- C - Introduction
- C - Comments
- C - Data Types
- C - Type Casting
- C - Operators
- C - Strings
- C - Booleans
- C - If Else
- C - While Loop
- C - For Loop
- C - goto Statement
- C - Continue Statement
- C - Break Statement
- C - Functions
- C - Scope of Variables
- C - Pointers
- C - Typedef
- C - Format Specifiers
- C Standard Library
- C - Data Structures
- C - Examples
- C - Interview Questions
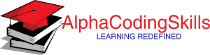
- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- PHP & MySQL
- C++ Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference

C - Bitwise XOR and assignment operator
The Bitwise XOR and assignment operator (^=) assigns the first operand a value equal to the result of Bitwise XOR operation of two operands.
(x ^= y) is equivalent to (x = x ^ y)
The Bitwise XOR operator (^) is a binary operator which takes two bit patterns of equal length and performs the logical exclusive OR operation on each pair of corresponding bits. It returns 1 if only one of the bits is 1, else returns 0.
The example below describes how bitwise XOR operator works:
The code of using Bitwise XOR and assignment operator (^=) is given below:
The output of the above code will be:
Example: Swap two numbers without using temporary variable
The bitwise XOR and assignment operator can be used to swap the value of two variables. Consider the example below.
The above code will give the following output:
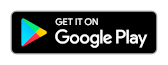
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Java.lang Package
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
C Precedence And Associativity Of Operators
C Programming Operators
- Convert Binary Number to Decimal and vice-versa
- Convert Binary Number to Octal and vice-versa
- Make a Simple Calculator Using switch...case
Bitwise Operators in C Programming
In the arithmetic-logic unit (which is within the CPU), mathematical operations like: addition, subtraction, multiplication and division are done in bit-level. To perform bit-level operations in C programming, bitwise operators are used.
Bitwise AND Operator &
The output of bitwise AND is 1 if the corresponding bits of two operands is 1 . If either bit of an operand is 0 , the result of corresponding bit is evaluated to 0 .
In C Programming, the bitwise AND operator is denoted by & .
Let us suppose the bitwise AND operation of two integers 12 and 25 .
Example 1: Bitwise AND
Bitwise or operator |.
The output of bitwise OR is 1 if at least one corresponding bit of two operands is 1 . In C Programming, bitwise OR operator is denoted by | .
Example 2: Bitwise OR
Bitwise xor (exclusive or) operator ^.
The result of bitwise XOR operator is 1 if the corresponding bits of two operands are opposite. It is denoted by ^ .
Example 3: Bitwise XOR
Bitwise complement operator ~.
Bitwise complement operator is a unary operator (works on only one operand). It changes 1 to 0 and 0 to 1 . It is denoted by ~ .
Twist in Bitwise Complement Operator in C Programming
The bitwise complement of 35 ( ~35 ) is -36 instead of 220 , but why?
For any integer n , bitwise complement of n will be -(n + 1) . To understand this, you should have the knowledge of 2's complement.
2's Complement
Two's complement is an operation on binary numbers. The 2's complement of a number is equal to the complement of that number plus 1. For example:
The bitwise complement of 35 is 220 (in decimal). The 2's complement of 220 is -36 . Hence, the output is -36 instead of 220 .
Bitwise Complement of Any Number N is -(N+1). Here's how:
Example 4: Bitwise complement
Shift operators in c programming.
There are two shift operators in C programming:
- Right shift operator
- Left shift operator.
Right Shift Operator
Right shift operator shifts all bits towards right by certain number of specified bits. It is denoted by >> .
Left Shift Operator
Left shift operator shifts all bits towards left by a certain number of specified bits. The bit positions that have been vacated by the left shift operator are filled with 0 . The symbol of the left shift operator is << .
Example #5: Shift Operators
Table of contents.
- Bitwise AND
- Bitwise XOR
- Bitwise complement
- Shift right
Sorry about that.
