Python looks at the first statement, what it does is that, first, it creates an object to represent the value 5. Then, it creates the variable x if it doesn’t exist and made it a reference to this new object 5. The second line causes Python to create the variable y, and it is not assigned with x, rather it is made to reference that object that x does. The net effect is that the variables x and y wind up referencing the same object. This situation, with multiple names referencing the same object, is called a Shared Reference in Python. Now, if we write:
This statement makes a new object to represent ‘Geeks’ and makes x reference this new object.
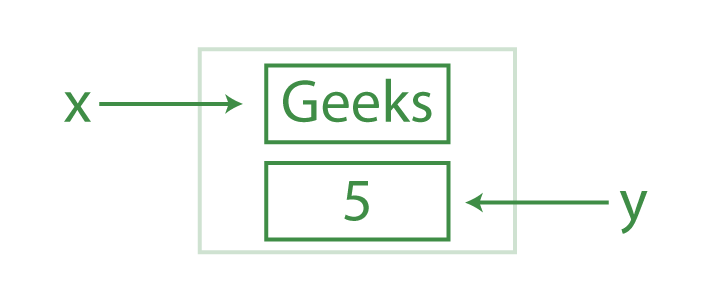
Now if we assign the new value in Y, then the previous object refers to the garbage values.
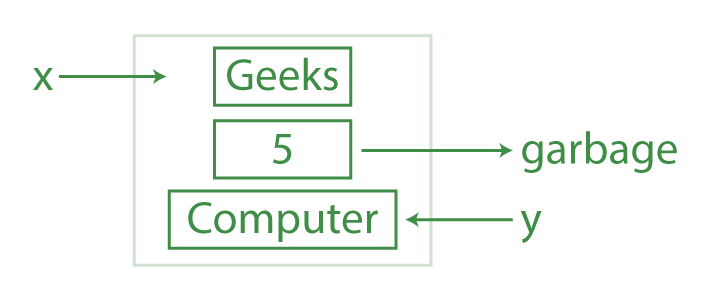
Creating objects (or variables of a class type)
Please refer to Class, Object, and Members for more details.
Frequently Asked Questions
1. how to define a variable in python.
In Python, we can define a variable by assigning a value to a name. Variable names must start with a letter (a-z, A-Z) or an underscore (_) and can be followed by letters, underscores, or digits (0-9). Python is dynamically typed, meaning we don’t need to declare the variable type explicitly; it will be inferred based on the assigned value.
2. Are there naming conventions for Python variables?
Yes, Python follows the snake_case convention for variable names (e.g., my_variable ). They should start with a letter or underscore, followed by letters, underscores, or numbers. Constants are usually named in ALL_CAPS.
3. Can I change the type of a Python variable?
Yes, Python is dynamically typed, meaning you can change the type of a variable by assigning a new value of a different type. For instance:
Please Login to comment...
Similar reads.
- python-basics
- School Programming
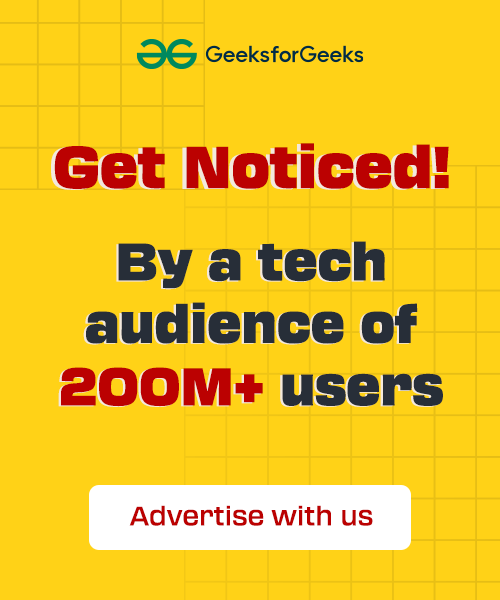
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Variables and Assignment Statements
- First Online: 23 August 2018
Cite this chapter
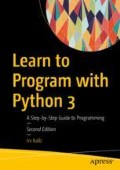
- Irv Kalb 2
5977 Accesses
This chapter covers the following topics:
A sample Python program
Building blocks of programming
Four types of data
What a variable is
Rules for naming variables
Giving a variable a value with an assignment statement
A good way to name variables
Special Python keywords
Case sensitivity
More complicated assignment statements
Print statements
Basic math operators
Order of operations and parentheses
A few small sample programs
Additional naming conventions
How to add comments in a program
Use of “whitespace”
Errors in programs
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Author information
Authors and affiliations.
Mountain View, California, USA
You can also search for this author in PubMed Google Scholar

Rights and permissions
Reprints and permissions
Copyright information
© 2018 Irv Kalb
About this chapter
Kalb, I. (2018). Variables and Assignment Statements. In: Learn to Program with Python 3. Apress, Berkeley, CA. https://doi.org/10.1007/978-1-4842-3879-0_2
Download citation
DOI : https://doi.org/10.1007/978-1-4842-3879-0_2
Published : 23 August 2018
Publisher Name : Apress, Berkeley, CA
Print ISBN : 978-1-4842-3878-3
Online ISBN : 978-1-4842-3879-0
eBook Packages : Professional and Applied Computing Apress Access Books Professional and Applied Computing (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
- Assignment Statement
An Assignment statement is a statement that is used to set a value to the variable name in a program .
Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted by a variable name.

The symbol used in an assignment statement is called as an operator . The symbol is ‘=’ .
Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’.
The Basic Syntax of Assignment Statement in a programming language is :
variable = expression ;
variable = variable name
expression = it could be either a direct value or a math expression/formula or a function call
Few programming languages such as Java, C, C++ require data type to be specified for the variable, so that it is easy to allocate memory space and store those values during program execution.
data_type variable_name = value ;
In the above-given examples, Variable ‘a’ is assigned a value in the same statement as per its defined data type. A data type is only declared for Variable ‘b’. In the 3 rd line of code, Variable ‘a’ is reassigned the value 25. The 4 th line of code assigns the value for Variable ‘b’.
Assignment Statement Forms
This is one of the most common forms of Assignment Statements. Here the Variable name is defined, initialized, and assigned a value in the same statement. This form is generally used when we want to use the Variable quite a few times and we do not want to change its value very frequently.
Tuple Assignment
Generally, we use this form when we want to define and assign values for more than 1 variable at the same time. This saves time and is an easy method. Note that here every individual variable has a different value assigned to it.
(Code In Python)
Sequence Assignment
(Code in Python)
Multiple-target Assignment or Chain Assignment
In this format, a single value is assigned to two or more variables.
Augmented Assignment
In this format, we use the combination of mathematical expressions and values for the Variable. Other augmented Assignment forms are: &=, -=, **=, etc.
Browse more Topics Under Data Types, Variables and Constants
- Concept of Data types
- Built-in Data Types
- Constants in Programing Language
- Access Modifier
- Variables of Built-in-Datatypes
- Declaration/Initialization of Variables
- Type Modifier
Few Rules for Assignment Statement
Few Rules to be followed while writing the Assignment Statements are:
- Variable names must begin with a letter, underscore, non-number character. Each language has its own conventions.
- The Data type defined and the variable value must match.
- A variable name once defined can only be used once in the program. You cannot define it again to store other types of value.
- If you assign a new value to an existing variable, it will overwrite the previous value and assign the new value.
FAQs on Assignment Statement
Q1. Which of the following shows the syntax of an assignment statement ?
- variablename = expression ;
- expression = variable ;
- datatype = variablename ;
- expression = datatype variable ;
Answer – Option A.
Q2. What is an expression ?
- Same as statement
- List of statements that make up a program
- Combination of literals, operators, variables, math formulas used to calculate a value
- Numbers expressed in digits
Answer – Option C.
Q3. What are the two steps that take place when an assignment statement is executed?
- Evaluate the expression, store the value in the variable
- Reserve memory, fill it with value
- Evaluate variable, store the result
- Store the value in the variable, evaluate the expression.
Customize your course in 30 seconds
Which class are you in.