Related Tutorials
C Ternary Operator

C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
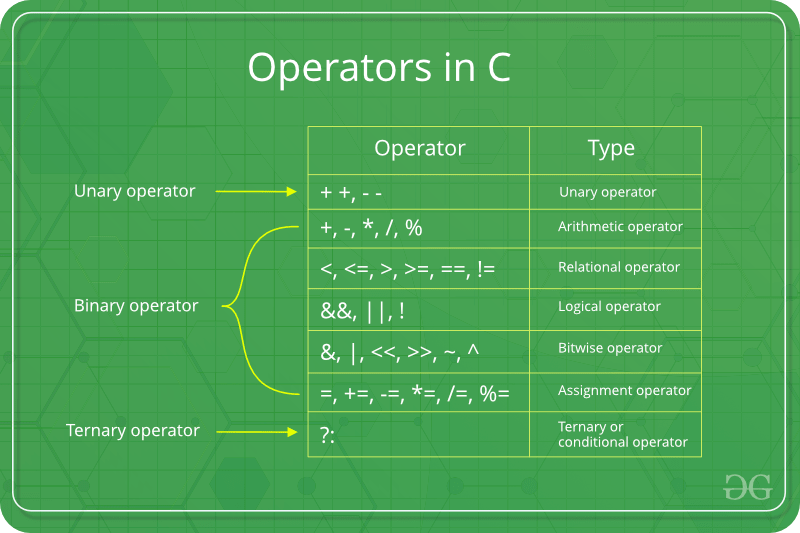
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
- C-Operators
- cpp-operator
- 10 Best Free Social Media Management and Marketing Apps for Android - 2024
- 10 Best Customer Database Software of 2024
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Logical XOR Operator in C
an Overview of XOR ( ^ ) Operator in C
Use ^ to apply xor in c programming language, apply xor in c programming language.
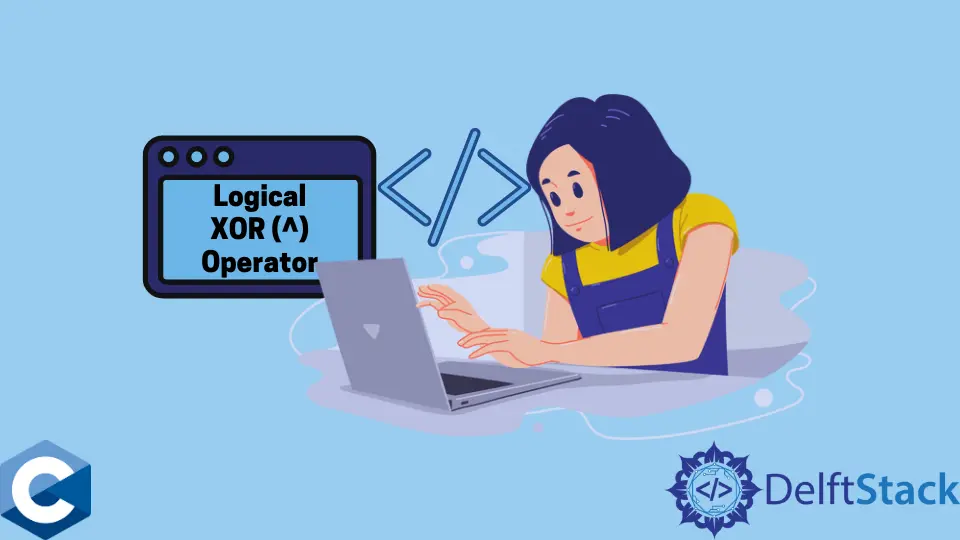
In this article, we will understand about logical XOR ( ^ ) operator in the C programming language.
Exclusive OR , also known as XOR, is a logical operator that returns a true result when either of the operands is true (one is true, and the other is false), but not when both are true nor when both are false. When both operands are true, using the basic or in logical condition creation might lead to confusion.
Because under such circumstances, it is challenging to comprehend what it is that precisely meets the criteria. This issue has been resolved by adding the qualifier exclusive to the word or , which also clarifies its meaning.
The ^ operator, also known as bitwise XOR, takes two integers as its operands and performs XOR on every bit of both numbers.
If both bits are different, the XOR operation will provide a value of 1 . If the bits are identical, it will return the value 0.
Let us take an example to apply the bitwise XOR in C. Firstly, import the libraries and create a main() function.
Inside the main function, establish two variables named firstValue and secondValue with the int datatype and set integer values for each. The firstValue is equal to 0101, and the secondValue is equal to 1001 in binary format.
Now that we have these two variables, we need to apply XOR to them, so let us create a new variable called applyXOR and use the symbol ^ on both of these variables.
In the above expression, the XOR operation will be performed on both binary values shown as follows.
Now we get the number 1100 in binary format, which, converted to decimal, is the same as the value 12 . Then we print the value that was obtained.
Code Example:
Let’s take another example to demonstrate the working of the XOR ( ^ ) operator.
Given a collection of numbers in which every item occurs an even number of times apart from one number, locate the odd occurring number. Simply applying the XOR( ^ ) operator to each integer to solve this issue is an effective way to tackle it.
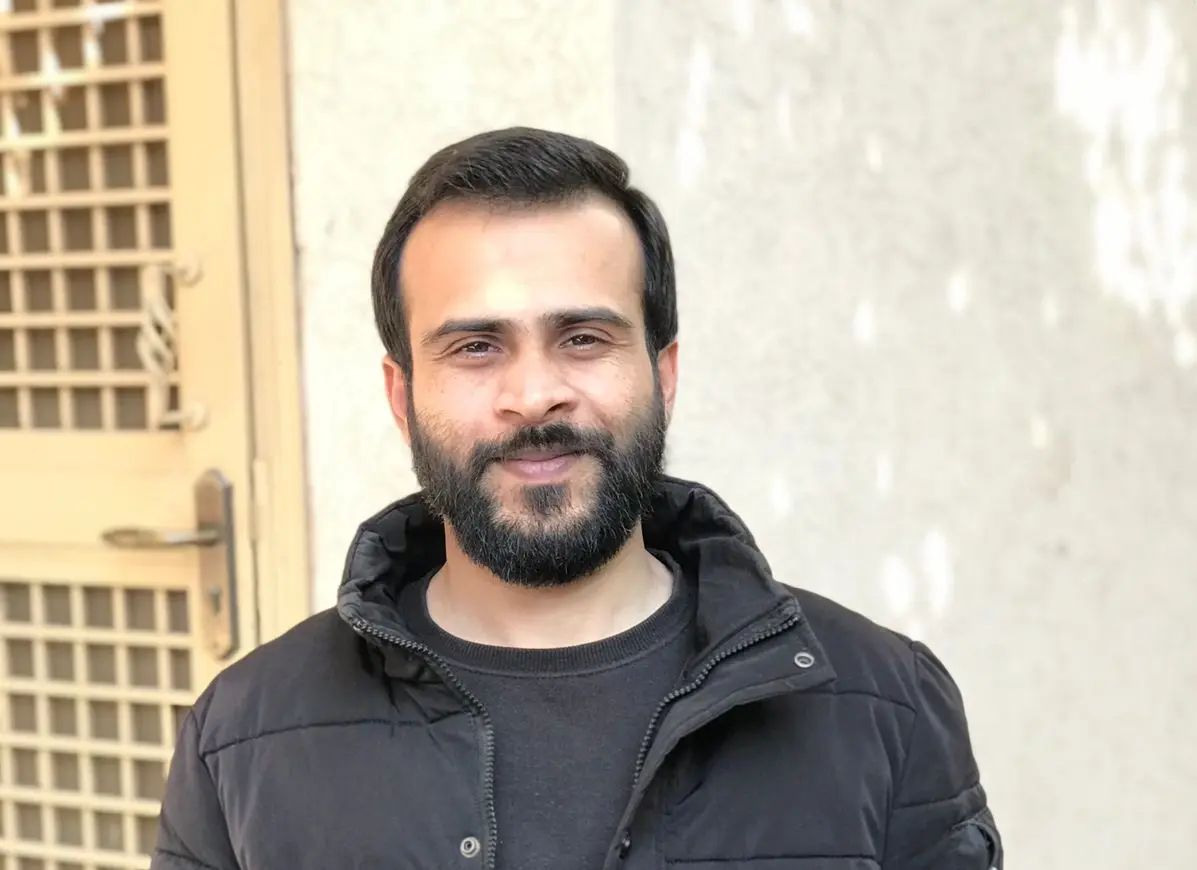
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
Related Article - C Operator
- Types of Shift Operators in C
- How to Generate Random Number in C
- i++ vs ++i in C
cppreference.com
C operator precedence.
The following table lists the precedence and associativity of C operators. Operators are listed top to bottom, in descending precedence.
- ↑ The operand of prefix ++ and -- can't be a type cast. This rule grammatically forbids some expressions that would be semantically invalid anyway. Some compilers ignore this rule and detect the invalidity semantically.
- ↑ The operand of sizeof can't be a type cast: the expression sizeof ( int ) * p is unambiguously interpreted as ( sizeof ( int ) ) * p , but not sizeof ( ( int ) * p ) .
- ↑ The expression in the middle of the conditional operator (between ? and : ) is parsed as if parenthesized: its precedence relative to ?: is ignored.