Data Types, Variables and Constants
- Variables in Programming Language
- Concept of Data Types
- Declaration of Variables
- Type Modifiers
- Access Modifiers
- Constants in Programming Language
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

CS105: Introduction to Python
Variables and assignment statements.
Computers must be able to remember and store data. This can be accomplished by creating a variable to house a given value. The assignment operator = is used to associate a variable name with a given value. For example, type the command:
in the command line window. This command assigns the value 3.45 to the variable named a . Next, type the command:
in the command window and hit the enter key. You should see the value contained in the variable a echoed to the screen. This variable will remember the value 3.45 until it is assigned a different value. To see this, type these two commands:
You should see the new value contained in the variable a echoed to the screen. The new value has "overwritten" the old value. We must be careful since once an old value has been overwritten, it is no longer remembered. The new value is now what is being remembered.
Although we will not discuss arithmetic operations in detail until the next unit, you can at least be equipped with the syntax for basic operations: + (addition), - (subtraction), * (multiplication), / (division)
For example, entering these command sequentially into the command line window:
would result in 12.32 being echoed to the screen (just as you would expect from a calculator). The syntax for multiplication works similarly. For example:
would result in 35 being echoed to the screen because the variable b has been assigned the value a * 5 where, at the time of execution, the variable a contains a value of 7.
After you read, you should be able to execute simple assignment commands using integer and float values in the command window of the Repl.it IDE. Try typing some more of the examples from this web page to convince yourself that a variable has been assigned a specific value.
In programming, we associate names with values so that we can remember and use them later. Recall Example 1. The repeated computation in that algorithm relied on remembering the intermediate sum and the integer to be added to that sum to get the new sum. In expressing the algorithm, we used th e names current and sum .
In programming, a name that refers to a value in this fashion is called a variable . When we think of values as data stored somewhere i n the computer, we can have a mental image such as the one below for the value 10 stored in the computer and the variable x , which is the name we give to 10. What is most important is to see that there is a binding between x and 10.
The term variable comes from the fact that values that are bound to variables can change throughout computation. Bindings as shown above are created, and changed by assignment statements . An assignment statement associates the name to the left of the symbol = with the value denoted by the expression on the right of =. The binding in the picture is created using an assignment statemen t of the form x = 10 . We usually read such an assignment statement as "10 is assigned to x" or "x is set to 10".
If we want to change the value that x refers to, we can use another assignment statement to do that. Suppose we execute x = 25 in the state where x is bound to 10.Then our image becomes as follows:
Choosing variable names
Suppose that we u sed the variables x and y in place of the variables side and area in the examples above. Now, if we were to compute some other value for the square that depends on the length of the side , such as the perimeter or length of the diagonal, we would have to remember which of x and y , referred to the length of the side because x and y are not as descriptive as side and area . In choosing variable names, we have to keep in mind that programs are read and maintained by human beings, not only executed by machines.
Note about syntax
In Python, variable identifiers can contain uppercase and lowercase letters, digits (provided they don't start with a digit) and the special character _ (underscore). Although it is legal to use uppercase letters in variable identifiers, we typically do not use them by convention. Variable identifiers are also case-sensitive. For example, side and Side are two different variable identifiers.
There is a collection of words, called reserved words (also known as keywords), in Python that have built-in meanings and therefore cannot be used as variable names. For the list of Python's keywords See 2.3.1 of the Python Language Reference.
Syntax and Sema ntic Errors
Now that we know how to write arithmetic expressions and assignment statements in Python, we can pause and think about what Python does if we write something that the Python interpreter cannot interpret. Python informs us about such problems by giving an error message. Broadly speaking there are two categories for Python errors:
- Syntax errors: These occur when we write Python expressions or statements that are not well-formed according to Python's syntax. For example, if we attempt to write an assignment statement such as 13 = age , Python gives a syntax error. This is because Python syntax says that for an assignment statement to be well-formed it must contain a variable on the left hand side (LHS) of the assignment operator "=" and a well-formed expression on the right hand side (RHS), and 13 is not a variable.
- Semantic errors: These occur when the Python interpreter cannot evaluate expressions or execute statements because they cannot be associated with a "meaning" that the interpreter can use. For example, the expression age + 1 is well-formed but it has a meaning only when age is already bound to a value. If we attempt to evaluate this expression before age is bound to some value by a prior assignment then Python gives a semantic error.
Even though we have used numerical expressions in all of our examples so far, assignments are not confined to numerical types. They could involve expressions built from any defined type. Recall the table that summarizes the basic types in Python.
The following video shows execution of assignment statements involving strings. It also introduces some commonly used operators on strings. For more information see the online documentation. In the video below, you see the Python shell displaying "=> None" after the assignment statements. This is unique to the Python shell presented in the video. In most Python programming environments, nothing is displayed after an assignment statement. The difference in behavior stems from version differences between the programming environment used in the video and in the activities, and can be safely ignored.
Distinguishing Expressions and Assignments
So far in the module, we have been careful to keep the distinction between the terms expression and statement because there is a conceptual difference between them, which is sometimes overlooked. Expressions denote values; they are evaluated to yield a value. On the other hand, statements are commands (instructions) that change the state of the computer. You can think of state here as some representation of computer memory and the binding of variables and values in the memory. In a state where the variable side is bound to the integer 3, and the variable area is yet unbound, the value of the expression side + 2 is 5. The assignment statement side = side + 2 , changes the state so that value 5 is bound to side in the new state. Note that when you type an expression in the Python shell, Python evaluates the expression and you get a value in return. On the other hand, if you type an assignment statement nothing is returned. Assignment statements do not return a value. Try, for example, typing x = 100 + 50 . Python adds 100 to 50, gets the value 150, and binds x to 150. However, we only see the prompt >>> after Python does the assignment. We don't see the change in the state until we inspect the value of x , by invoking x .
What we have learned so far can be summarized as using the Python interpreter to manipulate values of some primitive data types such as integers, real numbers, and character strings by evaluating expressions that involve built-in operators on these types. Assignments statements let us name the values that appear in expressions. While what we have learned so far allows us to do some computations conveniently, they are limited in their generality and reusability. Next, we introduce functions as a means to make computations more general and reusable.

Python Enhancement Proposals
- Python »
- PEP Index »
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
Power Spreadsheets
Excel and VBA tutorials and training. Learn how to use Microsoft Excel and Visual Basic for Applications now.
Define Variables In VBA: How To Declare Variables And Assign Them Expressions
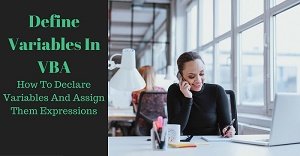
This data is generally stored in the computer's memory. For purposes of this Excel guide, we'll distinguish between the following 2 places to store data:
The former topic (objects) is covered in other Excel tutorials within Power Spreadsheets, including here and here . This blog post focuses on the latter topic (VBA variables).
Variables are often used when working with Visual Basic for Applications.
You have a substantial degree of flexibility when declaring VBA variables. This flexibility in declaring VBA variables can lead to poor coding practices. Poor coding practices can lead to potential problems down the road. These problems can lead to big headaches.
My purpose with this Excel tutorial is to help you avoid those headaches. Therefore, in this blog post, I explain the most important aspects surrounding VBA variable declaration . More specifically, this guide is divided in the following sections:
Table of Contents
Let's start by taking a detailed look at…
What Is A VBA Variable
For purposes of this blog post, is enough to remember that a variable is, broadly, a storage location paired with a name and used to represent a particular value .
As a consequence of this, variables are a great way of storing and manipulating data.
This definition can be further extended by referencing 3 characteristics of variables . A VBA variable:
- Is a storage location within the computer's memory.
- Is used by a program.
- Has a name.
In turn, these 3 main characteristics of variables provide a good idea of what you need to understand in order to be able to declare variables appropriately in VBA:
- How do you determine the way in which the data is stored, and how do you actually store the data. These items relate to the topics of data types (which I cover in a separate post ) and VBA variable declaration (which I explain below).
- How do you determine which program, or part of a program, can use a variable. This refers to the topics of variable scope and life.
- How do you name VBA variables.
I explain each of these 3 aspects below.
This Excel tutorial doesn't cover the topic of object variables . This is a different type of VBA variable, which serves as a substitute for an object. I may, however, cover that particular topic in a future blog post.
Since this how-to guide focuses on declaring variables in VBA, and we've already seen what a variable is, let's take a look at…
Why Should You Declare Variables Explicitly In VBA
Before I explain some of the most common reasons to support the idea that you should declare your VBA variables explicitly when working with Visual Basic for Applications, let's start by understanding what we're actually doing when declaring a variable explicitly . At a basic level: When you declare a variable, the computer reserves memory for later use.
Now, the common advice regarding VBA variable declaration says that declaring variables is an excellent habit .
There are a few reasons for this, but the strongest has to do with Excel VBA data types . The following are the main points that explain why, from these perspective, you should get used to declaring variables when working in Visual Basic for Applications:
- VBA data types determine the way in which data is stored in the computer's memory.
- You can determine the data type of a particular VBA variable when declaring it. However, you can also get away with not declaring it and allowing Visual Basic for Applications to handle the details.
- If you don't declare the data type for a VBA variable, Visual Basic for Applications uses Variant (the default data type).
- Despite being more flexible than other data types, and quite powerful/useful in certain circumstances, relying always on Variant has some downsides. Some of these potential problems include inefficient memory use and slower execution of VBA applications.
When you declare variables in VBA, you tell Visual Basic for Applications what is the data type of the variable . You're no longer relying on Variant all the time. Explicitly declaring variables and data types may result in (slightly) more efficient and faster macros.
Even though the above is probably the main reason why you should always declare variables in Visual Basic for Applications, it isn't the only one. Let's take a look at other reasons why you should declare variables when working with Visual Basic for Applications:
Additional Reason #1: Declaring Variables Allows You To Use The AutoComplete Feature
Let's take a look at the following piece of VBA code.

You can see the whole VBA code of the Variable_Test macro further below.
This Excel VBA Variables Tutorial is accompanied by Excel workbooks containing the data and macros I use. You can get immediate free access to these example workbooks by subscribing to the Power Spreadsheets Newsletter .
The next statements in the macro (after those that appear above) are:
MsgBox “the value of Variable One is ” & Variable_One & _ Chr(13) & “the value of Variable Two is ” & Variable_Two
Let's imagine that you're typing these statements and are currently at the following point:
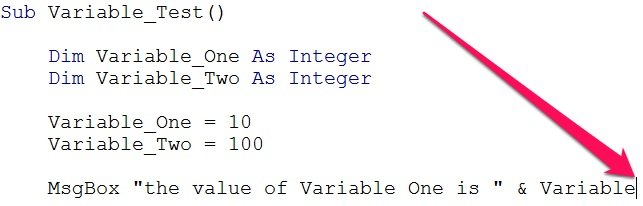
Theoretically, you must type the whole name of the variable: “Variable_One”. However, declaring the VBA variable previously allows you to take advantage of a keyboard shortcut : “Ctrl + Space”. Once you've typed the first few (can be as little as 2 or 3) letters of a declared variable name, you can press “Ctrl + Space” and the Visual Basic Editor does one of the following:
- As a general rule, the VBE completes the entry.
- If there is more than 1 option available, the Visual Basic Editor displays a list of possible matches to complete the entry.
In the case above, using the “Ctrl + Space” shortcut results in the Visual Basic Editor displaying a list of possible matches to complete the entry. This is shown in the following image.
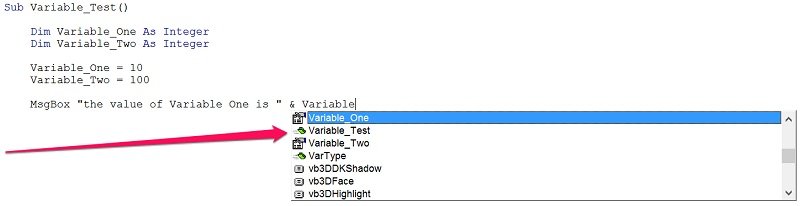
Notice how the list above, in addition to Variable_One and Variable_Two, also includes other reserved words and functions.
This is because the same keyboard shortcut (“Ctrl + Space”) also works for those 2 other elements. However, those are topics for future blog posts.
Now, let's assume that you pressed the “Ctrl + Space” keyboard shortcut at the following point:
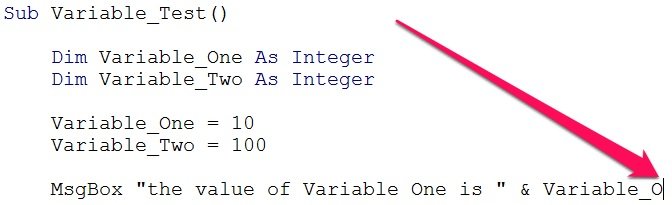
In this case, the Visual Basic Editor completes the entry.
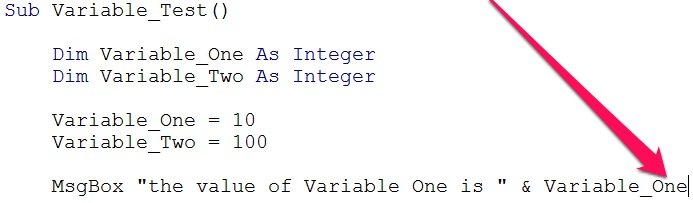
Additional Reason #2: Declaring Variables Makes VBA Carry Out Additional Error Checking
Some errors in Visual Basic for Applications may be very difficult to spot, particularly in large or complex VBA applications.
By declaring your VBA variables and defining their data type, you get some additional help from Visual Basic for Applications. When you explicitly declare the data type of your variables, VBA carries out some additional checking when compiling the code. In some of these cases, VBA can identify certain errors while you're working on the code.
The most common type of error that VBA can catch this way is data-typing errors. These errors are caused by incorrectly assigning certain information to a particular variable. More precisely, this usually happens when the assignment results in a mismatch between the following:
- The data type of the variable; and
- The type of the data assigned to the variable.
The following are some examples of how this can occur:
- You declare an Integer or Byte variable, but try to assign a string to it. The Integer and Byte data types are designed to store certain integers, not strings.
- You declare a Boolean variable, but try to assign a large integer to it. Variables of the Boolean data type can only be set to the 2 Boolean values (TRUE and FALSE).
Appropriately declaring your VBA variables (usually) allows you to notice these kind of mistakes as soon as possible after the variable has received an unexpected data type.
Additional Reason #3: Declaring Variables Improves Code Readability, Makes Debugging Easier And Reduces The Risk Of Certain Mistakes
As I explain below, whenever you declare VBA variables explicitly, you generally place all the variable declarations at the beginning of a module or procedure. This improves the readability of your VBA code.
Additionally, declaring variables explicitly makes your VBA code easier to debug and edit.
Finally, getting used to always declaring variables explicitly by following the different suggestions that appear in this Excel tutorial reduces the risk of certain mistakes. Among others, explicitly declaring variables reduces the risk of both:
- Variable naming-conflict errors; and
- Spelling or typographical mistakes.
Naming-conflict errors are quite dangerous. If you don't declare VBA variables, there is a higher risk of accidentally over-writing or wiping out a pre-existing variable when trying to create a new one.
Spelling mistakes can also create big problems. I explain one of the main ways in which taking measures to declare variables always helps you reduce spelling mistakes below.
However, in addition to that particular way, variable declaration also helps reduce spelling mistakes by capitalizing all the lower-case letters (in a variable name) that were capitalized at the point of the declaration statement.
To see how this works, let's go back to the VBA code for the Variable_Test macro. Let's assume that you type the name of Variable_One without capitalizing (as shown below):
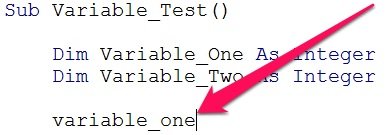
Once you complete the statement, the Visual Basic Editor automatically capitalizes the appropriate letters. This looks as follows:
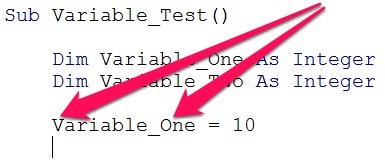
You can, for example, make it a habit to (i) use some capital letters in your VBA variable names but (ii) type the body of your VBA code in only lowercase letters. If the Visual Basic Editor doesn't capitalize a variable name after you've typed it, you may have typed the name wrongly. The VBE (generally) does this automatic capitalization for all keywords (not only VBA variables).
All of the advantages that I list under Additional Reason #3 are, in the end, related to the fact that variable declaration helps you (and VBA itself) have a better idea of what variables exist at any particular time.
So I suggest you save yourself some potential problems by getting used to declaring your VBA variables explicitly.
The Disadvantage Of Declaring VBA Variables Explicitly
Despite the advantages described above, the idea that you should always declare VBA variables explicitly is not absolutely accepted without reservations .
Most VBA users agree that declaring variables explicitly is (usually) a good idea.
However, some VBA users argue that both approaches have advantages and disadvantages. From this perspective, the main disadvantage of explicit variable declaration is the fact that it requires some upfront time and effort. In most cases, these disadvantages are (usually) outweighed by the advantages of explicit variable declaration.
In practice, however, it seems that for several VBA programmers the fact that declaring variables require slightly more time and effort isn't outweighed by the advantages described above. In fact, some developers do not declare variables explicitly.
I provide a general explanation of how to create a VBA variable without declaring it (known as declaring a variable implicitly) below.
However, let's assume for the moment that you're convinced about the benefits of declaring VBA variables. You want to do it always: no more undeclared variables.
In order to ensure that you follow through, you may want to know…
How To Remember To Declare Variables
Visual Basic for Applications has a particular statement that forces you to declare any variables you use . It's called the Option Explicit statement.
Whenever the Option Explicit statement is found within a module, you're prevented from executing VBA code containing undeclared variables .
The Option Explicit statement just needs to be used once per module. In other words:
- You only include 1 Option Explicit statement per module.
- If you're working on a particular VBA project that contains more than 1 module, you must have 1 Option Explicit statement in each module.
Including the Option Explicit statement in a module is quite simple:
Just type “Option Explicit” at the beginning of the relevant module, and before declaring any procedures . The following image shows how this looks like:
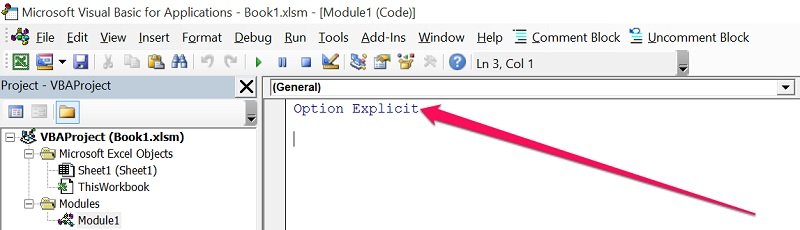
Once you've included the Option Explicit statement in a module, Visual Basic for Applications doesn't execute a procedure inside that module unless absolutely all the variables within that procedure are declared. If you try to call such procedure, Excel displays the following compile-time error message:
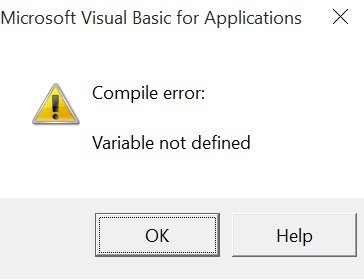
In addition to the reasons I provide above to support the idea that you should declare your VBA variables, there is an additional advantage when using the Option Explicit statement and having to declare absolutely all of your variables :
The Visual Basic Editor automatically proofreads the spelling of your VBA variable names .
Let's see exactly what I mean by taking a look at a practical example:
Let's go back to the Variable_Test macro that I introduced above. The following screenshot shows the full Sub procedure:

Notice how, at the beginning of the Sub procedure, the variables Variable_One and Variable_Two are declared. I explain how to declare VBA variables below.
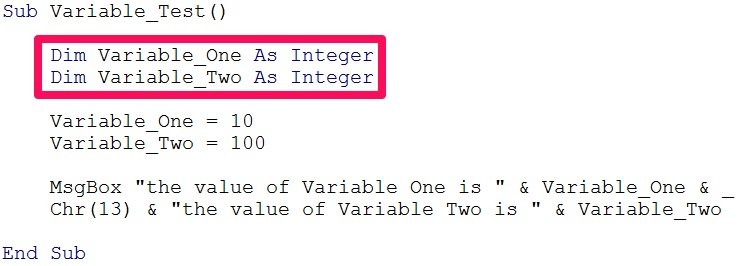
Notice, furthermore, how each of these variables appears 2 times after being declared:
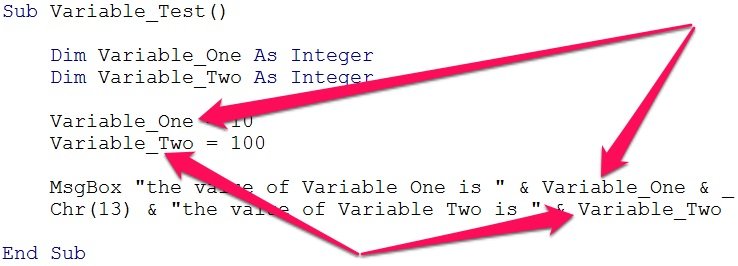
Let's assume that while writing the VBA code, you made a typo when writing the name of the VBA variable Variable_One (you typed “Variable_O m e”). In such a case, the code of the Variable_Test macro looks as follows:

Such small typos can be difficult to spot in certain situations. This is the case, for example, if they're present within the VBA code of a large Sub procedure or Function procedure where you're working with several different variables. This may be a big problem…
If you fail to notice these kind of typos within in the names of variables, Excel interprets the different spellings as different variables (for example, Variable_One and Variable_Ome). In other words, Excel creates a new variable using the misspelled name. This leads to having several variables even though you think you only have one and, in certain cases, may end in the macro returning the wrong result .
However, when you misspell the name of a VBA variable while having the Option Explicit statement enabled, Excel warns you about it . The following image shows the error message that is displayed when I try to run the Variable_Test macro with the typo shown above. Notice how the Visual Basic Editor:
- Clearly informs you that there is an undefined variable.
- Highlights the element of the VBA code where the mistake is.

Using the Option explicit statement is (generally) suggested .
Therefore, let's take this a step further by seeing how to ensure that the Option Explicit statement is always enabled:
How To Remember To Declare Variables Always
The Option Explicit statement is not enabled by default . This means that, if you want to use it always, you'd have to enable it for every single module you create.
However, the Visual Basic Editor is highly customizable. One of the customizations you can make is have the VBE always require that you declare your variables . The Visual Basic Editor does this by automatically inserting the Option Explicit statement at the beginning of any VBA module.
Enabling this option is generally recommended . If you want to have the Visual Basic Editor insert the Option Explicit statement in all your future modules, follow these 2 easy steps:
Step #1: Open The Options Dialog
You can get to the Options dialog of the Visual Basic Editor by clicking on the Tools menu and selecting “Options…”.

Step #2: Enable “Require Variable Declaration” And Click On The OK Button
The Visual Basic Editor should display the Editor tab of the Options dialog by default. Otherwise, simply click on the relevant tab at the top of the dialog.
Within the Editor tab, you simply need to enable “Require Variable Declaration” by ticking the box next to it. Then, click the OK button at the lower right corner of the dialog to complete the operation.

Note that enabling Require Variable Declaration option only applies to modules created in the future . In other words, Require Variable Declaration doesn't insert the Option Explicit statement in previously existing modules that were created while the option wasn't enabled.
You can learn more about customizing the Visual Basic Editor through the Options dialog, including a detailed description of the Require Variable Declaration setting, by clicking here .
You already know how to determine the way in which data is stored by using VBA data types. Let's take a look at how you actually store the data or, in other words…
How To Declare A Variable In VBA
The most common way of declaring a variable explicitly is by using the Dim statement . In practice, you're likely to use Dim for most of your variable declarations.
You've already seen this type of statement within the Variable_Test macro shown above. In that particular case, the Dim statement is used to declare the Variable_One and Variable_Two variables as follows:

Both variables are being declared as being of the Integer data type.
3 other keywords (in addition to Dim) may be used to declare a VBA variable explicitly :
As explained below, you generally use these 3 latter statements to declare variables with special characteristics regarding their scope or lifetime.
Regardless of the keyword that you're using to declare a variable, the basic structure of the declaration statement is the same :
Keyword Variable_Name As Data_Type
In this structure:
- “Keyword” is any of the above keywords: Dim, Static, Public or Private. In the following sections, I explain when is appropriate to use each of these.
- “Variable_Name” is the name you want to assign to the variable. I explain how to name VBA variables below.
- “Data_Type” makes reference to the data type of the variable. This element is optional, but recommended.
The question of which is the exact keyword that you should use to declare a particular VBA variable depends, mostly, on the scope and life you want that particular variable to have.
The following table shows the relationship between the 4 different statements you can use to declare a VBA variable and the 3 different scopes a variable can have :
I introduce the Dim, Static, Private and Public statements in the following sections. However, as you read their descriptions, you may want to constantly refer to the section below which covers VBA variable scope.
How To Declare A VBA Variable Using The Dim Statement
The Dim statement is the most common way to declare a VBA variable whose scope is procedure-level or module-level . I cover the topic of variable scope below.
Dim stands for dimension. In older versions of BASIC, this (Dim) statement was used to declare an array's dimensions .
Nowadays, in VBA, you use the Dim keyword to declare any variable, regardless of whether it is an array or not.
The image above shows the most basic way in which you can use the Dim statement to declare VBA variables. However, you can also use a single Dim statement to declare several variables . In such cases, you use a comma to separate the different variables.
The following screenshot shows how you can declare Variable_One and Variable_Two (used in the sample Variable_Test macro) by using a single Dim statement:

Even though this structure allows you to write fewer lines of VBA code, it (usually) makes the code less readable .
Regardless of whether you declare VBA variables in separate lines or in a single line, note that you can't declare several variables to be of a determined data type by simply separating the variables with a comma before declaring one data type . As explained in Excel 2013 Power Programming with VBA:
Unlike some languages, VBA doesn't let you declare a group of variables to be a particular data type by separating the variables with commas.
In other words, the following statement is not the equivalent as that which appears above:

In this latter case, only Variable_Two is being declared as an Integer.
In the case of Variable_One, this piece of code doesn't declare the VBA data type. Therefore, Visual Basic for Applications uses the default type: Variant.
The location of the Dim statement within a VBA module depends on the desired variable scope . I explain the topic of variable scope, and where to place your Dim statements depending on your plans, below.
How To Declare A Variable Using The Static Statement
You can use the Static statement to declare procedure-level VBA variables . It's an alternative to the Dim statement.
I explain the topic of variable scope below. However, for the moment, is enough to understand that procedure-level VBA variables can only be used within the procedure in which they are declared .
The main difference between VBA variables declared using the Dim statement and variables declared using the Static statement is the moment in which the variable is reset . Let's take a look at what this means precisely:
As a general rule (when declared with the Dim statement), all procedure-level variables are reset when the relevant procedure ends. Static variables aren't reset; they retain the values between calls of the procedure .
Static VBA variables are, however, reset when you close the Excel workbook in which they're stored. They're also reset (as I explain below) when a procedure is terminated by an End statement.
Despite the fact that Static variables retain their values after a procedure ends, this doesn't mean that their scope changes. Static variables continue to be procedure-level variables and, as such, they´re only available within the procedure in which they're declared.
These particular characteristics of Static variables make them particularly useful for purposes of storing data regarding a process that needs to be carried out more than 1 time. There are some special scenarios where this comes in very handy. The following are 2 of the main uses of Static variables:
- Storing data for a procedure that is executed again later and uses that information.
- Keeping track (counting) of a running total.
Let's take a quick look at these 2 examples:
Example #1: Toggling
You can use a Static variable within a procedure that toggles something between 2 states.
Consider, for example, a procedure that turns bold font formatting on and off. Therefore:
- The first time the procedure is called, bold formatting is turned on.
- In the second execution, bold formatting is turned off.
- For the third call, bold formatting is turned back on.
Example #2: Counters
A Static variable can (also) be useful to keep track of the number of times a particular procedure is executed. You can set up such a counter by, for example:
- Declaring a counter variable as a Static VBA variable.
- Increasing the value assigned to the Static variable by 1 every time the procedure is called.
How To Declare A VBA Variable Using The Private And Public Statements
You can use the Private statement to declare module-level variables . In these cases, it's an alternative to the Dim statement . I explain the topic of module-level variables in more detail below.
The fourth and final statement you can use to declare a VBA variable is Public. The Public statement is used to declare public variables . I explain public variables below.
At the beginning of this Excel tutorial, we saw that one of the defining characteristics of a VBA variable is that it is used by a program. Therefore, let's take a look at how do you determine which program, or part of a program, can use a determined variable. We start doing this by taking a look at…
How To Determine The Scope Of A VBA Variable
The scope of a VBA variable is what determines which modules and procedures can use that particular variable . In other words, it determines where that variable can be used.
Understanding how to determine the scope of a variable is very important because:
- A single module can have several procedures.
- A single Excel workbooks can have several modules.
If you're working with relatively simple VBA applications, you may only have one module with a few procedures. However, as your work with VBA starts to become more complex and sophisticated, this may change.
You may not want all of VBA variables to be accessible to every single module and procedure. At the same time, you want them to be available for the procedures that actually need them. Appropriately determining the scope of VBA variables allows you to do this.
Additionally, the scope of a VBA variable has important implications in connection with the other characteristics of the variable. An example of this is the life of a variable, a topic I explain below.
Therefore, let's take a look at the 3 main different scopes that you can apply :
Variable Scope #1: Procedure-Level (Or Local) VBA Variables
This is the most restricted variable scope.
As implied by its name, procedure-level variables (also known as local variables) can only be used within the procedure in which they are declared . This applies to both Sub procedures and Function procedures.
In other words, when the execution of a particular procedure ends, any procedure-level variable ceases to exist. Therefore, the memory used by the variable is freed up.
The fact that Excel frees up the memory used by procedure-level variables when the procedure ends makes this type of VBA variables particularly efficient. In most cases there's no reason to justify a variable having a broader scope than the procedure.
This is an important point: as a general rule, you should make your VBA variables as local as possible . Or, in other words: Limit a variable's scope to the level/scope you need them.
Excel doesn't store the values assigned to procedure-level VBA variables for use or access outside the relevant procedure. Therefore, if you call the same procedure again, any previous value of the procedure-level variables is lost.
Static variables (which I explain above) are, however, an exception to this rule . They generally retain their values after the procedure has ended.
Let's take a look at how you declare a procedure-level variable:
As I explain above, the usual way to declare a VBA variable is by using the Dim statement. This general rule applies to procedure-level variables.
You can also use the Static statement for declaring a procedure-level variable. In such a case, you simply use the Static keyword instead of the Dim keyword when declaring the VBA variable. You'd generally use the Static statement instead of Dim if you need the variable to retain its value once the procedure has ended .
The key to making a variable procedure-level is in the location of the particular declaration statement. This statement must be within a particular procedure .
The only strict requirement regarding the location of the variable declaration statement inside a procedure is that it is before the point you use that particular VBA variable . In practice, most VBA users declare all variables at the beginning of the applicable procedure.
More precisely, the most common location of a variable declaration statement within a procedure is:
- Immediately after the declaration statement of the relevant procedure.
- Before the main body of statements of the relevant procedure.
The VBA code of the Variable_Test macro is a good example of how you usually declare a VBA variable using the Dim statement. Notice how the Dim statement used to declare both variables (Variable_One and Variable_Two) is between the declaration statement of the Sub procedure and the main body of statements:

As you can imagine, placing all your VBA variable declaration statements at the beginning of a procedure makes the code more readable.
Since the scope of procedure-level VBA variables is limited to a particular procedure, you can use the same variable names in other procedures within the same Excel workbook or module. Bear in mind that, even though the name is the same, each variable is unique and its scope is limited to the relevant procedure.
I'm only explaining this for illustrative purposes, not to recommend that you repeat VBA variable names throughout your procedures. Repeating variable names in different procedures (inside the same project) can quickly become confusing. This is the case, particularly, when debugging those procedures. As you learn below, a common recommendation regarding variable name choice is to use unique variable names .
Let's see how this works in practice by going back to the module containing the Variable_Test Sub procedure and adding a second (almost identical procedure): Variable_Test_Copy.

Notice how, the only difference between the 2 Sub procedures is in the values assigned to the variables and the text that appears in the message box:

If I run the Variable_Test macro, Excel displays the following message box:

If I run the Variable_Test_Copy macro, Excel displays a very similar message box. Notice in particular how the values of Variable One and Variable Two change:
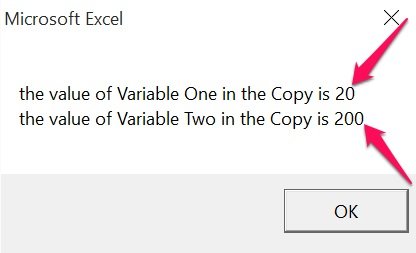
Let's move on to a wider variable scope:
Variable Scope #2: Module-Level (Also Known As Private) VBA Variables
The ideas behind the concept of module-level (also known as private) VBA variables are, to a certain extent, similar to what you've already seen regarding procedure-level variables (with a few variations).
As implied by their name, module-level variables are available for use in any procedure within the module in which the VBA variable is declared . Therefore, module-level variables survive the termination of a particular procedure. However, they're not available to procedures in other modules within the same VBA project.
As a consequence of the above, Excel stores the values assigned to module-level variables for use or access within the relevant module. Therefore, module-level variables retain their values between procedures, as long as those procedures are within the same module . Module-level variables allow you to pass data between procedures stored in the same module.
Despite the above, module-level variables don't retain their values between procedures (even if they're within the same module) if there is an End statement. As I explain below, VBA variables are purged and lose their values when VBA encounters such a statement.
The most common way to declare module-level VBA variables is by using the Dim statement . Alternatively, you can use the Private statement.
Module-level variables declared using the Dim statement are by default private. In other words, at a module-level, Dim and Private are equivalent. Therefore, you can use either.
However, some advanced VBA users suggest that using the Private statement can be a better alternative for declaring module-level variables . The reason for this is that, since you'll generally use the Dim statement to declare procedure-level variables, using a different keyword (Private) to declare module-level variables makes the code more readable by allowing you to distinguish between procedure-level and module-level variables easily.
Just as is the case for procedure-level variables, the key to determining the scope of a module-level variable is the location of the particular declaration statement . You declare a module-level VBA variable:
- Within the module in which you want to use the variable.
- Outside of any of the procedures stored within the module.
The way to achieve this is simply by including the relevant variable declaration statements within the Declarations section that appears at the beginning of a module.
In the case of the sample Excel workbooks that accompany this tutorial, the Declarations section is where the Option Explicit statement appears.
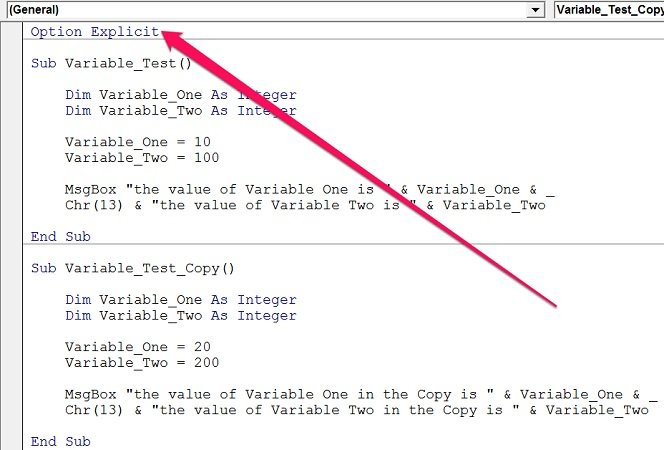
You can, when working within any VBA module, easily get to the Declarations section within the Visual Basic Environment by clicking on the drop-down list on the top right corner of the Code Window (known as the Procedure Box) and selecting “(Declarations)”.
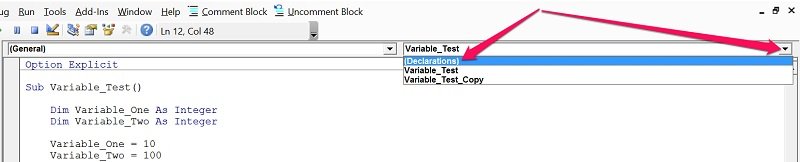
This same drop-down list continues to display “(Declarations)” as long as you're working within the Declarations section of the relevant module.

Let's see the practical consequences of working with module-level variables by modifying the VBA code above as follows:
- Making Variable_One and Variable_Two module-level variables by declaring them within the Declarations section.
- Deleting the statements that assigned values to Variable_One and Variable_Two in the Variable_Test_Copy macro. The assignment made within the Variable_Test Sub procedure is maintained.
The resulting VBA code is as follows:

The following message box is displayed by Excel when the Variable_Test macro is executed:
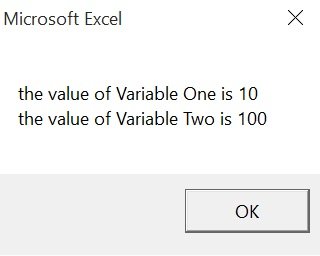
And the following is the message box displayed when the Variable_Test_Copy macro is executed. Notice how both variables (Variable_One and Variable_Two):
- Can be used in both of the procedures within the relevant module.
- Have retained the values that were assigned by the Variable_Test macro for use in the Variable_Test_Copy macro.