- ↑ Assignment operators' left operands must be unary (level-2 non-cast) expressions. This rule grammatically forbids some expressions that would be semantically invalid anyway. Many compilers ignore this rule and detect the invalidity semantically. For example, e = a < d ? a ++ : a = d is an expression that cannot be parsed because of this rule. However, many compilers ignore this rule and parse it as e = ( ( ( a < d ) ? ( a ++ ) : a ) = d ) , and then give an error because it is semantically invalid.
When parsing an expression, an operator which is listed on some row will be bound tighter (as if by parentheses) to its arguments than any operator that is listed on a row further below it. For example, the expression * p ++ is parsed as * ( p ++ ) , and not as ( * p ) ++ .
Operators that are in the same cell (there may be several rows of operators listed in a cell) are evaluated with the same precedence, in the given direction. For example, the expression a = b = c is parsed as a = ( b = c ) , and not as ( a = b ) = c because of right-to-left associativity.
[ edit ] Notes
Precedence and associativity are independent from order of evaluation .
The standard itself doesn't specify precedence levels. They are derived from the grammar.
In C++, the conditional operator has the same precedence as assignment operators, and prefix ++ and -- and assignment operators don't have the restrictions about their operands.
Associativity specification is redundant for unary operators and is only shown for completeness: unary prefix operators always associate right-to-left ( sizeof ++* p is sizeof ( ++ ( * p ) ) ) and unary postfix operators always associate left-to-right ( a [ 1 ] [ 2 ] ++ is ( ( a [ 1 ] ) [ 2 ] ) ++ ). Note that the associativity is meaningful for member access operators, even though they are grouped with unary postfix operators: a. b ++ is parsed ( a. b ) ++ and not a. ( b ++ ) .
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- A.2.1 Expressions
- C11 standard (ISO/IEC 9899:2011):
- C99 standard (ISO/IEC 9899:1999):
- C89/C90 standard (ISO/IEC 9899:1990):
- A.1.2.1 Expressions
[ edit ] See also
Order of evaluation of operator arguments at run time.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 31 July 2023, at 09:28.
- This page has been accessed 2,665,989 times.
- Privacy policy
- About cppreference.com
- Disclaimers


COMMENTS
The Bitwise XOR and assignment operator (^=) assigns the first operand a value equal to the result of Bitwise XOR operation of two operands. (x ^= y) is equivalent to (x = x ^ y) The Bitwise XOR operator (^) is a binary operator which takes two bit patterns of equal length and performs the logical exclusive OR operation on each pair of ...
The output of bitwise AND is 1 if the corresponding bits of two operands is 1. If either bit of an operand is 0, the result of corresponding bit is evaluated to 0. In C Programming, the bitwise AND operator is denoted by &. Let us suppose the bitwise AND operation of two integers 12 and 25. 12 = 00001100 (In Binary) 25 = 00011001 (In Binary)
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Bitwise Operators in C. In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C. The & (bitwise AND) in C takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
1. 1. 1. The bitwise AND operator is a single ampersand: &. It is just a representation of AND which does its work on the bits of the operands rather than the truth value of the operands. Bitwise binary AND performs logical conjunction (shown in the table above) of the bits in each position of a number in its binary form.
There are two important things about these operators: 1) they guarantee short-circuit evaluation, 2) they introduce a sequence point, 3) they evaluate their operands only once. XOR evaluation, as you understand, cannot be short-circuited since the result always depends on both operands. So 1 is out of question.
Bitwise XOR (exclusive OR) is a binary operation that takes two equal-length binary representations and performs the logical XOR operation on each pair of corresponding bits. The result in each position is 1 if only one of the two bits is 1 but will be 0 if both are 0 or both are 1. The truth table for the XOR (exclusive OR) operation is as ...
Different types of assignment operators are shown below: 1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: 2. “+=”: This operator is combination of ‘+’ and ‘=’ operators.
an Overview of XOR ( ^) Operator in C. Exclusive OR, also known as XOR, is a logical operator that returns a true result when either of the operands is true (one is true, and the other is false), but not when both are true nor when both are false. When both operands are true, using the basic or in logical condition creation might lead to confusion.
They are derived from the grammar. In C++, the conditional operator has the same precedence as assignment operators, and prefix ++ and -- and assignment operators don't have the restrictions about their operands. Associativity specification is redundant for unary operators and is only shown for completeness: unary prefix operators always ...