Now, let's take a look at the third type of variable scope:
Variable Scope #3: Public VBA Variables
The availability of public VBA variables is even broader than that of module-level variables:
- At the basic level, public variables they are available for any procedure within any VBA module stored in the relevant Excel workbook .
- At a more complex level, you can make public variables available to procedures and modules stored in different workbooks .
Variables that are declared with the Public statement are visible to all procedures in all modules unless Option Private Module applies. If Option Private Module is enabled, variables are only available within the project in which they are declared.
When deciding to work with public VBA variables, remember the general rule I describe above: make your VBA variables as local as possible .
Let's start by taking a look at the basic case:
How To Declare Public VBA Variables: The Basics
In order to declare a public VBA variable , the declaration needs to meet the following conditions:
- Be made in the Declarations section, just as you'd do when declaring a module-level variable.
- Use the Public statement in place of the Dim statement.
- The declaration must be made in a standard VBA module.
VBA variables whose declarations meet these conditions are available to any procedure (even those in different modules) within the same Excel workbook .
To see how this works, let's go back to the macros above: the Variable_Test and Variable_Test_Copy macros and see how the variables (Variable_One and Variable_Two) must be declared in order to be public.
For this example, I first store both macros in different modules. Notice how, in the screenshot below, there are 2 modules shown in the Project Explorer.
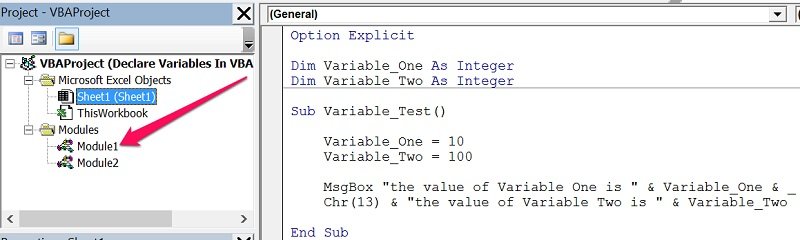
Module1 stores the Variable_Test macro while Module2 stores the Variable_Test_Copy macro. The variables are declared in Module1.
This Excel VBA Variables Tutorial is accompanied by Excel workbooks containing the data and macros I use (including the macros above). You can get immediate free access to these example workbooks by subscribing to the Power Spreadsheets Newsletter .
Notice how, in the screenshot above, both variables are declared using the Dim statement. Therefore, as it stands, they're module-level variables. If I try to execute the Variable_Test_Copy macro (which now has no variable declaration nor assignment) which is stored in Module2, Excel returns the following error message:
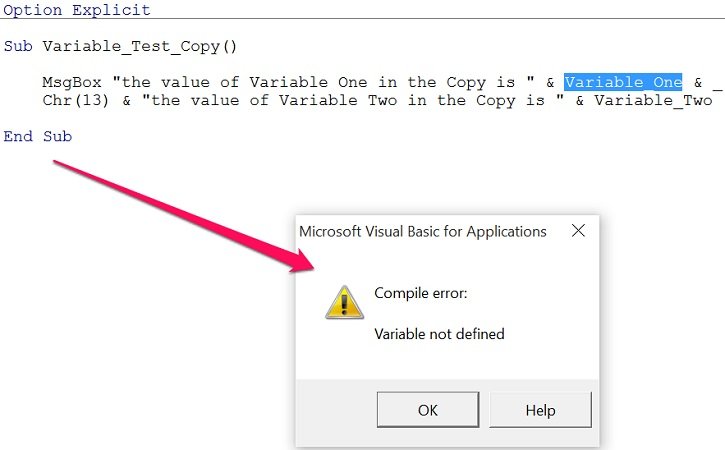
This is exactly the same error message displayed in the example above, when examining the Option Explicit statement and the reasons why you should always declare variables.
Let's go back to Module1, where both Variable_One and Variable_Two are declared, and change the keyword used to declare them. Instead of using Dim, we use Public. The rest of the macro remains unchanged.
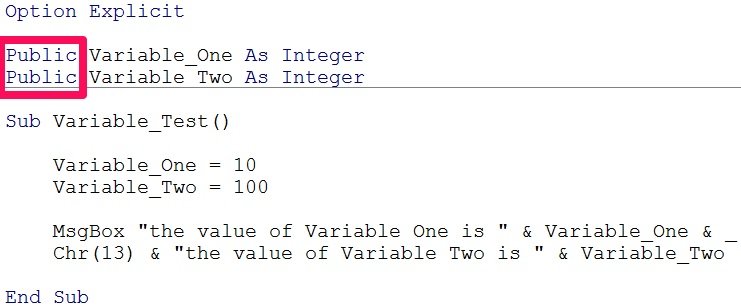
If I run again both macros (Variable_Test and Variable_Test_Copy) in the same order I did above, the message boxes displayed by Excel now show the same values. More precisely:

As shown by the screenshots above:
- Both variables are now available to all the procedures within the relevant workbook, even though they are stored in separate modules.
- The variables retain the values assigned by the first macro (Variable_Test) for use by the second macro (Variable_Test_Copy).
This basic method of declaring public VBA variables is, in practice, the only one that you really need to know. However, in case you're curious, let's take a look at…
How To Declare Public VBA Variables Available Across Different Workbooks
In practice, you'll likely only use public VBA variables that are available for all the procedures within the particular Excel workbook in which the variable is declared. This is the method I describe in the previous section.
However, it is also possible to make public VBA variables available to procedures within modules stored in different Excel workbooks. This is rarely needed (or done).
You make a variable that is available to modules stored in Excel workbooks different from that in which the variable is actually declared by following these 2 simple steps:
- Step #1: Declare the variable as Public, following the indications in the previous section.
- Step #2: Create a reference to the Excel workbook where the relevant variable is declared. You can set up a reference by using the References command in the Visual Basic Editor. This command appears in the Tools menu of the VBE.
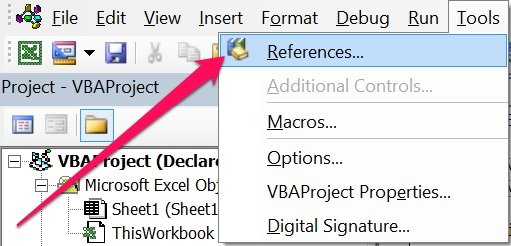
As a general rule, the scope of a variable determines its lifetime. Therefore, let's take a look at…
What Is The Life Of A VBA Variable And How Is It Determined
The term “life” refers to how long a variable is stored in the computer's memory .
A variable's life is closely related to its scope. In addition to determining where a variable may be used, a variable's scope may (in certain cases) determine the moment at which the variable is removed from the computer's memory.
In other words: The life of a VBA variable (usually) depends on its scope .
Fortunately, the topic of variable life is simpler than that of scope. More precisely, there are 2 basic rules :
- Rule #1: Procedure-level VBA variables declared with the Dim statement are removed from the memory when the relevant procedure finishes.
- Rule #2: Procedure-level Static variables, module-level variables and public variables retain their values between procedure calls.
You can purge all the variables that are currently stored in memory using any of the following 3 methods :

- Method #3: Include an End statement within your VBA code. From a broad point of view, the End statement has different syntactical forms. However, they one I'm referring to here is simply “End”. You can put this End statement anywhere in your code. The End statement can have several consequences, such as terminating code execution and closing files that were opened with the Open statement. For purposes of this Excel tutorial, the most important consequence of using the End statement is that it clears variables from the memory.
Having a good understanding of these 3 variable-purging methods is important, even if you don't plan to use them explicitly. The reason for this is that a variable purge may cause your module-level or public variables to lose their content (even without you noticing) .
Therefore, when using module-level or public variables, ensure they hold/represent the values you expect them to hold/represent .
Now that we've fully covered the topic of how to determine which application or part of an application can use a variable (by looking at the topics of VBA variable scope and life), let's understand…
How To Name VBA Variables
Visual Basic for Applications provides a lot of flexibility in connection with how you can name variables . As a general matter, the rules that apply to variable naming are substantially the same rules that apply to most other elements within Visual Basic for Applications.
The main rules that apply to VBA variable naming can be summarized as follows . I also cover the topic of naming (in the context of naming procedures) within Visual Basic for Applications here and here , among other places.
- The first character of the variable name must be a letter.
- Characters (other than the first) can be letters, numbers, and some punctuation characters. One of the most commonly used punctuation characters is the underscore (_). Some VBA programmers, use it to improve the readability of VBA variable names. For illustration purposes, take a look at the macro names I use in this blog post . Other VBA users omit the underscore and use mixed case variable names (as explained below).
- VBA variable names can't include spaces ( ), periods (.), mathematical operators (for example +, –, /, ^ or *), comparison operators (such as =, < or >) or certain punctuation characters (such as the type-declaration characters @, #, $, %, & and ! that I explain below).
- The names of VBA variables can't be the same as those of any of Excel's reserved keywords. These reserved keywords include Sub, Dim, With, End, Next and For. If you try to use any of this words, Excel returns a syntax error message. These messages aren't very descriptive. Therefore, if you get a strange compile error message, it may be a good idea to confirm that you're not using a reserved keyword to name any of your VBA variables . You can (theoretically) use names that match names in Excel's VBA object model (such as Workbook, Worksheet and Range). However, this increases the risk of confusion Therefore, it is generally suggested that you avoid using such variable names. Similarly, consider avoiding variable names that match names used by VBA, or that match the name of built-in functions, statements or object members . Even if this practice doesn't cause direct problems, it may prevent you from using the relevant function, statement or object member without fully qualifying the applicable reference. In summary: it's best to create your own variable names, and make them unique and unambiguous .
- Visual Basic for Applications doesn't distinguish between upper and lowercase letters. For example, “A” is the same as “a”. Regardless of this rule, some VBA programmers use mixed case variable names for readability purposes. For an example, take a look at the variable names used in macro example #5 , such as aRow and BlankRows. Some advanced VBA users suggest that it may be a good idea to start variable names with lowercase letters. This reduces the risk of confusing those names with built-in VBA keywords.
- The maximum number of characters a VBA variable name can have is 255. However, as mentioned in Excel 2013 Power Programming with VBA , very long variable names aren't recommended.
- The name of each VBA variable must be unique within its relevant scope. This means that the name of a procedure-level variable must be unique within the relevant procedure, the name of a module-level variable must be unique within its module, and so on. This requirement makes sense if you consider how important it is to ensure that Visual Basic for Applications uses the correct variable (instead of confusing it with another one). At a more general level, it makes sense to have (almost) always unique names . As I explain above, re-using variable names can be confusing when debugging. Some advanced VBA users consider that there are some reasonable exceptions to this rule, such as certain control variables.
In addition to these rules, and suggestions related to those rules, you can find a few additional practices or suggestions made by Excel experts below.
For example, one of the most common suggestions regarding VBA variable naming, is to make variable names descriptive .
In some case, you can use comments to describe variables that don't have descriptive names. I suggest, however, that you try to use descriptive variable names. They are, in my opinion, more helpful.
Finally, advanced VBA users suggest that you add a prefix to the names of VBA variables for purposes of identifying their data type . These users argue that adding these prefixes makes the code more readable and easier to modify since all variables can be easily identified as of a specific data type throughout the VBA code.
The following table shows the main tags that apply to VBA variables:
In Excel 2013 Power Programming with VBA , John Walkenbach introduces a similar naming convention. In that case, the convention calls for using a lowercase prefix indicating the data type. The following table shows these prefixes (some are the same as those in the table above):
Walkenbach doesn't use this convention because he thinks it makes the code less readable. However, other advanced VBA users include a couple of letters at the beginning of the variable name to represent the data type.
In the end, you'll develop your own variable-naming method that works for you. What's more important than following the ideas of other VBA users, is to develop and (consistently) use some variable naming conventions . Please feel free to use any of the ideas and suggestions that appear (or are linked to) above when creating your variable-naming system…
And make sure to let me know your experience and ideas by leaving a comment below .
The sections above cover all the main items within the declaration statement of a VBA variable. Let's take a quick look at what happens after you've declared the variable by understanding…
How To Assign A Value Or Expression To A VBA Variable
Before you assign a value to a declared VBA variable, Excel assigns the default value . Which value is assigned by default depends on the particular data type of the variable.
- The default value for numeric data types (such as Byte, Integer, Long, Currency, Single and Double) is 0.
- In the case of string type variables (such as String and Date), the default value is an empty string (“”), or the ASCII code 0 (or Chr(0)). This depends on whether the length of the string is variable or fixed.
- In a numeric context, the Empty value is represented by 0.
- In a string context, it's represented by the zero-length string (“”).
As a general rule, however, you should explicitly assign a value or expression to your VBA variables instead of relying in the default values.
The statement that you use to assign a value to a VBA variable is called, fittingly, an Assignment statement. Assigning an initial value or expression to a VBA variable is also known as initializing the variable.
Assignment statements take a value or expression (yielding a string, number or object) and assign it to a VBA variable or a constant .
You've probably noticed that you can't declare a VBA variable and assign a value or expression to it in the same line. Therefore, in Visual Basic for Applications, assignment statements are separate from variable declaration statements.
This Excel tutorial refers only to variables (not constants) and expressions are only covered in an introductory manner. Expressions are more useful and advanced than values. Among other strengths, expressions can be as complex and sophisticated as your purposes may require.
Additionally, if you have a good understanding of expressions, you shouldn't have any problem with values. Therefore, let's take a closer look at what an expression is.
Within Visual Basic for Applications, expressions can be seen as having 3 main characteristics :
- Element #1: Keywords.
- Element #2: Operators.
- Element #3: Variables.
- Element #4: Constants.
- Item #1: A string.
- Item #2: A number.
- Item #3: An object.
- Purpose #1: Perform calculations .
- Purpose #2: Manipulate characters.
- Purpose #3: Test data.
Expressions aren't particularly complicated. The main difference between formulas and expressions is what you do with them:
- When using worksheet formulas , Excel displays the result they return in a cell.
- When using expressions, you can use Visual Basic for Applications to assign the result to a VBA variable.
As a general rule, you use the equal sign (=) to assign a value or expression to a VBA variable . In other words, the equal sign (=) is the assignment operator.
When used in this context, an equal sign (=) doesn't represent an equality. In other words, the equal sign (=) simply the assignment operator and not a mathematical symbol denoting an equality.
The basic syntax of an assignment statement using the equal sign is as follows:
- On the right hand side of the equal sign: The value or expression you're storing.
- On the left hand side of the equal sign: The VBA variable where you're storing the data.
You can change the value or expression assigned to a variable during the execution of an application .
To finish this section about Assignment statements, let's take a quick look at the assignments made in the sample macros Variable_Test and Variable_Test_Copy. The following image shows how this (quite basic and simple) assignments were made.
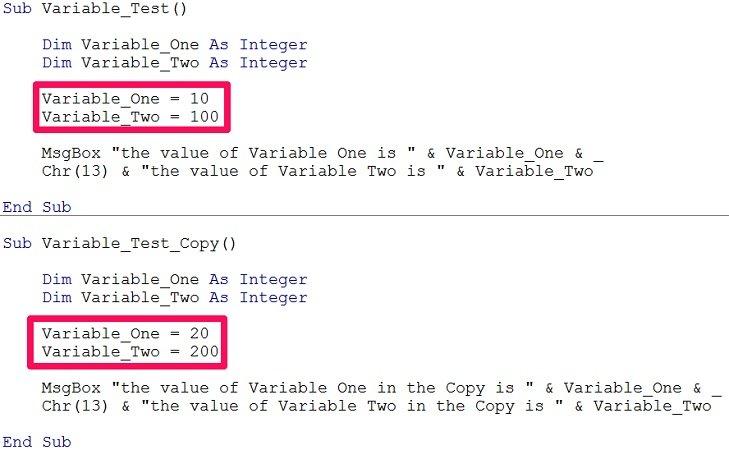
Notice the use of the equal sign (=) in both cases. In both the Variable_Test and the Variable_Test_Copy macros, the value that appears on the right side of the equal sign is assigned to the variable that appears on the left side of the sign.
By now we've covered pretty much all the basics in connection with declaring variables in Visual Basic for Applications. You may, however, be wondering…
What if I don't want to follow the suggestions above and don't want to declare variables explicitly?
The following section explains how you can do this…
How To Declare VBA Variables Implicitly (a.k.a. How To Create Variables Without Declaring Them)
The first few sections of this Excel tutorial explain why declaring variables explicitly is a good idea.
However, realistically speaking, this may not be always possible or convenient. In practice, several VBA Developers don't declare variables explicitly. In fact, these Developers tend to (simply) create variables as (and when) required.
Doing this is relatively simple and is known as declaring a variable implicitly. In practical terms, this means using a VBA variable in an assignment statement without declaring explicitly (as explained throughout most of this blog post) first. This works because, when VBA encounters a name that it doesn't recognize (for example as a variable, reserved word, property or method ), it creates a new VBA variable using that name.
Let's take a look at a practical example by going back to the Variable_Test and Variable_Test_Copy macros…
The image below shows the VBA code of both the Variable_Test and Variable_Test_Copy macros without Variable_One and Variable_Two being ever declared explicitly. Notice how there is no Dim statement, as it is the case in the image above. Both VBA Sub procedures begin by simply assigning a value to the relevant variables.
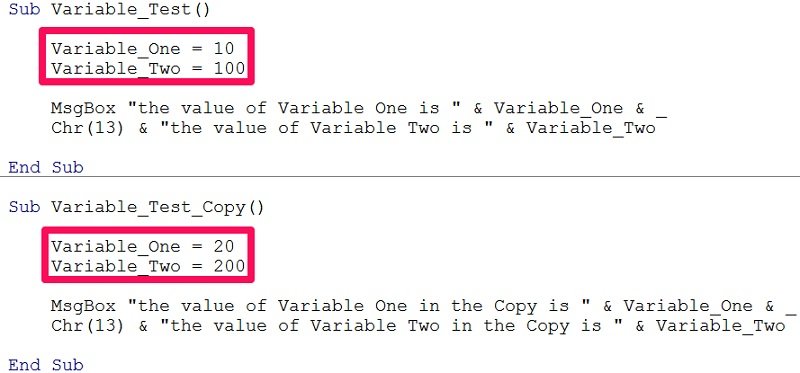
When this happens, Visual Basic for Applications proceeds as follows :
- Step #1: It carries out a check to confirm that there isn't an existing variable with the same name.
- Step #2: If there are no other variables with the same name, it creates the variable. Since the data type of the variable isn't declared, VBA uses the default Variant type and assigns one of its sub-types.
You can, actually, set the data type of an implicitly declared variable by using type-declaration (also known as type-definition) characters . These are characters that you can add at the end of the variable name to assign the data type you want.
As explained by John Walkenbach in Excel 2013 Power Programming with VBA , type-declaration characters are a “holdover” from BASIC. He agrees that it's generally better to declare variables using the methods I describe above .
The following table displays the main type-declaration characters you can use:
For example, in the case of the Variable_Test and Variable_Test_Copy macros that appear above, you can assign the Integer data type to the variables Variable_One and Variable_Two by adding the % type-declaration character at the end of their respective names. The VBA code looks, then, as follows:
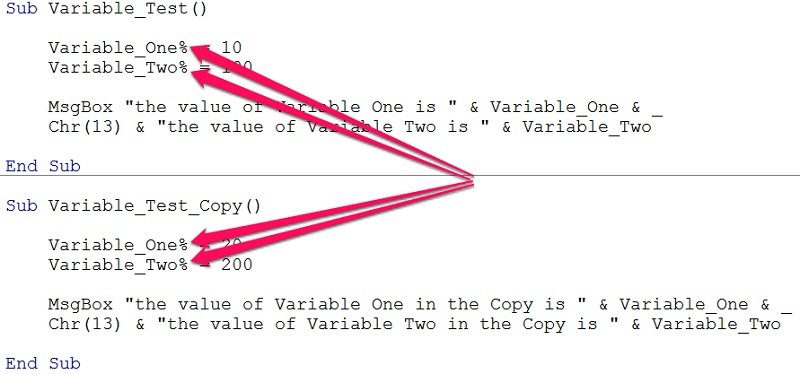
As shown above, you only need to include the type-declaration character once . Afterwards, you can use the variable name as usual (without type-declaration character). The following image shows how this is the case in the Variable_Test and Variable_Test_Copy macros:
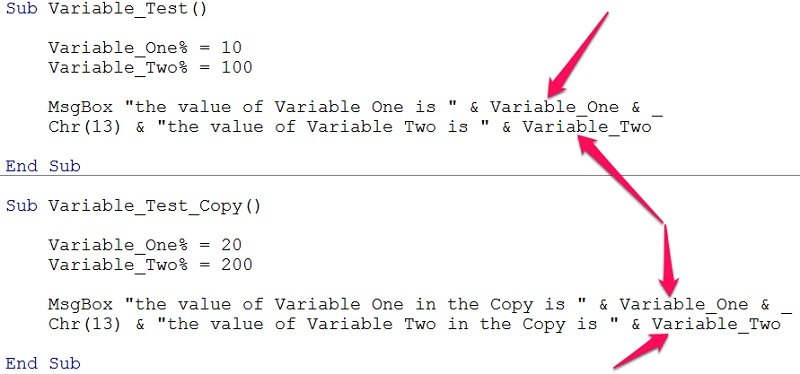
There are 2 main advantages to declaring variables implicitly as described above :
- Advantage #1: More flexibility: By not declaring variables you are more flexible since, whenever you need a variable, you simply use it in a statement to declare it implicitly.
- Advantage #2: You write less VBA code.
However, before you decide to start declaring your variables implicitly as explained in this section, remember the advantages of always declaring your variables and using the Option Explicit statement, and the potential problems whose origin is the lack of variable declaration.
Variables are a very flexible element that you're likely to encounter constantly while working with Visual Basic for Applications. As a consequence of that flexibility and ubiquity, is important to have a good understanding of how to work with them .
Otherwise, you may eventually run into a lot of problems while debugging your VBA code. Or even worse: your macros may simply not work as intended (without you noticing) and return wrong results.
This Excel tutorial has covered the most important topics related to VBA variable declaration . Additionally, we've seen several different suggestions and best practices regarding work with VBA variables. Some of the most important topics covered by this VBA tutorial are the following:
- What is a VBA variable.
- Why is it convenient to declare VBA variables explicitly.
- How can you remember to declare variables explicitly.
- How to declare VBA variables taking into consideration, among others, the desired scope and life of that variable.
- How to name variables.
- How to assign values or expressions to VBA variables.
Finally, in case I didn't convince you to declare your VBA variables explicitly, the last section of this Excel tutorial covered the topic of implicit variable declaration. This section provides some guidance of how to work with variables without declaring them first.
As you may have noticed, there are a lot of different opinions on the topic of best practices regarding VBA variables. As explained above, I encourage you to develop your own variable naming method .
- See what works for you.
- Formalize it.
- Use it consistently.
Books Referenced In This Excel Tutorial
- Walkenbach, John (2013). Excel 2013 Power Programming with VBA . Hoboken, NJ: John Wiley & Sons Inc.
I publish a lot of Tutorials and Training Resources about Microsoft Excel and VBA . Here are some of my most popular Excel Training Resources:
- Free Excel VBA Email Course
- Excel Macro Tutorial for Beginners
- Excel Power Query (Get and Transform) Tutorial for Beginners
- Excel Keyboard Shortcut Cheat Sheet
- Excel Resources

IMAGES
VIDEO
COMMENTS
int x; // define an integer variable named x int y, z; // define two integer variables, named y and z. Variable assignment. After a variable has been defined, you can give it a value (in a separate statement) using the = operator. This process is called assignment, and the = operator is called the assignment operator.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ): Python. >>> n = 300. This is read or interpreted as " n is assigned the value 300 .". Once this is done, n can be used in a statement or expression, and its value will be substituted: Python.
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
Variable Assignment. Think of a variable as a name attached to a particular object. In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ).
Assigning variables. Here's how we create a variable named score in JavaScript: var score = 0; That line of code is called a statement. All programs are made up of statements, and each statement is an instruction to the computer about something we need it to do. Let's add the lives variable: var score = 0; var lives = 3;
Assignment sets a value to a variable. To assign variable a value, use the equals sign (=) myFirstVariable = 1 mySecondVariable = 2 myFirstVariable = "Hello You" Assigning a value is known as binding in Python. In the example above, we have assigned the value of 2 to mySecondVariable. Note how I assigned an integer value of 1 and then a string ...
This is called an assignment statement.We've pointed the variable count to the value 4.. We don't have declarations or initializations. Some programming languages have an idea of declaring variables.. Declaring a variable says a variable exists with this name, but it doesn't have a value yet.After you've declared the variable, you then have to initialize it with a value.
In general, assigning a variable b to a variable a will cause the variables to reference the same object in the system's memory, and assigning c to a or b will simply have a third variable reference this same object. Then any change (a.k.a mutation) of the object will be reflected in all of the variables that reference it (a, b, and c).
A variable is created the moment you first assign a value to it. Example. x = 5 y = "John" print(x) print(y) Try it Yourself » Variables do not need to be declared with any particular type, and can even change type after they have been set. Example. x = 4 # x is of type int x = "Sally" # x is now of type str
Python Variable is containers that store values. Python is not "statically typed". We do not need to declare variables before using them or declare their type. A variable is created the moment we first assign a value to it. A Python variable is a name given to a memory location. It is the basic unit of storage in a program.
when a variable name appears on the left-hand side of an assignment statement it is used as a reference to a storage allocation (lvalue); when it is used on the right-hand side of an assignment statement it stands for the variable's value (rvalue). (However, certain C operators require that their operands be lvalues - e.g. operators like ++.)
In an assignment statement, everything on the right of the equals sign is calculated, and the result is assigned to the variable on the left. Whenever you see an assignment statement, you can read or think of the equals sign as meaning any of the following: "is assigned". "is given the value of". "is set to". "becomes".
defines the variable named x and initialises it with the expression 5. 5 is a literal value. If x has been previously declared, x = 5; is a simple expression statement - its sole purpose is evaluating an assignment expression. The 5, again, is a literal with value 5. x = 5 is therefore an expression, which assigns x to have the value 5.
An Assignment statement is a statement that is used to set a value to the variable name in a program. Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted.
The assignment operator = is used to associate a variable name with a given value. For example, type the command: a=3.45. in the command line window. This command assigns the value 3.45 to the variable named a. Next, type the command: a. in the command window and hit the enter key. You should see the value contained in the variable a echoed to ...
Rule 1. Name must be comprised of digits, upper case letters, lower case letters, and the underscore character "_". Rule 2. Must begin with a letter or underscore. A good name for a variable is short but suggestive of its role: Circle_Area.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME: ... if anonymous, function definition) counts as a scope for this purpose. There is one special case: an assignment expression occurring in a list, set or dict ... If either assignment statements or assignment expressions can be used ...
The statement that you use to assign a value to a VBA variable is called, fittingly, an Assignment statement. Assigning an initial value or expression to a VBA variable is also known as initializing the variable. Assignment statements take a value or expression (yielding a string, number or object) and assign it to a VBA variable or a constant.
The one liner doesn't work because, in Python, assignment (fruit = isBig(y)) is a statement, not an expression.In C, C++, Perl, and countless other languages it is an expression, and you can put it in an if or a while or whatever you like, but not in Python, because the creators of Python thought that this was too easily misused (or abused) to write "clever" code (like you're trying to).
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...
Overview. These are some of the most common ways that you can create variables in a DATA step: use an assignment statement. read data with the INPUT statement in a DATA step. specify a new variable in a FORMAT or INFORMAT statement. specify a new variable in a LENGTH statement. specify a new variable in an ATTRIB statement.